Description
This robot enters the trend and leaves when the average loses inclination..
FIX - Trailing Stop - 2020-05-28
If you enjoy, donate us any value
using System;
using System.Linq;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class SmartTrendBot : Robot
{
[Parameter("Quantity (Lots)", Group = "Volume", DefaultValue = 0.01, MinValue = 0.01, Step = 0.01)]
public double Quantity { get; set; }
[Parameter("Source", Group = "RSI")]
public DataSeries Source { get; set; }
[Parameter("Periods", Group = "RSI", DefaultValue = 19)]
public int Periods { get; set; }
[Parameter("MME Medium", Group = "RSI", DefaultValue = 21)]
public int p_mmeMedium { get; set; }
[Parameter("Entry Inclination", Group = "RSI", DefaultValue = 20)]
public int inclinacao_ent { get; set; }
[Parameter("Exit Inclination", Group = "RSI", DefaultValue = 10)]
public int inclinacao_sai { get; set; }
[Parameter("Include Trailing Stop", Group = "Trailing Stop", DefaultValue = false)]
public bool IncludeTrailingStop { get; set; }
[Parameter("Trailing Stop Trigger (pips)", Group = "Trailing Stop", DefaultValue = 5)]
public int TrailingStopTrigger { get; set; }
[Parameter("Trailing Stop Step (pips)", Group = "Trailing Stop", DefaultValue = 1)]
public int TrailingStopStep { get; set; }
private RelativeStrengthIndex rsi;
private double volumeInUnits;
private MovingAverage mmeMedium;
private double inclinacaoMme;
protected override void OnStart()
{
volumeInUnits = Symbol.QuantityToVolumeInUnits(Quantity);
mmeMedium = Indicators.MovingAverage(Bars.ClosePrices, p_mmeMedium, MovingAverageType.Exponential);
rsi = Indicators.RelativeStrengthIndex(Source, Periods);
}
protected override void OnTick()
{
if (IncludeTrailingStop)
{
SetTrailingStop();
}
}
protected override void OnBar()
{
inclinacaoMme = (((mmeMedium.Result.LastValue * 100) / mmeMedium.Result.Last(2)) - 100) * 360;
foreach (Position position in Positions)
{
if (position.Label.Equals("Trend Buy"))
{
if (inclinacaoMme < inclinacao_sai)
ClosePosition(position);
}
else if (position.Label.Equals("Trend Sell"))
{
if (inclinacaoMme > (inclinacao_sai * -1))
ClosePosition(position);
}
}
if (rsi.Result.LastValue >= 20 && rsi.Result.LastValue <= 80)
{
if (Bars.LastBar.Open >= Bars.Last(1).Close)
{
if (inclinacaoMme >= inclinacao_ent)
{
ExecuteMarketOrder(TradeType.Buy, SymbolName, volumeInUnits, "Trend Buy");
}
}
else if (Bars.LastBar.Open < Bars.Last(1).Close)
{
if (inclinacaoMme <= (inclinacao_ent * -1))
{
ExecuteMarketOrder(TradeType.Sell, SymbolName, volumeInUnits, "Trend Sell");
}
}
}
}
private void SetTrailingStop()
{
var sellPositions = Positions.FindAll("Trend Sell", SymbolName, TradeType.Sell);
foreach (Position position in sellPositions)
{
double distance = position.EntryPrice - Symbol.Ask;
if (distance < TrailingStopTrigger * Symbol.PipSize)
continue;
double newStopLossPrice = Symbol.Ask + TrailingStopStep * Symbol.PipSize;
if (position.StopLoss == null || newStopLossPrice < position.StopLoss)
{
ModifyPosition(position, newStopLossPrice, position.TakeProfit);
}
}
var buyPositions = Positions.FindAll("Trend Buy", SymbolName, TradeType.Buy);
foreach (Position position in buyPositions)
{
double distance = Symbol.Bid - position.EntryPrice;
if (distance < TrailingStopTrigger * Symbol.PipSize)
continue;
double newStopLossPrice = Symbol.Bid - TrailingStopStep * Symbol.PipSize;
if (position.StopLoss == null || newStopLossPrice > position.StopLoss)
{
ModifyPosition(position, newStopLossPrice, position.TakeProfit);
}
}
}
}
}
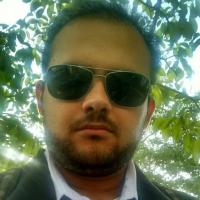
CabralTrader
Joined on 10.03.2020
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Smart Trend Bot.algo
- Rating: 5
- Installs: 3121
- Modified: 13/10/2021 09:54
Warning! Running cBots downloaded from this section may lead to financial losses. Use them at your own risk.
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
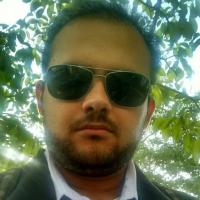
Man, I got it right, download the new version and test again, please.
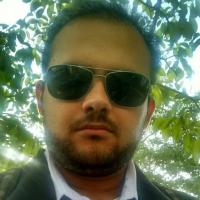
I will see the trailing stop, follow here that I think that in one of the days I post the correction in the robots that I published
K0
Hi CabralTrader.
Thank you for providing a useful bot.
But Trailing Stop doesn't seem to work, is it just me?
please , what is the difference between the smart and trend cbots.