Warning! This section will be deprecated on February 1st 2025. Please move all your Indicators to the cTrader Store catalogue.
Description
This indicator is known as SuperTrend or ATR Stop. The Calculation is based on the ATR to better identify a trend change.
You can find me by joyining this Telegram Group http://t.me/cTraderCoders
Grupo de Telegram Para Brasileiros, Portugueses e todos aqueles que falam portugues:http://t.me/ComunidadeCtrader
Grupo CTrader en Español para Latinos: http://t.me/ComunidadCtrader
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Cloud("Close Up", "Bull Line", Opacity = 0.2, FirstColor = "FF7CFC00")]
[Cloud("Bear Line", "Close Down", Opacity = 0.2, FirstColor = "Red")]
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class SuperTrendExperto : Indicator
{
[Parameter("ATR Period", DefaultValue = 13)]
public int Period { get; set; }
[Parameter("ATR Multiplier", DefaultValue = 2)]
public double atrMulti { get; set; }
[Parameter("ATR Source", DefaultValue = MovingAverageType.Exponential)]
public MovingAverageType AtrSource { get; set; }
[Output("Bear Line", LineColor = "A8FF0000", PlotType = PlotType.DiscontinuousLine, LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries HighLine { get; set; }
[Output("Bull Line", LineColor = "877CFC00", PlotType = PlotType.DiscontinuousLine, LineStyle = LineStyle.Solid, Thickness = 1)]
public IndicatorDataSeries LowLine { get; set; }
[Output("Close Up", PlotType = PlotType.DiscontinuousLine, LineColor = "Transparent")]
public IndicatorDataSeries UpClose { get; set; }
[Output("Close Down", PlotType = PlotType.DiscontinuousLine, LineColor = "Transparent")]
public IndicatorDataSeries DownClose { get; set; }
public AverageTrueRange atr;
public double AtrDistance;
public IndicatorDataSeries Direction;
protected override void Initialize()
{
atr = Indicators.AverageTrueRange(Period, AtrSource);
Direction = CreateDataSeries();
}
public override void Calculate(int index)
{
AtrDistance = atr.Result[index] * atrMulti;
if (index == 0 && Bars.ClosePrices[index] >= Bars.OpenPrices[index])
{
Direction[index] = 1;
LowLine[index] = Bars.LowPrices[index] - AtrDistance;
HighLine[index] = double.NaN;
UpClose[index] = Bars.ClosePrices[index];
DownClose[index] = double.NaN;
}
else if (index == 0 && Bars.ClosePrices[index] < Bars.OpenPrices[index])
{
Direction[index] = -1;
HighLine[index] = Bars.HighPrices[index] + AtrDistance;
LowLine[index] = double.NaN;
DownClose[index] = Bars.ClosePrices[index];
UpClose[index] = double.NaN;
}
//*****************************************************************************************
if (Direction[index - 1] == 1)
{
if (Bars.ClosePrices[index] < LowLine[index - 1])
{
LowLine[index] = Bars.LowPrices[index] - AtrDistance;
Direction[index] = -1;
UpClose[index] = Bars.ClosePrices[index];
DownClose[index] = double.NaN;
}
else
{
Direction[index] = 1;
}
}
//********************************* Bear Trend **************************************
else if (Direction[index - 1] == -1)
{
if (Bars.ClosePrices[index] > HighLine[index - 1])
{
HighLine[index] = Bars.HighPrices[index] + AtrDistance;
Direction[index] = 1;
DownClose[index] = Bars.ClosePrices[index];
UpClose[index] = double.NaN;
}
else
{
Direction[index] = -1;
}
}
if (Direction[index] == 1)
{
Direction[index] = 1;
if (LowLine[index - 1] > Bars.LowPrices[index] - AtrDistance)
{
LowLine[index] = LowLine[index - 1];
HighLine[index] = double.NaN;
UpClose[index] = Bars.ClosePrices[index];
DownClose[index] = double.NaN;
}
else
{
LowLine[index] = Bars.LowPrices[index] - AtrDistance;
HighLine[index] = double.NaN;
UpClose[index] = Bars.ClosePrices[index];
DownClose[index] = double.NaN;
}
}
//********************************* Bear Trend **************************************
else if (Direction[index] == -1)
{
Direction[index] = -1;
if (HighLine[index - 1] < Bars.HighPrices[index] + AtrDistance)
{
HighLine[index] = HighLine[index - 1];
LowLine[index] = double.NaN;
DownClose[index] = Bars.ClosePrices[index];
UpClose[index] = double.NaN;
}
else
{
HighLine[index] = Bars.HighPrices[index] + AtrDistance;
LowLine[index] = double.NaN;
DownClose[index] = Bars.ClosePrices[index];
UpClose[index] = double.NaN;
}
}
}
}
}
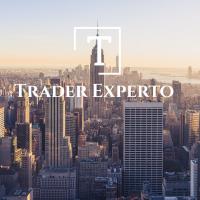
TraderExperto
Joined on 07.06.2019
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: SuperTrend Experto.algo
- Rating: 5
- Installs: 5866
- Modified: 13/10/2021 09:54
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
Hi, your supertrend indicator has a parameter for ATR source (moving average type). How is ATR source used in calculation of supertrend?
I am asking because default supertrend available on ctrader platform doesn't have ATR source (moving average type). Thanks