Description
V4 is in blue - same as V3 (green) but:
Flips trend direction if the bar is either an inner or outer bar to previous.
Then checks the trend direction using the relationship of the current and previous high prices.
Once again, let us know if there are any bugs.
Find us at: fxtradersystems.com/development/
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class DrawPriceMoveV4 : Indicator
{
[Output("ZigZag", Thickness = 2, PlotType = PlotType.Line, LineColor = "Lime")]
public IndicatorDataSeries Value { get; set; }
enum Direction
{
up,
down
}
private Direction direction = Direction.down;
private double extremumPrice = 0.0;
private int extremumIndex = 0;
private void moveExtremum(int index, double price)
{
Value[extremumIndex] = Double.NaN;
setExtremum(index, price);
}
private void setExtremum(int index, double price)
{
extremumIndex = index;
extremumPrice = price;
Value[extremumIndex] = extremumPrice;
}
private bool isInside(int index)
{
return (Bars.LowPrices[index] >= Bars.LowPrices[index - 1]) && (Bars.HighPrices[index] <= Bars.HighPrices[index - 1]);
}
private bool isOutside(int index)
{
return (Bars.LowPrices[index] <= Bars.LowPrices[index - 1]) && (Bars.HighPrices[index] >= Bars.HighPrices[index - 1]);
}
public override void Calculate(int index)
{
double low = Bars.LowPrices[index];
double high = Bars.HighPrices[index];
if (extremumPrice == 0.0)
extremumPrice = high;
if (Bars.OpenTimes.Count < 2)
return;
if (direction == Direction.down)
{
if (isInside(index) || isOutside(index))
{
setExtremum(index, high);
direction = Direction.up;
}
else if (Bars.HighPrices[index] <= Bars.HighPrices[index - 1])
moveExtremum(index, low);
else
{
setExtremum(index, high);
direction = Direction.up;
}
}
else
{
if (isInside(index) || isOutside(index))
{
setExtremum(index, low);
direction = Direction.down;
}
else if (Bars.HighPrices[index] >= Bars.HighPrices[index - 1])
moveExtremum(index, high);
else
{
setExtremum(index, low);
direction = Direction.down;
}
}
}
}
}
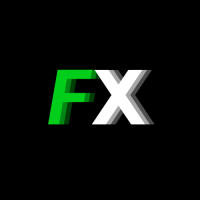
fxtradersystems
Joined on 10.09.2020
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: DrawPriceMoveV4.algo
- Rating: 0
- Installs: 1416
- Modified: 13/10/2021 09:54
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
Hi, could u add amount tick delta per swing (uptick-downtick), pips per swing, cumulative tick delta into it?
Highlight current swing up, when previous swing up has more pips, less delta per swing than current swing up, vice versa for down swing.