Description
This indicator is a combination of RSI, CCI, Stochastic, DeMarker and Money Flow indicators
Indicator simply calculates the averaged values with adjustable weighting.
Stochastics is calculated from K% only.
You can omit oscillator by inputting weight = 0.
Version updated: 2019/11/06
Normalised CCI values to fit between 0-100
Added "Multiply All Periods" parameter, which will multiply all oscillator periods by the chosen value
Version updated: 2020/2/10
cTrader 3.7 compatible. Added moving average smoothing and signal. If "Average Smooth Period" set to 1 there is no smoothing.
Converted "Multiply All Periods" to double, conversion back to an integer by Convert.ToInt32(Period * MultAll)
Follow cTrader Telegram group at https://t.me/cTraderCommunity; it's a new community but it will grow fast, plus everyone can talk about cTrader indicators and algorithm without restrictions, though it is not allowed to spam commercial indicators to sell them. There's also a Discord Server now @ https://discord.gg/5GAPMtp
/*
==================================================================================
==================================================================================
AverageRSI_CCI_Stoch_DeMarker_MoneyFlow
Copyright © 2020, Fibonacci2011
Developer: Fibonacci2011 // https://ctrader.com/users/profile/23066
Telegram: @Fibonacci2011
==================================================================================
==================================================================================
This indicator is a combination of RSI, CCI, Stochastics, DeMarker and Money Flow indicators
Indicator simply calculates the averaged values with adjustable weighting.
Stochastics is calculated from K% only.
You can omit oscillator by inputting weight = 0.
Version update: 20191106:
Normalised CCI values to fit between 0-100
Added "Multiply All Periods" parameter, which will multiply all oscillator periods by chosen value
Version update: 20200219
cTrader 3.7. compatible. Added moving average smoothing and signal. If "Average Smooth Period" set to 1 there is no smoothing.
Converted "Multiply All Periods" to double, conversion back to an integer by Convert.ToInt32(Period * MultAll)
*/
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
using System;
namespace cAlgo.Indicators
{
[Indicator(IsOverlay = false, TimeZone = TimeZones.UTC, AutoRescale = false, AccessRights = AccessRights.None)]
public class AverageRSI_CCI_Stoch_DeMarker_MoneyFlow : Indicator
{
[Parameter("Source", Group = "--- RSI ---")]
public DataSeries Source { get; set; }
[Parameter("Stochastic Period", Group = "--- Stochastic ---", DefaultValue = 9)]
public int StochPeriod { get; set; }
[Parameter("Stochastic K% Smooth", Group = "--- Stochastic ---", DefaultValue = 3)]
public int KSmooth { get; set; }
[Parameter("RSI Period:", Group = "--- RSI ---", DefaultValue = 14)]
public int Period { get; set; }
[Parameter("CCI Period:", Group = "--- CCI ---", DefaultValue = 20)]
public int CCIPeriod { get; set; }
[Parameter("DeMarker Period", Group = "--- DeMarker ---", DefaultValue = 14)]
public int DEMPeriod { get; set; }
[Parameter("Money Flow Period", Group = "--- Money Flow ---", DefaultValue = 14)]
public int MFPeriod { get; set; }
[Parameter("** Stoch Weight", Group = "--- Stochastic ---", DefaultValue = 1)]
public double StochWeight { get; set; }
[Parameter("** RSI Weight", Group = "--- RSI ---", DefaultValue = 1)]
public double RSIWeight { get; set; }
[Parameter("** CCI Weight", Group = "--- CCI ---", DefaultValue = 1)]
public double CCIWeight { get; set; }
[Parameter("** DeMarker Weight", Group = "--- DeMarker ---", DefaultValue = 1)]
public double DEMWeight { get; set; }
[Parameter("** Money Flow Weight", Group = "--- Money Flow ---", DefaultValue = 1)]
public double MFWeight { get; set; }
[Parameter("Multiply All Periods", Group = "--- All Period Multiplier ---", DefaultValue = 1, MinValue = 1, Step = 0.1)]
public double MultAll { get; set; }
[Parameter("Average Smooth Period", Group = "--- Smoothing & Signal ---", DefaultValue = 5, MinValue = 0)]
public int AllSmoothPeriod { get; set; }
[Parameter("Average Signal Period", Group = "--- Smoothing & Signal ---", DefaultValue = 5, MinValue = 0)]
public int AllSignalPeriod { get; set; }
[Parameter("Average MA Type:", Group = "--- Smoothing & Signal ---", DefaultValue = MovingAverageType.Simple)]
public MovingAverageType MAType { get; set; }
[Parameter("Levels 1", Group = "--- LEVELS ---", DefaultValue = 70)]
public int Level1 { get; set; }
[Output("level 80", LineColor = "Yellow", LineStyle = LineStyle.Dots, PlotType = PlotType.Line, Thickness = 2)]
public IndicatorDataSeries level80 { get; set; }
[Output("level 20", LineColor = "Yellow", LineStyle = LineStyle.Dots, PlotType = PlotType.Line, Thickness = 2)]
public IndicatorDataSeries level20 { get; set; }
[Output("leve 50", LineColor = "PeachPuff", LineStyle = LineStyle.Dots, PlotType = PlotType.Line, Thickness = 2)]
public IndicatorDataSeries level50 { get; set; }
[Output("Average Result", LineColor = "Lime", PlotType = PlotType.Line, Thickness = 2)]
public IndicatorDataSeries AverageResult { get; set; }
[Output("Fast ROC Signal", LineColor = "White", LineStyle = LineStyle.Lines, Thickness = 1)]
public IndicatorDataSeries AverageSignal { get; set; }
private RelativeStrengthIndex rsi;
private CommodityChannelIndex CCI;
private StochasticOscillator Stoch;
private IndicatorDataSeries deMin;
private IndicatorDataSeries deMax;
private MovingAverage deMinMA;
private MovingAverage deMaxMA;
private IndicatorDataSeries DMark;
private MoneyFlowIndex moneyflow;
private IndicatorDataSeries _AverageResult;
private MovingAverage MA;
private MovingAverage MASignal;
public IndicatorDataSeries Result { get; set; }
protected override void Initialize()
{
deMin = CreateDataSeries();
deMax = CreateDataSeries();
deMinMA = Indicators.MovingAverage(deMin, Convert.ToInt32(DEMPeriod * MultAll), MovingAverageType.Simple);
deMaxMA = Indicators.MovingAverage(deMax, Convert.ToInt32(DEMPeriod * MultAll), MovingAverageType.Simple);
DMark = CreateDataSeries();
_AverageResult = CreateDataSeries();
Stoch = Indicators.StochasticOscillator(Convert.ToInt32(StochPeriod * MultAll), KSmooth, 0, MovingAverageType.Simple);
CCI = Indicators.CommodityChannelIndex(Convert.ToInt32(CCIPeriod * MultAll));
// rsi = Indicators.RelativeStrengthIndex(Bars.TypicalPrices, Convert.ToInt32(Period * MultAll));
rsi = Indicators.RelativeStrengthIndex(Source, Convert.ToInt32(Period * MultAll));
moneyflow = Indicators.MoneyFlowIndex(Convert.ToInt32(MFPeriod * MultAll));
MA = Indicators.MovingAverage(_AverageResult, AllSmoothPeriod, MAType);
MASignal = Indicators.MovingAverage(MA.Result, AllSignalPeriod, MAType);
}
public override void Calculate(int index)
{
deMin[index] = Math.Max(Bars.LowPrices[index - 1] - Bars.LowPrices[index], 0);
deMax[index] = Math.Max(Bars.HighPrices[index] - Bars.HighPrices[index - 1], 0);
var min = deMinMA.Result[index];
var max = deMaxMA.Result[index];
DMark[index] = max / (min + max);
if (CCI.Result[index] >= 0)
{
CCI.Result[index] = Math.Min(CCI.Result[index], 200);
}
if (CCI.Result[index] < 0)
{
CCI.Result[index] = Math.Max(CCI.Result[index], -200);
}
CCI.Result[index] = (CCI.Result[index] / 4) + 50;
_AverageResult[index] = ((moneyflow.Result[index] * MFWeight) + (DMark[index] * 100 * DEMWeight) + (rsi.Result[index] * RSIWeight) + (CCI.Result[index] * CCIWeight) + (Stoch.PercentK[index] * StochWeight)) / (MFWeight + DEMWeight + RSIWeight + CCIWeight + StochWeight);
AverageResult[index] = MA.Result[index];
AverageSignal[index] = MASignal.Result[index];
level80[index] = Level1;
level50[index] = 50;
level20[index] = (100 - Level1);
// ChartObjects.DrawText("RLabels", " \n Bars.Count: " +double.NaN + " // " + Bars.Count, StaticPosition.TopLeft, Colors.White);
}
}
}
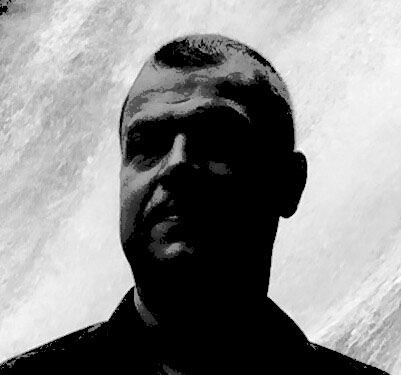
jani
Joined on 05.04.2019
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Average RSI_CCI_Stoch_DeMarker_MoneyFlow.algo
- Rating: 5
- Installs: 2129
Comments
Divergence alert is good idea. Possible ?
Similar to:
https://ctrader.com/algos/indicators/show/1666
There is a kind of myth, a kind of Graal search of the "ultimate" indicator able to read the market whatever the market state or the instrument you're trading. Averaging is a way to have a more robust approach in these different conditions. I suggest adding a signal moving average (Period and MaType) on the average result to visualize, breakout or retracement in addition to the existing OB, OS and pivot levels.
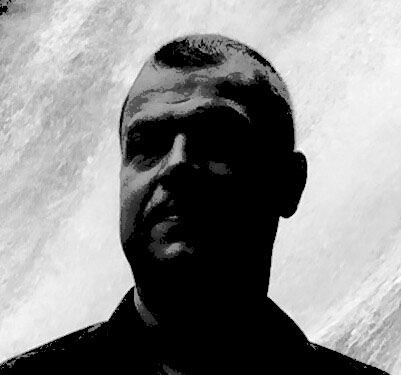
... but the truth is no indicator will help one much, unless one understands the market maker dynamics and games... :)
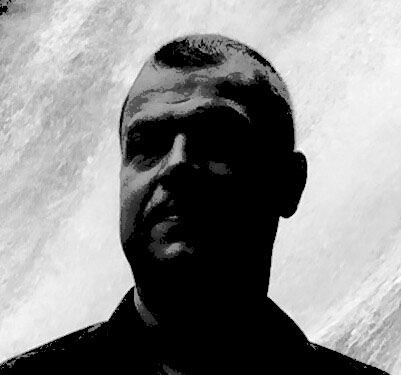
Now with the update and normalized CCI values indicator should be better. (CCi negative values influenced average too much even when capped to -+200 ). Now no need by default to weight other oscillators.
You can also use "Multiply All Periods" to see longer term momentum
Very interesting approach, thanks for sharing. The difficult part now is to find out the right setting for each of the various inputs for a specific TF.
All divergence indicators:
https://ctrader.com/algos/indicators/show/2067