Description
This is a modified version of the traditional MACD indicator.
Cut-Off levels indicate levels where MACD and signal line difference (=histogram) is greater than the set level.
I have also added a feature to count MACD from any standard moving average.
Version update 2020/1/20
* Added tick volume feature. Increasing tick volume factor increases tick volume relative weight when calculating the MAs
* Replaced lookback with MA smoothing feature. LB and SMA accomplish the same thing, MA smoothing now more versatile.
Version update 2020/2/3
* Added zero lag feature
Version update 2020/2/10
* Removed Tick Volume, error in logic
=====================================================================================================
SETTINGS
=====================================================================================================
Traditional MACD:
MACD periods: 12 & 26 // Signal period: 9 // MA Type: Exponential
Linda Raschke:
MACD periods: 3 & 10 // Signal period: 16 // MA Type: Simple
Awesome Oscillator:
MACD periods: 5 & 34 // Signal period:N/A // MA Type: Simple
// -------------------------------------------------------------------------------------------------
//
// This code is a cAlgo API MACD Crossover indicator provided by njardim@email.com on Dec 2015.
//
// Based on the original MACD Crossover indicator from cTrade.
//
// -------------------------------------------------------------------------------------------------
/*
* Indicator was modified by Telegram @Fibonacci2011 on Jan 2020.
*
* Modifications:
*
* 2020/1/21 Added MAType. Levels and Lookback feature
* 2020/1/21 Added tick volume feature
* 2020/1/22 Replaced lookback with MA smoothing feature
* 2020/1/22 Added zero lag feature
* 2020/2/10 Removed Tick Volume, error in logic
This is a modified version of the traditional MACD indicator.
Ma Smoothing attempts to show longer-term momentum and cycles better than the standard MACD.
Cut-Off levels indicate levels where MACD and signal line difference is greater than the level.
Tick volume feature takes into consideration the changes in tick volume. Increasing
Tick Volume Factor will increasi tick volume feature relative weight
=====================================================================================================
SETTINGS
=====================================================================================================
Traditional MACD:
MACD periods: 12 & 26 // Signal period: 9 // MA Type: Exponential
Linda Raschke:
MACD periods: 3 & 10 // Signal period: 16 // MA Type: Simple
Awesome Oscillator:
MACD periods: 5 & 34 // Signal period:N/A // MA Type: Simple
*/
// -------------------------------------------------------------------------------------------------
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = false, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class MACDCrossover : Indicator
{
// public MACDCrossover MACDCrossover;
[Parameter()]
public DataSeries Source { get; set; }
[Parameter("MA Type", Group = "--- MA Type ---", DefaultValue = MovingAverageType.Exponential)]
public MovingAverageType MAType { get; set; }
[Parameter("Long Cycle", Group = "--- Period ---", DefaultValue = 26)]
public int LongCycle { get; set; }
[Parameter("Short Cycle", Group = "--- Period ---", DefaultValue = 12)]
public int ShortCycle { get; set; }
[Parameter("Signal Periods", Group = "--- Period ---", DefaultValue = 9)]
public int Periods { get; set; }
[Parameter("Use Zero Lag MACD ", Group = "--- Zero Lag ---", DefaultValue = false)]
public bool UseZeroLag { get; set; }
// ---------------
[Parameter("Smoothing MA Period, Void if 0 ", Group = "--- Smoothing MA ---", DefaultValue = 0, MinValue = 0)]
public int SmoothPeriod { get; set; }
[Parameter("Smoothing MA Type", Group = "--- Smoothing MA ---", DefaultValue = MovingAverageType.Exponential)]
public MovingAverageType SmoothMAType { get; set; }
// [Parameter("Use Tick Volume ", Group = "--- Tick Volume ---", DefaultValue = false)]
// public bool UseTicks { get; set; }
// [Parameter("Tick Volume Factor ", Group = "--- Tick Volume ---", DefaultValue = 0.04, Step = 0.01, MinValue = 1E-06)]
// public double TickVolumeFactor { get; set; }
[Parameter("Cut-Off Levels", Group = "--- Level ---", DefaultValue = 0.00025, Step = 1E-05)]
public double Level1 { get; set; }
[Parameter("Secondary Levels", Group = "--- Level ---", DefaultValue = 0.0004, Step = 1E-05)]
public double Level2 { get; set; }
// ---------------
[Output("Histogram UpSlope", PlotType = PlotType.Histogram, LineColor = "Green", Thickness = 5)]
public IndicatorDataSeries HistogramVeryPositive { get; set; }
[Output("Histogram UpDownSlope", PlotType = PlotType.Histogram, LineColor = "DeepSkyBlue", Thickness = 5)]
public IndicatorDataSeries HistogramVeryDownPositive { get; set; }
[Output("Histogram Up", PlotType = PlotType.Histogram, LineColor = "Aquamarine", Thickness = 5)]
public IndicatorDataSeries HistogramPositive { get; set; }
// ---------------
[Output("Histogram DownSlope", PlotType = PlotType.Histogram, LineColor = "DarkRed", Thickness = 5)]
public IndicatorDataSeries HistogramVeryNegative { get; set; }
[Output("Histogram DownUpSlope", PlotType = PlotType.Histogram, LineColor = "Red", Thickness = 5)]
public IndicatorDataSeries HistogramVeryUpNegative { get; set; }
[Output("Histogram Down", PlotType = PlotType.Histogram, LineColor = "Pink", Thickness = 5)]
public IndicatorDataSeries HistogramNegative { get; set; }
// ---------------
[Output("MACD continuous", LineColor = "Gray", PlotType = PlotType.DiscontinuousLine, LineStyle = LineStyle.Solid, Thickness = 2)]
public IndicatorDataSeries MACDcont { get; set; }
[Output("MACD Up", LineColor = "DodgerBlue", PlotType = PlotType.DiscontinuousLine, LineStyle = LineStyle.Solid, Thickness = 2)]
public IndicatorDataSeries MACDup { get; set; }
[Output("MACD Down", LineColor = "Red", PlotType = PlotType.DiscontinuousLine, LineStyle = LineStyle.Solid, Thickness = 2)]
public IndicatorDataSeries MACDdown { get; set; }
[Output("Signal", LineColor = "Yellow", PlotType = PlotType.DiscontinuousLine, LineStyle = LineStyle.Lines, Thickness = 1)]
public IndicatorDataSeries Signal { get; set; }
// ---------------
[Output("levelup", LineColor = "Silver", LineStyle = LineStyle.Dots, PlotType = PlotType.Line, Thickness = 1)]
public IndicatorDataSeries levelup { get; set; }
[Output("leveldown", LineColor = "Silver", LineStyle = LineStyle.Dots, PlotType = PlotType.Line, Thickness = 1)]
public IndicatorDataSeries leveldown { get; set; }
[Output("levelup2", LineColor = "Yellow", LineStyle = LineStyle.Dots, PlotType = PlotType.Line, Thickness = 1)]
public IndicatorDataSeries levelup2 { get; set; }
[Output("leveldown2", LineColor = "Yellow", LineStyle = LineStyle.Dots, PlotType = PlotType.Line, Thickness = 1)]
public IndicatorDataSeries leveldown2 { get; set; }
// ======================================================
private MovingAverage SlowMa;
private MovingAverage SlowMa2;
private MovingAverage FastMa;
private MovingAverage FastMa2;
private MovingAverage SignalMa;
private IndicatorDataSeries MACDAlways;
private IndicatorDataSeries MACDhistogram;
private IndicatorDataSeries MACDcontinuous;
private MovingAverage SmoothMa;
private MovingAverage SmoothedSignalMa;
int lastindex = 0;
// =========================================================
protected override void Initialize()
{
SlowMa = Indicators.MovingAverage(Source, LongCycle, MAType);
FastMa = Indicators.MovingAverage(Source, ShortCycle, MAType);
SlowMa2 = Indicators.MovingAverage(SlowMa.Result, LongCycle, MAType);
FastMa2 = Indicators.MovingAverage(FastMa.Result, ShortCycle, MAType);
MACDAlways = CreateDataSeries();
SignalMa = Indicators.MovingAverage(MACDAlways, Periods, MAType);
MACDhistogram = CreateDataSeries();
MACDcontinuous = CreateDataSeries();
SmoothMa = Indicators.MovingAverage(MACDAlways, SmoothPeriod, SmoothMAType);
SmoothedSignalMa = Indicators.MovingAverage(SignalMa.Result, SmoothPeriod, SmoothMAType);
// =========================================================
}
public override void Calculate(int index)
{
//--------------------------------
if (IsLastBar)
{
if (index != lastindex)
lastindex = index;
else
return;
}
MACDAlways[index] = UseZeroLag ? (FastMa.Result[index] * 2 - FastMa2.Result[index]) - (SlowMa.Result[index] * 2 - SlowMa2.Result[index]) : FastMa.Result[index] - SlowMa.Result[index];
MACDcontinuous[index] = SmoothPeriod > 0 ? SmoothMa.Result[index] : MACDAlways[index];
// ======================================= Drawing ===========================================
Signal[index] = SmoothPeriod > 0 ? SmoothedSignalMa.Result[index] : SignalMa.Result[index];
MACDcont[index] = MACDcontinuous[index];
if (MACDcontinuous.IsRising())
MACDup[index] = SmoothPeriod > 0 ? SmoothMa.Result[index] : MACDAlways[index];
else
MACDdown[index] = SmoothPeriod > 0 ? SmoothMa.Result[index] : MACDAlways[index];
MACDhistogram[index] = MACDcontinuous[index] - Signal[index];
levelup[index] = Level1;
leveldown[index] = -Level1;
levelup2[index] = Level2;
leveldown2[index] = -Level2;
//--------------------------------
//ChartObjects.DrawText("RLabels", " TickVolumeFactor: " + TickVolumeFactor, StaticPosition.TopLeft, Colors.White);
if (MACDhistogram[index] > 0)
{
if (MACDhistogram[index] > Level1)
{
if (MACDhistogram.IsRising())
HistogramVeryPositive[index] = MACDhistogram[index];
else
HistogramVeryDownPositive[index] = MACDhistogram[index];
}
else
HistogramPositive[index] = MACDhistogram[index];
}
else if (MACDhistogram[index] < 0)
{
if (MACDhistogram[index] < -Level1)
{
if (MACDhistogram.IsRising())
HistogramVeryUpNegative[index] = MACDhistogram[index];
else
HistogramVeryNegative[index] = MACDhistogram[index];
}
else
{
HistogramNegative[index] = MACDhistogram[index];
}
}
}
}
}
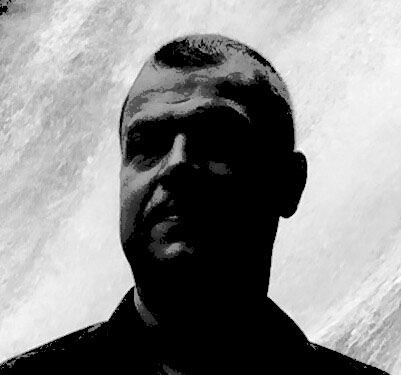
jani
Joined on 05.04.2019
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: MACD Crossover (7).algo
- Rating: 5
- Installs: 5518
Comments
how to get original code of macd i wont to use ema in instead of sma
MACD is now a standard indicator on cTrader. With the above, you can choose which MA to use.