Description
Version uploaded: 2019/11/27
Version updated: 2019/12/11
- Used API Functions Maximum & Minimum https://ctrader.com/api/reference/functions instead of the FOR-loops to get Market Series High & Low. Should result in much fewer calculations by this indicator
I have used Kijun-Sen as a baseline for different trading strategies.
I have simply just combined 3 Kijun-Sen lines and added optional moving average smoothing (MA period 0 will void smoothing).
Original Kijun-Sen line uses 26 periods, I have chosen Fibonacci sequence numbers for the default periods.
Idea is to use Kijun-Sen lines a little bit like normal MAs combined with Ichimoku Cloud idea. Meaning that when lines are close to each other the resistance/support is stronger than when they are far from each other (dense cloud vs. scattered cloud). e.g price crossing together closely coiled lines can be thought of as more valid breakout than if lines are scattered far apart. Price inside lines/cloud => no-mans-land.
Let me know if you find new ideas about how to use this indicator.
What is the Kijun-Sen (Japanese for Reference / Baseline)?
The Kijun-sen is an indicator and important component of the Ichimoku Kinko Hyo method of technical analysis, which is also known as the Ichimoku cloud.
The original Kijun-sen is the midpoint price of the last 26-periods, and therefore an indicator of short- to medium-term price momentum. The indicator aids in assessing the trend, and can also be useful for identifying trading opportunities when combined with the other components of the Ichimoku cloud.
How to Calculate the original Kijun Line (Base Line)
- Find the highest price over the last determined periods.
- Find the lowest price over the last determined periods.
- Combine the high and low, then divide by two.
- Update the calculation after each period ends.
Follow cTrader Telegram group at https://t.me/cTraderCommunity; it's a new community but it will grow fast, plus everyone can talk about cTrader indicators and algorithm without restrictions, though it is not allowed to spam commercial indicators to sell them. There's also a Discord Server now @ https://discord.gg/5GAPMtp
using System;
using cAlgo.API;
using cAlgo.API.Indicators;
namespace cAlgo.Indicators
{
[Indicator(IsOverlay = true, AccessRights = AccessRights.None)]
public class Kijunsen3X : Indicator
{
[Parameter(" Fibonacci:: ", Group = "Fibonacci sequence - !! reference only !! - ", DefaultValue = "8,13,21,34,55,89,144,233,377")]
public string indicatorinfo { get; set; }
[Parameter(" 1) Fast Period", Group = "--- KijunSen Period ---", DefaultValue = 21)]
public int periodFast { get; set; }
[Parameter(" 2) Medium Period", Group = "--- KijunSen Period ---", DefaultValue = 55)]
public int periodMedium { get; set; }
[Parameter(" 3) Slow Period", Group = "--- KijunSen Period ---", DefaultValue = 89)]
public int periodSlow { get; set; }
[Parameter(" MA Period", Group = "--- MA Smoothing Period --- (0 = no smoothing)", DefaultValue = 6, MinValue = 0)]
public int MAPeriod { get; set; }
[Parameter(" Read New Bars Only!", Group = "--- Resource Manager --- (calculate only on new bar!)", DefaultValue = true)]
public bool ReadBars { get; set; }
[Output("Fast Kijunsen ", LineColor = "Red", Thickness = 1, LineStyle = LineStyle.Lines)]
public IndicatorDataSeries KijunSen1 { get; set; }
[Output("Medium Kijunsen", LineColor = "Orange", Thickness = 2, LineStyle = LineStyle.Lines)]
public IndicatorDataSeries KijunSen2 { get; set; }
[Output("Slow Kijunsen 3", LineColor = "Aquamarine", Thickness = 3, LineStyle = LineStyle.Lines)]
public IndicatorDataSeries KijunSen3 { get; set; }
private MovingAverage MA1;
private MovingAverage MA2;
private MovingAverage MA3;
private IndicatorDataSeries Kijun1;
private IndicatorDataSeries Kijun2;
private IndicatorDataSeries Kijun3;
int lastindex = 0;
protected override void Initialize()
{
Kijun1 = CreateDataSeries();
Kijun2 = CreateDataSeries();
Kijun3 = CreateDataSeries();
MA1 = Indicators.MovingAverage(Kijun1, MAPeriod, MovingAverageType.Simple);
MA2 = Indicators.MovingAverage(Kijun2, MAPeriod, MovingAverageType.Simple);
MA3 = Indicators.MovingAverage(Kijun3, MAPeriod, MovingAverageType.Simple);
}
public override void Calculate(int index)
{
if (index < periodSlow)
{
return;
}
if (ReadBars)
{
if (IsLastBar)
{
if (index != lastindex)
lastindex = index;
else
return;
}
}
var FastMaxHigh = MarketSeries.High.Maximum(periodFast);
var FastMinLow = MarketSeries.Low.Minimum(periodFast);
var MediumMaxHigh = MarketSeries.High.Maximum(periodMedium);
var MediumMinLow = MarketSeries.Low.Minimum(periodMedium);
var SlowMaxHigh = MarketSeries.High.Maximum(periodSlow);
var SlowMinLow = MarketSeries.Low.Minimum(periodSlow);
if (MAPeriod > 0)
{
Kijun1[index] = (FastMaxHigh + FastMinLow) / 2;
Kijun2[index] = (MediumMaxHigh + MediumMinLow) / 2;
Kijun3[index] = (SlowMaxHigh + SlowMinLow) / 2;
KijunSen1[index] = MA1.Result[index];
KijunSen2[index] = MA2.Result[index];
KijunSen3[index] = MA3.Result[index];
}
else
{
KijunSen1[index] = (FastMaxHigh + FastMinLow) / 2;
KijunSen2[index] = (MediumMaxHigh + MediumMinLow) / 2;
KijunSen3[index] = (SlowMaxHigh + SlowMinLow) / 2;
}
}
}
}
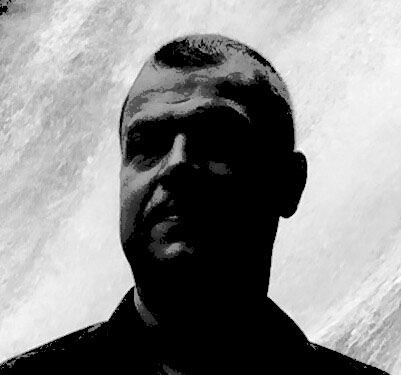
jani
Joined on 05.04.2019
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Kijunsen 3X.algo
- Rating: 0
- Installs: 2341
- Modified: 13/10/2021 09:54