Description
This is a very known indicator used by a Modern adaptation of Wyckoff method known as VSA (Volume Spread Analysis) or VPA (Volume Price Analysis)
Strategies around this indicator are taught by Anna Couling and many Others. The indicator paints Volume activity by colors in the candles, where
Red = Ultra High Volume, DarkOrange = High Volume, Yellow = Average Volume, White = Low Volume, Aqua = Almost no Activity or very low Activity.
Para los que quieran participar de un Grupo CTrader en Español poder compartir estrategias, indicadores e mucho más, aquí abajo les dejo el link.
Grupo CTrader en Español -->> http://t.me/ComunidadCtrader
Grupo CTrader em Português -->> http://t.me/ComunidadeCtrader
using System;
using cAlgo.API;
using cAlgo.API.Indicators;
namespace cAlgo.Indicators
{
[Indicator(IsOverlay = true, AccessRights = AccessRights.None)]
public class CandleVolumeTraderExperto : Indicator
{
[Parameter("MA Length", DefaultValue = 60)]
public int Period { get; set; }
[Parameter("Up Ultra Volume", DefaultValue = "Red")]
public string UpUltraVolume { get; set; }
[Parameter("Down Ultra Volume", DefaultValue = "Red")]
public string DownUltraVolume { get; set; }
[Parameter("Up High Volume", DefaultValue = "DarkOrange")]
public string UpHighVolume { get; set; }
[Parameter("Down High Volume", DefaultValue = "DarkOrange")]
public string DownHighVolume { get; set; }
[Parameter("Up Average Volume", DefaultValue = "Yellow")]
public string UpAverageVolume { get; set; }
[Parameter("Down Average Volume", DefaultValue = "Yellow")]
public string DownAverageVolume { get; set; }
[Parameter("Up Low Volume", DefaultValue = "White")]
public string UpLowVolume { get; set; }
[Parameter("Down Low Volume", DefaultValue = "White")]
public string DownLowVolume { get; set; }
[Parameter("Up Low Activity", DefaultValue = "Aqua")]
public string UpLowActivity { get; set; }
[Parameter("Down Low Activity", DefaultValue = "Aqua")]
public string DownLowActivity { get; set; }
public IndicatorDataSeries dataUltraVolume { get; set; }
public IndicatorDataSeries dataHighVolume { get; set; }
public IndicatorDataSeries dataAverageVolume { get; set; }
public IndicatorDataSeries dataLowVolume { get; set; }
private SimpleMovingAverage smaVol;
private StandardDeviation stdDev;
protected override void Initialize()
{
smaVol = Indicators.SimpleMovingAverage(Bars.TickVolumes, Period);
stdDev = Indicators.StandardDeviation(Bars.TickVolumes, Period, MovingAverageType.Simple);
}
public override void Calculate(int index)
{
double open = Bars.OpenPrices[index];
double high = Bars.HighPrices[index];
double low = Bars.LowPrices[index];
double close = Bars.ClosePrices[index];
double volume = Bars.TickVolumes[index];
double stdbar = (volume - smaVol.Result[index]) / stdDev.Result[index];
if (Bars.ClosePrices[index] >= Bars.OpenPrices[index] && stdbar > 3.5)
{
Chart.SetBarColor(index, UpUltraVolume);
}
else if (Bars.ClosePrices[index] >= Bars.OpenPrices[index] && stdbar > 2.5)
{
Chart.SetBarColor(index, UpHighVolume);
}
else if (Bars.ClosePrices[index] >= Bars.OpenPrices[index] && stdbar > 1)
{
Chart.SetBarColor(index, UpAverageVolume);
}
else if (Bars.ClosePrices[index] >= Bars.OpenPrices[index] && stdbar > -0.5)
{
Chart.SetBarColor(index, UpLowVolume);
}
else if (Bars.ClosePrices[index] >= Bars.OpenPrices[index] && stdbar <= -0.5)
{
Chart.SetBarColor(index, UpLowActivity);
}
if (Bars.ClosePrices[index] < Bars.OpenPrices[index] && stdbar > 3.5)
{
Chart.SetBarColor(index, UpUltraVolume);
}
else if (Bars.ClosePrices[index] >= Bars.OpenPrices[index] && stdbar > 2.5)
{
Chart.SetBarColor(index, DownHighVolume);
}
else if (Bars.ClosePrices[index] < Bars.OpenPrices[index] && stdbar > 1)
{
Chart.SetBarColor(index, DownAverageVolume);
}
else if (Bars.ClosePrices[index] < Bars.OpenPrices[index] && stdbar > -0.5)
{
Chart.SetBarColor(index, DownLowVolume);
}
else if (Bars.ClosePrices[index] < Bars.OpenPrices[index] && stdbar <= -0.5)
{
Chart.SetBarColor(index, DownLowActivity);
}
}
}
}
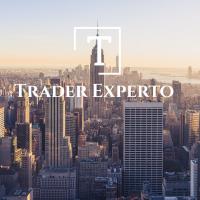
TraderExperto
Joined on 07.06.2019
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Candle Volume .algo
- Rating: 5
- Installs: 3887
- Modified: 13/10/2021 09:54
Error missing dependencies
Can not install “Candle Volume .algo”
Could not find @C:\Users…………Candle Volume Trader Experto.sln
(Swing Gann Experto has same issue - can not install)