Warning! This section will be deprecated on February 1st 2025. Please move all your Indicators to the cTrader Store catalogue.
Description
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class HLAveragesTF : Indicator
{
[Parameter("Custom TF")]
public TimeFrame customTimeFrame { get; set; }
[Output("Result1", LineColor = "Green")]
public IndicatorDataSeries Result1 { get; set; }
[Output("Result2", LineColor = "Red")]
public IndicatorDataSeries Result2 { get; set; }
[Output("Result3", LineColor = "Aqua")]
public IndicatorDataSeries Result3 { get; set; }
private Bars customBars;
private int idx;
private int previousIdx;
private int buyPeriod;
private int sellPeriod;
private double buyAverage;
private double sellAverage;
protected override void Initialize()
{
customBars = MarketData.GetBars(customTimeFrame);
}
public override void Calculate(int index)
{
idx = customBars.OpenTimes.GetIndexByTime(Bars.OpenTimes[index]);
if (idx > previousIdx)
{
buyPeriod++;
sellPeriod++;
}
buyAverage = 0;
for (int i = idx - buyPeriod + 1; i <= idx; i++)
buyAverage += customBars.HighPrices[i];
buyAverage = buyAverage / buyPeriod;
sellAverage = 0;
for (int i = idx - sellPeriod + 1; i <= idx; i++)
sellAverage += customBars.LowPrices[i];
sellAverage = sellAverage / sellPeriod;
if (Bars.HighPrices[index] >= buyAverage)
{
buyPeriod = 1;
buyAverage = Bars.HighPrices[index];
}
if (Bars.LowPrices[index] <= sellAverage)
{
sellPeriod = 1;
sellAverage = Bars.LowPrices[index];
}
Result1[index] = sellAverage;
Result2[index] = buyAverage;
Result3[index] = (buyAverage + sellAverage) / 2;
previousIdx = idx;
}
}
}
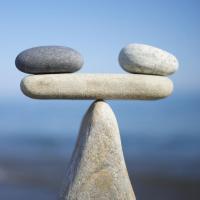
srm_bcn
Joined on 01.09.2019
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: HLAveragesTF.algo
- Rating: 0
- Installs: 1225
- Modified: 13/10/2021 09:54
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
EL
Does this repaint or not? I was used to MT4 but never thought about repainting issue on cTrader. Thank You! :)
Lovely indi. Looks great on my chart.
Hi. Do not repaint.
The market always tries to make new highs and if it does not succeed it tries to make new lows and vice versa.
This indicator tries to represent in a single time frame the support and resistance lines that are in higher time frames.
The visual result does not satisfy me at all, it works better with the market active since with the market stopped, the highs and lows are already known and the indicator anticipates, which does not happen with the market active.
The idea is to find support and resistance levels.
Thanks for your kind question and good luck.