Description
This bot use Relative Strength Index (RSI) to Trade.
using System;
using System.Linq;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class DragonRSI : Robot
{
[Parameter(" Volume Percent", DefaultValue = 1, MinValue = 0)]
public double VolumePercent { get; set; }
[Parameter("Stop Loss", DefaultValue = 100)]
public int StopLoss { get; set; }
[Parameter("Take Profit", DefaultValue = 100)]
public int TakeProfit { get; set; }
[Parameter()]
public DataSeries Source { get; set; }
[Parameter(DefaultValue = 20)]
public int Period { get; set; }
[Parameter(DefaultValue = 70)]
public int MaxLevel { get; set; }
[Parameter(DefaultValue = 30)]
public int MinLevel { get; set; }
private RelativeStrengthIndex _rsi;
protected override void OnStart()
{
_rsi = Indicators.RelativeStrengthIndex(Source, Period);
}
protected override void OnBar()
{
var volumne = Math.Floor(Account.Balance * 10 * VolumePercent / 1000) * 1000;
if (_rsi.Result.Last(2) > MaxLevel && _rsi.Result.Last(1) < MaxLevel)
{
ExecuteMarketOrder(TradeType.Sell, Symbol.Name, volumne, "DragonRSI", StopLoss, TakeProfit);
}
if (_rsi.Result.Last(2) < MinLevel && _rsi.Result.Last(1) > MinLevel)
{
ExecuteMarketOrder(TradeType.Buy, Symbol.Name, volumne, "DragonRSI", StopLoss, TakeProfit);
}
}
protected override void OnTick()
{
// Put your core logic here
}
protected override void OnStop()
{
// Put your deinitialization logic here
}
}
}
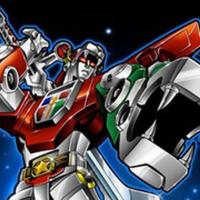
nghiand.amz
Joined on 06.07.2020
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: DragonRSI.algo
- Rating: 0
- Installs: 2126
- Modified: 13/10/2021 09:54
Warning! Running cBots downloaded from this section may lead to financial losses. Use them at your own risk.
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.