Warning! This section will be deprecated on February 1st 2025. Please move all your cBots to the cTrader Store catalogue.
Description
1.Buy order if the parabolic SAR of the previous bar is below the candlestick.
2. A Sell order will be created if the parabolic SAR of the previous bar is above the candlestick.
3. If SAR change position from below to above or vice versa Close position
using System;
using System.Linq;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class DragonPSAR : Robot
{
[Parameter("Min AF", DefaultValue = 0.02, MinValue = 0)]
public double MinAF { get; set; }
[Parameter("Max AF", DefaultValue = 0.2, MinValue = 0)]
public double MaxAF { get; set; }
[Parameter("Initial Volume Percent", DefaultValue = 1, MinValue = 0)]
public double InitialVolumePercent { get; set; }
private ParabolicSAR parabolicSAR;
protected override void OnStart()
{
parabolicSAR = Indicators.ParabolicSAR(MinAF, MaxAF);
}
protected override void OnBar()
{
var volumne = Math.Floor(Account.Balance * 10 * InitialVolumePercent / 1000) * 1000;
var position = Positions.Find("DragonPSAR");
if (parabolicSAR.Result.Last(1) < Bars.Last(1).Close)
{
if (position != null && position.TradeType == TradeType.Sell)
{
position.Close();
}
if (Positions.Count == 0)
{
ExecuteMarketOrder(TradeType.Buy, Symbol.Name, volumne, "DragonPSAR", 0, 0);
}
}
if (parabolicSAR.Result.Last(1) > Bars.Last(1).Close)
{
if (position != null && position.TradeType == TradeType.Buy)
{
position.Close();
}
if (Positions.Count == 0)
{
ExecuteMarketOrder(TradeType.Sell, Symbol.Name, volumne, "DragonPSAR", 0, 0);
}
}
}
protected override void OnTick()
{
// Put your core logic here
}
protected override void OnStop()
{
// Put your deinitialization logic here
}
}
}
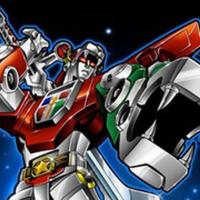
nghiand.amz
Joined on 06.07.2020
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: DragonPSAR.algo
- Rating: 0
- Installs: 2028
- Modified: 13/10/2021 09:54
Warning! Running cBots downloaded from this section may lead to financial losses. Use them at your own risk.
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
RI
Is it possible with a stoploss and a takeprofit of a view pips?
Is it possible with a stoploss and a takeprofit of a view pips?