Description
Developed this through a forum request.
Currently limited functionality as it was a quick turn around, will only work on the daily timeframe.
Takes as input a CSV file with Dates and numbers (two colums with one row of titles), and the delimiter type (here we are displaying EVZ Daily data).
Depending on how people find it, we would be happy to expand its capabilities. Future pipeline could include:
- Functionality for more timeframes
- Full on EVZ pipeline for those NNFX traders out there.
Just let us know if you find any bugs, and we ccan update the source code :)
You can support the team at: fxtradersystems.com/products/donate/
Or submit development requests at: fxtradersystems.com/support/
Equally, you can email: development@fxtradersystems.com
using System;
using System.IO;
using System.Collections.Generic;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
using System.Globalization;
namespace cAlgo
{
[Indicator(IsOverlay = false, TimeZone = TimeZones.UTC, AccessRights = AccessRights.FullAccess)]
public class DailyCustomIndi : Indicator
{
[Parameter("Path to CSV:")]
public string Path { get; set; }
[Parameter("Delimiter", DefaultValue = ",")]
public string Delimiter { get; set; }
[Output("Main")]
public IndicatorDataSeries Result { get; set; }
// Set up a dictionary to hold the dates and values
Dictionary<DateTime, double> keyValuePairs = new Dictionary<DateTime, double>();
int count;
protected override void Initialize()
{
keyValuePairs = new Dictionary<DateTime, double>();
count = 0;
Print("keyValuePairs initialised");
using (var reader = new StreamReader(Path))
{
Print("Streamer initialised");
while (!reader.EndOfStream)
{
// Use the reader to read in the lines
var line = reader.ReadLine();
var values = line.Split(Convert.ToChar(Delimiter));
if (values.Length < 2)
{
}
else
{
// Split out dates and values, and then convert them to datetime and double
string stringDate = values[0];
string stringIndi = values[1];
if (count != 0)
{
DateTime date = Convert.ToDateTime(stringDate, CultureInfo.InvariantCulture);
DateTime shortDate = CompactDate(date);
double doubleIndi;
// Try converting the double part to a double:
bool convertDoubleSuccessful = Double.TryParse(stringIndi, out doubleIndi);
// Set incorrect parses to NaN
if (!convertDoubleSuccessful)
{
Print("Warning:" + shortDate.ToString() + " is NaN");
doubleIndi = double.NaN;
}
// Add to dictionary
keyValuePairs.Add(shortDate, doubleIndi);
}
else
{
count = 1;
}
}
}
Print("Streamer finished");
}
}
public override void Calculate(int index)
{
// Get the datetime of the current bar (at Index)
DateTime indexDateTime = Bars.OpenTimes[index];
DateTime shortIndexDate = CompactDate(indexDateTime);
double indexResult;
// Find the appropriate dateTime in the dictionary and then add to result:
bool findSuccessful = keyValuePairs.TryGetValue(shortIndexDate, out indexResult);
if (findSuccessful)
{
Result[index] = indexResult;
}
else
{
Result[index] = double.NaN;
Print("Warning " + shortIndexDate.ToString() + " key could not be found.");
}
}
public DateTime CompactDate(DateTime longDate)
{
DateTime shortDate = new DateTime(longDate.Year, longDate.Month, longDate.Day);
return shortDate;
}
}
}
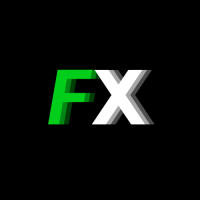
fxtradersystems
Joined on 10.09.2020
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Daily Custom Indi.algo
- Rating: 5
- Installs: 1025
- Modified: 13/10/2021 09:54
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
great work