Description
I made this indicator for my cBot so, I thought maybe others will find this useful.
How it works:
ATR Value * Input Multiply and instead of changing every value on each bar, it acts as a trailing with a little bit of calculation to switch it up to the top or to the bottom of the prices
Cheers
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class ATRTrailing : Indicator
{
[Parameter("Peroids", DefaultValue = 14, MinValue = 1, MaxValue = 100)]
public int Peroid { get; set; }
[Parameter("ATR Multiply By", DefaultValue = 2.0, MinValue = 0.1, MaxValue = 100.0)]
public double Multiply { get; set; }
[Parameter("ATR MA Type", DefaultValue = MAType.Exponential)]
public MAType MaType { get; set; }
public enum MAType
{
Simple,
Exponential,
Time_Series,
Triangular,
VIDYA,
Weighted,
Wilder_Smoothing
}
[Parameter("Show ATR Trailing", DefaultValue = true)]
public bool ShowATRT { get; set; }
[Parameter("Show ATR Trailing S & R", DefaultValue = false)]
public bool BothSides { get; set; }
[Output("ATR T", LineStyle = LineStyle.Solid, LineColor = "FF68D0F7")]
public IndicatorDataSeries Trailing { get; set; }
[Output("ATR T Sup", LineStyle = LineStyle.Dots, LineColor = "FF65FE66")]
public IndicatorDataSeries Trailing2 { get; set; }
[Output("ATR T Resist", LineStyle = LineStyle.Dots, LineColor = "FFFF6666")]
public IndicatorDataSeries Trailing1 { get; set; }
AverageTrueRange ATR { get; set; }
bool Switch = false;
double T1LastValue = 0.0;
double T2LastValue = 0.0;
protected override void Initialize()
{
MovingAverageType ATRMAType;
if (MaType == MAType.Simple)
ATRMAType = MovingAverageType.Simple;
else if (MaType == MAType.Exponential)
ATRMAType = MovingAverageType.Exponential;
else if (MaType == MAType.Time_Series)
ATRMAType = MovingAverageType.TimeSeries;
else if (MaType == MAType.Triangular)
ATRMAType = MovingAverageType.Triangular;
else if (MaType == MAType.VIDYA)
ATRMAType = MovingAverageType.VIDYA;
else if (MaType == MAType.Weighted)
ATRMAType = MovingAverageType.Weighted;
else
ATRMAType = MovingAverageType.WilderSmoothing;
ATR = Indicators.AverageTrueRange(Peroid, ATRMAType);
}
public override void Calculate(int index)
{
var ClosePrice = Bars.ClosePrices.LastValue;
var ATRTop = ClosePrice + (ATR.Result[index] * Multiply);
var ATRBot = ClosePrice + (-ATR.Result[index] * Multiply);
var PriceBetweenHighAndLow = (Bars.HighPrices[index] + Bars.LowPrices[index]) / 2;
var _Trailing1 = T1LastValue > ATRTop ? ATRTop : T1LastValue < PriceBetweenHighAndLow ? PriceBetweenHighAndLow : T1LastValue;
var _Trailing2 = T2LastValue < ATRBot ? ATRBot : T2LastValue > PriceBetweenHighAndLow ? PriceBetweenHighAndLow : T2LastValue;
if (index == 0)
_Trailing1 = _Trailing2 = PriceBetweenHighAndLow;
if (ShowATRT)
{
if (_Trailing1 <= PriceBetweenHighAndLow)
Switch = true;
else if (_Trailing2 >= PriceBetweenHighAndLow)
Switch = false;
if (!Switch)
Trailing[index] = _Trailing1;
else
Trailing[index] = _Trailing2;
}
if (BothSides)
{
Trailing1[index] = _Trailing1;
Trailing2[index] = _Trailing2;
}
T1LastValue = _Trailing1;
T2LastValue = _Trailing2;
}
}
}
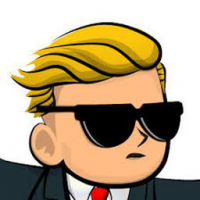
Kbee
Joined on 28.03.2020
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: ATR Trailing.algo
- Rating: 5
- Installs: 3811
- Modified: 13/10/2021 09:55
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
Be blessed