Description
This robot performs operations when the candlestick opens with a value or equal to the simple average of 10 periods based on the lows, and closes orders with the same average only calculated with the lows.
Good results with larger H1 graphics
v2 - Added Take Profit and Stop Loss params
using System;
using System.Linq;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class TenMovingAverage : Robot
{
[Parameter("Quantity (Lots)", Group = "Volume", DefaultValue = 0.01, MinValue = 0.01, Step = 0.01)]
public double Quantity { get; set; }
[Parameter("Enable TakeProfit?", Group = "TakeProfit", DefaultValue = true)]
public bool useTakeProfit { get; set; }
[Parameter("Take Profit", Group = "TakeProfit", DefaultValue = 1, MinValue = 1, Step = 1)]
public double takeProfit { get; set; }
[Parameter("Enable Stop Loss?", Group = "StopLoss", DefaultValue = true)]
public bool useStopLoss { get; set; }
[Parameter("Stop Loss", Group = "StopLoss", DefaultValue = 1, MinValue = 1, Step = 1)]
public double stopLoss { get; set; }
private MovingAverage tenMinimum;
private MovingAverage tenMaximum;
private double volumeInUnits;
protected override void OnStart()
{
volumeInUnits = Symbol.QuantityToVolumeInUnits(Quantity);
tenMinimum = Indicators.MovingAverage(Bars.LowPrices, 10, MovingAverageType.Simple);
tenMaximum = Indicators.MovingAverage(Bars.HighPrices, 10, MovingAverageType.Simple);
}
protected override void OnBar()
{
if (Positions.Count() > 0)
{
if (Bars.LastBar.High >= tenMaximum.Result.LastValue && !useTakeProfit)
{
foreach (var position in Positions)
{
ClosePosition(position);
}
}
}
if (Bars.LastBar.Close <= tenMinimum.Result.LastValue)
{
ExecuteMarketOrder(TradeType.Buy, SymbolName, volumeInUnits, "TenMov", (useStopLoss ? stopLoss : double.MaxValue), (useTakeProfit ? takeProfit : double.MaxValue));
}
}
}
}
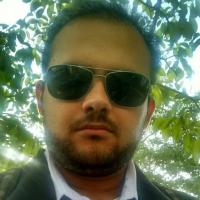
CabralTrader
Joined on 10.03.2020
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: TenMovingAverage.algo
- Rating: 5
- Installs: 2596
- Modified: 13/10/2021 09:54
Comments
Hi CabralTrader
The bot works in backtesting but not when running on a demo account.
It fails with "technical error" could you please assist?
Excellent Bot, Thanks
as in my study, since 2016, there is someone who uses 4 Ma, Multiangle 1 + 1 and Triangle .. I know you can remove or change that movement in your script to make sure no one uses it .. script. Script .. help me please um. . this is my mistake for changing it. I do not know if it is wrong. And i Don't know anything on how to write it. . i stop and telling u once i realise it..
another reason I remind you here is because of my concern for what I have done , which is something I do not know, Plus i am new here.. in fact it is a mistake and i am newbie.. I have told you before .. and I hope you can forgive me, because this my third reminder to you to agknowledge. .. and to the trader c please delete this .. no wonder that the script brings the most ..
I'm sorry Sir.. can u remove this?? I realised it was a mistake before.. but i'm not using it anymore but i Don't want anyone are using it too...
i dont have enough fund and margin to start trade with this bot if it goes opposite of the order, so it makes me stop out all the times due to lack of funds.. So if u could help me to put a limit order or stop order on it, it will be much better for me and maybe for us to use this, im new in this, so i didnt know waht to do, and how to manage all this code.. it is usefell for a few simbol, but as i can say, EU,GU, and some other Simbol are Perfect to use this,.. it need a big fund to use this, but im confidently suggesting others that it will give u a 100% WINNING TRADE WITHOUT ANY LOSE..
- Estimate = >laverage for
0.01 is a $1000USD
0.10 is $10,000USD
1 Lot is $100,000USD
if u can make a few change on it, it'll be much better... in order to put a Limit Order on every signal it received, this bot will be greater!!! I hope u can do it and make an improvement on it.
I've been inspired from your bot, which i've made some change on it. And here it is,
A 100% Winning Bots and a '0' Losing Trade...
using System;
using System.Linq;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class FourMovingAverage : Robot
{
[Parameter("Quantity (Lots)", Group = "Volume", DefaultValue = 0.01, MinValue = 0.01, Step = 0.01)]
public double Quantity { get; set; }
public double LastValue { get; set; }
private MovingAverage FourMinimum;
private MovingAverage FourMaximum;
private double volumeInUnits;
protected override void OnStart()
{
volumeInUnits = Symbol.QuantityToVolumeInUnits(Quantity);
FourMinimum = Indicators.MovingAverage(Bars.LowPrices, 4, MovingAverageType.Simple);
FourMaximum = Indicators.MovingAverage(Bars.HighPrices, 4, MovingAverageType.Simple);
}
protected override void OnBar()
{
if (Positions.Count() > 1)
{
if (Bars.LastBar.High >= FourMaximum.Result.LastValue)
{
foreach (var position in Positions)
{
if (position.Pips > 0)
{
ClosePosition(position);
}
}
}
}
if (Bars.LastBar.Close >= FourMinimum.Result.LastValue)
{
ExecuteMarketOrder(TradeType.Buy, Symbol, 1000, "Buy");
}
else if (Bars.LastBar.Low <= FourMaximum.Result.LastValue)
{
ExecuteMarketOrder(TradeType.Sell, Symbol, 1000, "Sell");
}
{
foreach (var position in Positions)
{
if (position.Pips > 0)
{
ClosePosition(position);
}
}
}
}
}
}
(^_^)
Hi there, i've been playing with this indicator a bit...
A trailing stop loss would be another nice addition. I tried to add this feature and it works in backtest but does not place any trades in demo mode.
Could this be added or if i send the code to you could you tweak my errors and u[date on here please?
Kind regards.