Warning! This section will be deprecated on February 1st 2025. Please move all your Indicators to the cTrader Store catalogue.
Description
This requires the Pivot Channels indicator.
This allows you to plot the three support / resistance lines, as well as the pivot, and the high-low of a previous period on a lower time frame.
For example, we've shown here the pivot lines and high-low from the daily chart on the H1 chart, and also the pivot lines from the H4 chart on the H1 chart.
Find us at: fxtradersystems.com
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Cloud("Yesterday's High", "Yesterday's Low")]
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class MTFPivotChannels : Indicator
{
[Parameter("TimeFrame", DefaultValue = "Daily")]
public TimeFrame timeFrame { get; set; }
[Output("Pivot", LineColor = "White")]
public IndicatorDataSeries Pivot { get; set; }
[Output("Yesterday's Low", LineColor = "Cyan")]
public IndicatorDataSeries YestLow { get; set; }
[Output("Support 1", LineColor = "LawnGreen")]
public IndicatorDataSeries Support1 { get; set; }
[Output("Support 2", LineColor = "Lime")]
public IndicatorDataSeries Support2 { get; set; }
[Output("Support 3", LineColor = "Green")]
public IndicatorDataSeries Support3 { get; set; }
[Output("Yesterday's High", LineColor = "Cyan")]
public IndicatorDataSeries YestHigh { get; set; }
[Output("Resistance 1", LineColor = "Tomato")]
public IndicatorDataSeries Resistance1 { get; set; }
[Output("Resistance 2", LineColor = "Red")]
public IndicatorDataSeries Resistance2 { get; set; }
[Output("Resistance 3", LineColor = "DarkRed")]
public IndicatorDataSeries Resistance3 { get; set; }
private Bars customBars;
private PivotChannels pivotChannels;
protected override void Initialize()
{
customBars = MarketData.GetBars(timeFrame);
pivotChannels = Indicators.GetIndicator<PivotChannels>(customBars.HighPrices, customBars.LowPrices, customBars.ClosePrices);
}
public override void Calculate(int index)
{
if (index == 0)
return;
int customIndex = customBars.OpenTimes.GetIndexByTime(Bars.OpenTimes[index]);
Pivot[index] = pivotChannels.Pivot[customIndex];
YestHigh[index] = pivotChannels.YestHigh[customIndex];
YestLow[index] = pivotChannels.YestLow[customIndex];
Resistance1[index] = pivotChannels.Resistance1[customIndex];
Support1[index] = pivotChannels.Support1[customIndex];
Resistance2[index] = pivotChannels.Resistance2[customIndex];
Support2[index] = pivotChannels.Support2[customIndex];
Resistance3[index] = pivotChannels.Resistance3[customIndex];
Support3[index] = pivotChannels.Support3[customIndex];
}
}
}
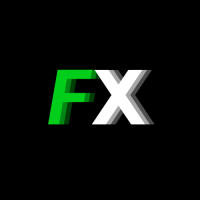
fxtradersystems
Joined on 10.09.2020
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: MTF Pivot Channels.algo
- Rating: 0
- Installs: 1563
- Modified: 13/10/2021 09:54
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.