Description
Chart Colors has several predefined chart color schemes that you can quickly apply.
Share your color schemes in comments here. I'll will update indicator with shared color schemes.
To do this:
1. Go to Automate
2. Create instance for this indicator
3. Click Print Colors to Log button
4. Copy text from Log tab and paste as a comment here
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
using System.Text.RegularExpressions;
using System.Linq;
namespace cAlgo
{
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class ChartColors : Indicator
{
protected override void Initialize()
{
var rootBorder = new Border
{
HorizontalAlignment = HorizontalAlignment.Left,
BackgroundColor = "#292929",
BorderThickness = "0 0 5 0",
BorderColor = "#525252"
};
var root = new Grid(2, 1)
{
};
root.Rows[1].SetHeightToAuto();
rootBorder.Child = root;
var colorSchemesPanel = CreateColorSchemesPanel();
root.AddChild(colorSchemesPanel, 0, 0);
var printCurrentSchemeButton = CreatePrintCurrentColorSchemeButton();
root.AddChild(printCurrentSchemeButton, 1, 0);
Chart.AddControl(rootBorder);
}
private ControlBase CreateColorSchemesPanel()
{
var stackPanel = new StackPanel
{
Orientation = Orientation.Vertical,
HorizontalAlignment = HorizontalAlignment.Left
};
var schemes = ColorSchemes.Schemes;
for (var i = 0; i < schemes.Length; i++)
{
var scheme = schemes[i];
var isFirst = i == 0;
var isLast = i == schemes.Length - 1;
var tile = new ChartTile(scheme)
{
Margin = new Thickness(0, isFirst ? 8 : 4, 0, isLast ? 8 : 4)
};
tile.Click += Tile_Click;
stackPanel.AddChild(tile);
}
var scrollViewer = new ScrollViewer
{
VerticalScrollBarVisibility = ScrollBarVisibility.Auto,
BackgroundColor = Color.Transparent,
Padding = "12 8 8 8"
};
scrollViewer.Content = stackPanel;
return scrollViewer;
}
private Button CreatePrintCurrentColorSchemeButton()
{
var printCurrentSchemeButton = new Button
{
Margin = "8 4",
Text = "Print Colors to Log"
};
printCurrentSchemeButton.Click += PrintCurrentSchemeButton_Click;
return printCurrentSchemeButton;
}
private void PrintCurrentSchemeButton_Click(ButtonClickEventArgs obj)
{
var colorScheme = GetCurrentColorSchemeText();
Print(colorScheme);
}
private void Tile_Click(ChartColorScheme scheme)
{
ApplyCollorScheme(scheme);
}
private void ApplyCollorScheme(ChartColorScheme scheme)
{
Chart.DisplaySettings.Grid = false;
Chart.DisplaySettings.PeriodSeparators = false;
Chart.ColorSettings.BackgroundColor = scheme.BackgoundColor;
Chart.ColorSettings.ForegroundColor = scheme.ForegroundColor;
Chart.ColorSettings.BullOutlineColor = scheme.BullOutlineColor;
Chart.ColorSettings.BearOutlineColor = scheme.BearOutlineColor;
Chart.ColorSettings.BullFillColor = scheme.BullFillColor;
Chart.ColorSettings.BearFillColor = scheme.BearFillColor;
}
private string GetCurrentColorSchemeText()
{
var colors = new[]
{
Chart.ColorSettings.BackgroundColor.ToHexString(),
Chart.ColorSettings.ForegroundColor.ToHexString(),
Chart.ColorSettings.BullOutlineColor.ToHexString(),
Chart.ColorSettings.BearOutlineColor.ToHexString(),
Chart.ColorSettings.BullFillColor.ToHexString(),
Chart.ColorSettings.BearFillColor.ToHexString()
};
var text = "\"" + string.Join("\",\"", colors) + "\"";
return text;
}
public override void Calculate(int index)
{
}
}
public class ChartColorScheme
{
public Color BackgoundColor { get; set; }
public Color ForegroundColor { get; set; }
public Color BullOutlineColor { get; set; }
public Color BearOutlineColor { get; set; }
public Color BullFillColor { get; set; }
public Color BearFillColor { get; set; }
public Color TickVolumeColor { get; set; }
}
public class ChartTile : CustomControl
{
private static readonly int[][] OhlcPrices = new[]
{
new[]
{
60,
64,
30,
35
},
new[]
{
35,
40,
30,
39
},
new[]
{
37,
50,
33,
47
},
new[]
{
47,
53,
39,
43
},
new[]
{
43,
57,
25,
30
},
new[]
{
38,
53,
34,
43
},
new[]
{
38,
52,
34,
49
},
new[]
{
49,
64,
47,
62
},
new[]
{
62,
77,
58,
73
},
new[]
{
75,
79,
63,
67
},
new[]
{
67,
70,
48,
52
},
new[]
{
52,
53,
40,
43
},
new[]
{
43,
48,
40,
47
},
new[]
{
47,
53,
39,
43
},
new[]
{
43,
55,
40,
46
},
new[]
{
46,
43,
62,
58
},
new[]
{
58,
74,
52,
68
},
new[]
{
72,
87,
72,
84
},
new[]
{
84,
93,
80,
90
}
};
public ChartColorScheme ColorScheme { get; private set; }
public event Action<ChartColorScheme> Click;
public ChartTile(ChartColorScheme colorScheme)
{
var canvas = new Canvas
{
BackgroundColor = colorScheme.BackgoundColor,
Width = 170,
Height = 120
};
var button = new Button
{
Content = canvas,
Padding = 0
};
button.Click += Button_Click;
for (var i = 0; i < OhlcPrices.Length; i++)
{
var ohlc = OhlcPrices[i];
DrawBar(canvas, i, ohlc[0], ohlc[1], ohlc[2], ohlc[3], colorScheme);
}
AddChild(button);
ColorScheme = colorScheme;
}
private void Button_Click(ButtonClickEventArgs obj)
{
if (Click != null)
Click.Invoke(ColorScheme);
}
private void DrawBar(Canvas canvas, int index, int open, int high, int low, int close, ChartColorScheme scheme)
{
var fillColor = close >= open ? scheme.BullFillColor : scheme.BearFillColor;
var outlineColor = close >= open ? scheme.BullOutlineColor : scheme.BearOutlineColor;
var barLeft = 5 + index * 5 + index * 3;
var bodyFill = new Rectangle
{
Left = barLeft + 1,
Bottom = Math.Min(open, close) + 1,
Height = Math.Abs(open - close) - 2,
Width = 4,
FillColor = fillColor
};
var bodyOutline = new Rectangle
{
Left = barLeft,
Bottom = Math.Min(open, close),
Height = Math.Abs(open - close),
Width = 5,
StrokeThickness = 1,
StrokeColor = outlineColor
};
var bottomShadow = new Rectangle
{
Width = 1,
Height = Math.Min(open, close) - low,
FillColor = outlineColor,
Left = barLeft + 2,
Bottom = low
};
var topShadow = new Rectangle
{
Width = 1,
Height = high - Math.Max(open, close),
FillColor = outlineColor,
Left = barLeft + 2,
Bottom = Math.Max(open, close)
};
canvas.AddChild(bodyFill);
canvas.AddChild(bodyOutline);
canvas.AddChild(bottomShadow);
canvas.AddChild(topShadow);
}
}
public static class ColorSchemes
{
private static readonly string[][] SchemesRaw = new[]
{
new[]
{
"#FF18201F",
"#FFB3B3B3",
"#7D44B5FF",
"#7EFF528B",
"#7D44B5FF",
"#7DFF528B"
},
new[]
{
"#424242",
"#E9E9E9",
"#E9E9E9",
"##3299BB",
"#E9E9E9",
"#3299BB"
},
new[]
{
"#292933",
"#BBBBBB",
"#F59C19",
"#F59C19",
"#00FFFFFF",
"#F59C19"
},
new[]
{
"#131722",
"#BBBBBB",
"#53B987",
"#EB4D5C",
"#53B987",
"#EB4D5C"
},
new[]
{
"#FF292929",
"#FFB3B3B3",
"#FF1BB61B",
"#FFDB090D",
"#FF1BB61B",
"#FFDB090D"
},
new[]
{
"#FFFFFFFF",
"#FF000000",
"#FF00843B",
"#FFF15923",
"#FF00843B",
"#FFF15923"
},
new[]
{
"#FFFFFFFF",
"#FF000000",
"#FF000000",
"#FF000000",
"#FFFFFFFF",
"#FF000000"
}
};
public static ChartColorScheme[] Schemes = SchemesRaw.Select(s => { return new ChartColorScheme {BackgoundColor = s[0],ForegroundColor = s[1],BullOutlineColor = s[2],BearOutlineColor = s[3],BullFillColor = s[4],BearFillColor = s[5]}; }).ToArray();
}
}
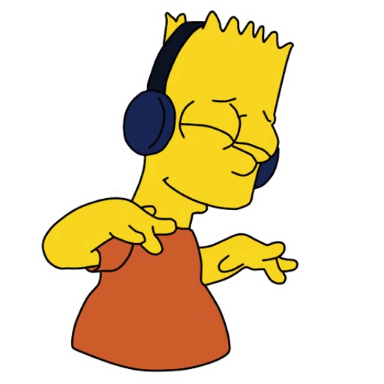
bart1
Joined on 03.08.2017
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Chart Colors.algo
- Rating: 5
- Installs: 1911
- Modified: 13/10/2021 09:54
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.