Description
@lookformoneyy just asked for this one on the forum (https://ctrader.com/forum/indicator-support/24945) so we did a quick bit of work.
Any development projects people want doing, you can email development@fxtradersystems.com, or get in contact at: fxtradersystems.com/support/
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class DrawPriceMove : Indicator
{
[Output("ZigZag", Thickness = 1, PlotType = PlotType.Line)]
public IndicatorDataSeries Value { get; set; }
/*
[Output("UpTrend", Thickness = 2, PlotType = PlotType.DiscontinuousLine, LineColor = "Lime")]
public IndicatorDataSeries UpTrend { get; set; }
[Output("DownTrend", Thickness = 1, PlotType = PlotType.DiscontinuousLine, LineColor = "Tomato")]
public IndicatorDataSeries DownTrend { get; set; }
*/
enum Direction
{
up,
down
}
private Direction direction = Direction.down;
private double extremumPrice = 0.0;
private int extremumIndex = 0;
private void moveExtremum(int index, double price)
{
Value[extremumIndex] = Double.NaN;
setExtremum(index, price);
}
private void setExtremum(int index, double price)
{
extremumIndex = index;
extremumPrice = price;
Value[extremumIndex] = extremumPrice;
}
public override void Calculate(int index)
{
double low = Bars.LowPrices[index];
double high = Bars.HighPrices[index];
if (extremumPrice == 0.0)
extremumPrice = high;
if (Bars.OpenTimes.Count < 2)
return;
if (direction == Direction.down)
{
if (Bars.HighPrices[index] <= Bars.HighPrices[index - 1])
moveExtremum(index, low);
else
{
setExtremum(index, high);
direction = Direction.up;
}
}
else
{
if (Bars.HighPrices[index] >= Bars.HighPrices[index - 1])
moveExtremum(index, high);
else
{
setExtremum(index, low);
direction = Direction.down;
}
}
}
}
}
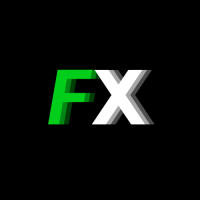
fxtradersystems
Joined on 10.09.2020
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: DrawPriceMove.algo
- Rating: 0
- Installs: 1115
- Modified: 13/10/2021 09:54
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
@lookformoneyy got in contact, and asked for a change. Have updated to more closely resemble the photos they sent.
Will continue to update till @lookformoneyy is happy.
(Have not implemented up / down color changes yet)