Description
The indicator normalises all standard moving averages as well as Tilson T3 Moving Average
Standard moving averages can be smoothed by the MA Factor (values range: 1-6)
For example, EMA with Factor 1 is normal EMA, by using factor 2 we get EMA of EMA,
by using factor 3 gives EMA of EMA of EMA etc.
There is also a feature to add a final smoothing. All smoothing features apply only to MAs, not to T3
The idea of the indicator is to capture price cycles using two different cycle periods. Shorter cycle show
short/medium-term price cycles as longer cycle confirms the trend direction and longer-term price cycles.
// ----------------------------------------------------------------------------------------------------
// ----------------------------------------------------------------------------------------------------
/*
The indicator normalises all standard moving averages as well as Tilson T3 Moving Average
Standard moving averages can be smoothed by the MA Factor (values range: 1-6)
For example, EMA with Factor 1 is normal EMA, by using factor 2 we get EMA of EMA,
by using factor 3 gives EMA of EMA of EMA etc.
There is also a feature to add a final smoothing. All smoothing features apply only to MAs, not to T3
The idea of the indicator is to capture price cycles using two different cycle periods. Shorter cycle show
short/medium-term price cycles as longer cycle confirms the trend direction and longer-term price cycles.
*/
// ----------------------------------------------------------------------------------------------------
// ----------------------------------------------------------------------------------------------------
// Version Updates:
// 2020.1.9 Added all moving averages and ATR Channels and EMA factor feature (EMA, TEMA, DEMA etc till 6th factor)
//
// 2020/1/19 - Added Slope LookBack feature. Purpose to filter out short slope changes by checking if slope color persist throughout the lookback period. E.g. we're filtering out small red/green paches
//
// 2020/2/4 - Added s2nd MA line & 2nd MA Factor & 2nd Final Smoothing
// ----------------------------------------------------------------------------------------------------
// ----------------------------------------------------------------------------------------------------
using System;
using System.Linq;
using System.Text;
using System.Threading;
using System.Collections.Generic;
using System.Reflection;
using System.Globalization;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
using System.IO;
namespace cAlgo.Indicators
{
[Indicator(IsOverlay = false, AccessRights = AccessRights.None)]
public class MultiMANormalised : Indicator
{
[Parameter("Fibonacci: ", Group = "-- For Reference Only ---", DefaultValue = "5,8,13,21,34,55,89,144,233,377,610")]
public string indicatorinfo { get; set; }
[Parameter()]
public DataSeries Source { get; set; }
[Parameter("Method", Group = " --- T3/MA Method ---", DefaultValue = MovingAverageType.Simple)]
public MovingAverageType MAType { get; set; }
[Parameter("1st Period ", Group = " --- T3/MA Period ---", DefaultValue = 21, MinValue = 1)]
public int Period { get; set; }
[Parameter("2nd Period, !Void if 0!", Group = " --- T3/MA Period ---", DefaultValue = 55, MinValue = 0)]
public int Period2 { get; set; }
[Parameter("Use T3", Group = " --- T3 ---", DefaultValue = false)]
public bool UseT3 { get; set; }
[Parameter("Volume Factor (T3 only) ", Group = " --- T3 ---", DefaultValue = 0.7)]
public double Volume_Factor { get; set; }
[Parameter("1st MA Factor (1-6) ", Group = " --- MA Smoothing Factor ---", DefaultValue = 3, MinValue = 1, MaxValue = 6)]
public int MAFactor { get; set; }
[Parameter("2nd MA Factor (1-6) ", Group = " --- MA Smoothing Factor ---", DefaultValue = 6, MinValue = 1, MaxValue = 6)]
public int MAFactor2 { get; set; }
[Parameter("1St Final Smooting ", Group = " --- Final MA Smoothing ---", DefaultValue = 13)]
public int MASmoothPeriod { get; set; }
[Parameter("2nd Final Smooting ", Group = " --- Final MA Smoothing ---", DefaultValue = 34)]
public int MASmoothPeriod2 { get; set; }
[Output("MA steady", LineColor = "Gray", PlotType = PlotType.DiscontinuousLine, Thickness = 3)]
public IndicatorDataSeries steady { get; set; }
[Output("MA rise", LineColor = "Lime", PlotType = PlotType.DiscontinuousLine, Thickness = 3)]
public IndicatorDataSeries rise { get; set; }
[Output("MA fall", LineColor = "Red", PlotType = PlotType.DiscontinuousLine, Thickness = 3)]
public IndicatorDataSeries fall { get; set; }
[Output("MA steady 2", LineColor = "Gray", PlotType = PlotType.DiscontinuousLine, Thickness = 5)]
public IndicatorDataSeries steady2 { get; set; }
[Output("MA fall 2", LineColor = "DarkMagenta", PlotType = PlotType.DiscontinuousLine, Thickness = 5)]
public IndicatorDataSeries fall2 { get; set; }
[Output("T3MA rise 2", LineColor = "Green", PlotType = PlotType.DiscontinuousLine, Thickness = 5)]
public IndicatorDataSeries rise2 { get; set; }
[Output("Channel Up", LineColor = "Yellow", Thickness = 1, LineStyle = LineStyle.Lines)]
public IndicatorDataSeries ChannelUp { get; set; }
[Output("Channel Down", LineColor = "Yellow", Thickness = 1, LineStyle = LineStyle.Lines)]
public IndicatorDataSeries ChannelDw { get; set; }
[Output("0 Level", LineColor = "Yellow", Thickness = 1)]
public IndicatorDataSeries Level0 { get; set; }
private MovingAverage ma1;
private MovingAverage ma2;
private MovingAverage ma3;
private MovingAverage ma4;
private MovingAverage ma5;
private MovingAverage ma6;
private MovingAverage ma12;
private MovingAverage ma22;
private MovingAverage ma32;
private MovingAverage ma42;
private MovingAverage ma52;
private MovingAverage ma62;
private MovingAverage MaSmooth;
private MovingAverage MaSmooth2;
private IndicatorDataSeries val2;
private IndicatorDataSeries val1;
int lastindex = 0;
protected override void Initialize()
{
ma1 = Indicators.MovingAverage(Source, Period, MAType);
ma2 = Indicators.MovingAverage(ma1.Result, Period, MAType);
ma3 = Indicators.MovingAverage(ma2.Result, Period, MAType);
ma4 = Indicators.MovingAverage(ma3.Result, Period, MAType);
ma5 = Indicators.MovingAverage(ma4.Result, Period, MAType);
ma6 = Indicators.MovingAverage(ma5.Result, Period, MAType);
ma12 = Indicators.MovingAverage(Source, Period2, MAType);
ma22 = Indicators.MovingAverage(ma12.Result, Period2, MAType);
ma32 = Indicators.MovingAverage(ma22.Result, Period2, MAType);
ma42 = Indicators.MovingAverage(ma32.Result, Period2, MAType);
ma52 = Indicators.MovingAverage(ma42.Result, Period2, MAType);
ma62 = Indicators.MovingAverage(ma52.Result, Period2, MAType);
val2 = CreateDataSeries();
val1 = CreateDataSeries();
MaSmooth = Indicators.MovingAverage(val1, MASmoothPeriod, MAType);
MaSmooth2 = Indicators.MovingAverage(val2, MASmoothPeriod2, MAType);
}
public override void Calculate(int index)
{
if (IsLastBar)
{
if (index != lastindex)
lastindex = index;
else
return;
}
val1[index] = ma(index);
steady[index] = UseT3 ? (maketema(index) - maketema(index - 1)) / 2 : (MaSmooth.Result[index] - MaSmooth.Result[index - 1]) / 2;
if (steady.IsFalling())
fall[index] = steady[index];
if (steady.IsRising())
rise[index] = steady[index];
if (Period2 > 0)
{
val2[index] = UseT3 ? maketema2(index) : _ma2(index);
steady2[index] = (MaSmooth2.Result[index] - MaSmooth2.Result[index - 1]) / 2;
if (steady2.IsFalling())
fall2[index] = steady2[index];
if (steady2.IsRising())
rise2[index] = steady2[index];
}
Level0[index] = 0;
}
double maketema(int index)
{
double b, b2, b3, c1, c2, c3, c4;
double result = 0;
b = Volume_Factor;
b2 = Math.Pow(b, 2);
// Volume Factor Squared
b3 = Math.Pow(b, 3);
// Volume Factor Cubed
c1 = -b3;
c2 = 3 * b2 + 3 * b3;
c3 = -6 * b2 - 3 * b - 3 * b3;
c4 = 1 + 3 * b + b3 + 3 * b2;
result = c1 * ma6.Result[index] + c2 * ma5.Result[index] + c3 * ma4.Result[index] + c4 * ma3.Result[index];
return result;
}
double maketema2(int index)
{
double b, b2, b3, c1, c2, c3, c4;
double result = 0;
b = Volume_Factor;
b2 = Math.Pow(b, 2);
// Volume Factor Squared
b3 = Math.Pow(b, 3);
// Volume Factor Cubed
c1 = -b3;
c2 = 3 * b2 + 3 * b3;
c3 = -6 * b2 - 3 * b - 3 * b3;
c4 = 1 + 3 * b + b3 + 3 * b2;
result = c1 * ma62.Result[index] + c2 * ma52.Result[index] + c3 * ma42.Result[index] + c4 * ma32.Result[index];
return result;
}
double ma(int index)
{
double result = 0;
if (MAFactor == 6)
result = (ma6.Result[index] + ma5.Result[index] + ma4.Result[index] + ma3.Result[index] + ma2.Result[index] + ma1.Result[index]) / 6;
if (MAFactor == 5)
result = (ma5.Result[index] + ma4.Result[index] + ma3.Result[index] + ma2.Result[index] + ma1.Result[index]) / 5;
if (MAFactor == 4)
result = (ma4.Result[index] + ma3.Result[index] + ma2.Result[index] + ma1.Result[index]) / 4;
if (MAFactor == 3)
result = (ma3.Result[index] + ma2.Result[index] + ma1.Result[index]) / 3;
if (MAFactor == 2)
result = (ma2.Result[index] + ma1.Result[index]) / 2;
if (MAFactor == 1)
result = ma1.Result[index];
return result;
}
double _ma2(int index)
{
double result2 = 0;
if (MAFactor2 == 6)
result2 = (ma62.Result[index] + ma52.Result[index] + ma42.Result[index] + ma32.Result[index] + ma22.Result[index] + ma12.Result[index]) / 6;
if (MAFactor2 == 5)
result2 = (ma52.Result[index] + ma42.Result[index] + ma32.Result[index] + ma22.Result[index] + ma12.Result[index]) / 5;
if (MAFactor2 == 4)
result2 = (ma42.Result[index] + ma32.Result[index] + ma22.Result[index] + ma12.Result[index]) / 4;
if (MAFactor2 == 3)
result2 = (ma32.Result[index] + ma22.Result[index] + ma12.Result[index]) / 3;
if (MAFactor2 == 2)
result2 = (ma22.Result[index] + ma12.Result[index]) / 2;
if (MAFactor2 == 1)
result2 = ma12.Result[index];
return result2;
}
}
}
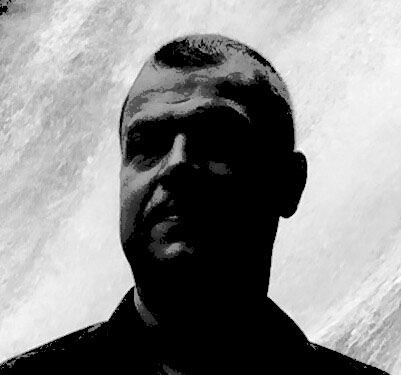
jani
Joined on 05.04.2019
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Multi MA Normalised.algo
- Rating: 3.75
- Installs: 2307
- Modified: 13/10/2021 09:54
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
I love this indicator.
You mind adding notification gateway to it? Such as Telegram notification and email