Description
Volume indicator of PVSRA
using System;
using System.Linq;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
namespace cAlgo
{
[Levels(0)]
[Indicator(IsOverlay = false, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class PVSRAVolume : Indicator
{
[Parameter("LookBack", DefaultValue = 10)]
public int LookBack { get; set; }
[Output("Bullish Normal", LineColor = "EE9FA0A2", PlotType = PlotType.Histogram, Thickness = 5)]
public IndicatorDataSeries BullNormalVolume { get; set; }
[Output("Bearish Normal", LineColor = "EE6A6A75", PlotType = PlotType.Histogram, Thickness = 5)]
public IndicatorDataSeries BearNormalVolume { get; set; }
[Output("Bullish Climax", LineColor = "EE1FC047", PlotType = PlotType.Histogram, Thickness = 5)]
public IndicatorDataSeries BullClimaxVolume { get; set; }
[Output("Bearish Climax", LineColor = "EEE00106", PlotType = PlotType.Histogram, Thickness = 5)]
public IndicatorDataSeries BearClimaxVolume { get; set; }
[Output("Bullish Rising", LineColor = "EE1188FF", PlotType = PlotType.Histogram, Thickness = 5)]
public IndicatorDataSeries BullRisingVolume { get; set; }
[Output("Bearish Rising", LineColor = "EEAD0FFF", PlotType = PlotType.Histogram, Thickness = 5)]
public IndicatorDataSeries BearRisingVolume { get; set; }
protected override void Initialize()
{
}
public override void Calculate(int index)
{
var open = Bars.OpenPrices[index];
var high = Bars.HighPrices[index];
var low = Bars.LowPrices[index];
var close = Bars.ClosePrices[index];
var volume = Bars.TickVolumes[index];
var isBullish = close > open;
double av = 0;
int va = 0;
for (int n = 1; n <= LookBack; n++)
{
av += Bars.TickVolumes.Last(n);
}
av = av / LookBack;
var range = high - low;
var val = volume * range;
double hiValue = 0;
for (int n = 1; n <= LookBack; n++)
{
var temp = Bars.TickVolumes.Last(n) * (Bars.HighPrices.Last(n) - Bars.LowPrices.Last(n));
if (temp >= hiValue)
hiValue = temp;
}
if (val >= hiValue || volume >= av * 2)
{
va = 1;
}
if (va == 0 && volume >= av * 1.5)
{
va = 2;
}
switch (va)
{
case 0:
if (isBullish)
BullNormalVolume[index] = volume;
else
BearNormalVolume[index] = volume;
break;
case 1:
if (isBullish)
BullClimaxVolume[index] = volume;
else
BearClimaxVolume[index] = volume;
break;
case 2:
if (isBullish)
BullRisingVolume[index] = volume;
else
BearRisingVolume[index] = volume;
break;
}
}
private static T GetAttributeFrom<T>(object instance, string propertyName)
{
var attrType = typeof(T);
var property = instance.GetType().GetProperty(propertyName);
return (T)property.GetCustomAttributes(attrType, false).GetValue(0);
}
}
}
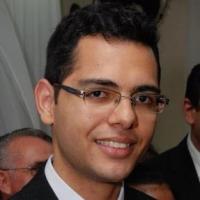
reyx
Joined on 16.02.2019
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: PVSRA Volume.algo
- Rating: 5
- Installs: 3014
- Modified: 13/10/2021 09:54
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
TOPPPPP VALEU
EXATAMENTE O QUE EU ESTAVA PROCURANDO.