Description
A simple indicator that show account live numbers in chart
using System;
using System.Globalization;
using System.Linq;
using System.Collections.Generic;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = true, TimeZone = TimeZones.ESouthAmericaStandardTime, AccessRights = AccessRights.None)]
public class AccountSummary : Indicator
{
[Parameter("Stop Loss Risk %", DefaultValue = 5)]
public int stopLossRiskPercent { get; set; }
[Parameter("Stop Loss in Pips", DefaultValue = 25)]
public int stopLossInPips { get; set; }
private AverageTrueRange atr;
protected override void Initialize()
{
atr = Indicators.AverageTrueRange(14, MovingAverageType.Exponential);
}
public override void Calculate(int index)
{
double spread = 0;
double swap = 0;
string textPositions = "\r\n";
spread = Math.Round(Symbol.Spread / Symbol.PipSize, 5);
var history = History.ToList();
double totalProfit = history.Sum(t => t.NetProfit);
double totalProfitToday = history.Where(t => t.ClosingTime.DayOfYear == Time.DayOfYear).Sum(t => t.NetProfit);
double yesterdayProfit = totalProfit - totalProfitToday;
double initialBalance = Account.Balance - totalProfit;
double totalGain = totalProfit / initialBalance;
double totalGainToday = 0.0;
if (totalProfitToday != 0)
{
totalGainToday = totalProfitToday / (initialBalance + yesterdayProfit);
}
double costPerPip = (double)((int)(Symbol.PipValue * 10000000)) / 100;
double positionSizeForRisk = (Account.Balance * stopLossRiskPercent / 100) / (stopLossInPips * costPerPip);
string positionSizeForRiskText = string.Format("{3} lot{4} [{0}% x {1}pip{2}]", stopLossRiskPercent, stopLossInPips, stopLossInPips > 1 ? "s" : "", Math.Round(positionSizeForRisk, 2), positionSizeForRisk > 1 ? "s" : "");
CultureInfo culture = CultureInfo.CreateSpecificCulture("en-US");
culture.NumberFormat.CurrencyNegativePattern = 1;
Dictionary<string, double> symbolValues = new Dictionary<string, double>();
foreach (var position in Positions)
{
var symbolValue = symbolValues.FirstOrDefault(t => t.Key == position.SymbolName);
if (symbolValue.Key == null)
{
symbolValues.Add(position.SymbolName, position.NetProfit);
}
else
{
symbolValues[symbolValue.Key] += position.NetProfit;
}
swap += position.Swap;
}
foreach (var symbolValue in symbolValues.OrderBy(t => t.Key))
{
textPositions += string.Format(culture, "\r\n{0}: {1:C}", symbolValue.Key, symbolValue.Value);
}
var atrValue = Math.Round(atr.Result.LastValue, 5) * (SymbolName.Contains("JPY") ? 100 : 10000);
var labels = new[]
{
"Spread:\t {0}",
"Margin:\t {1:0.00%}",
"Total:\t {2:C} [{3:0.000%}]",
"Today:\t {4:C} [{5:0.000%}]",
"Balance:\t {6:C}",
"Equity:\t {7:C}",
"Swap:\t {8:C}",
"Profit:\t {9:C}",
"Lot Size:\t {10}",
"ATR:\t {11:0.0}"
};
var formattedString = string.Join("\r\n", labels);
string text = string.Format(culture, formattedString, spread, (Account.MarginLevel ?? 0) / 100, totalProfit, totalGain, totalProfitToday, totalGainToday, Account.Balance, Account.Equity,
swap, Account.UnrealizedNetProfit, positionSizeForRiskText, atrValue);
Chart.DrawStaticText("Account Summary", "Account Summary:", VerticalAlignment.Top, HorizontalAlignment.Left, Color.MediumSpringGreen);
Chart.DrawStaticText("Account Text", string.Concat("\r\n", text, textPositions), VerticalAlignment.Top, HorizontalAlignment.Left, Color.White);
}
}
}
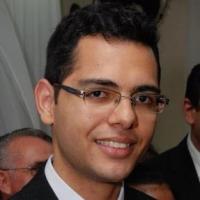
reyx
Joined on 16.02.2019
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Account Summary.algo
- Rating: 0
- Installs: 1519
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.