Description
Version Update 2019.12.11
- Corrected small error in average slope calculation /// "for (var i = index - end; i < index - start; i++)" insted of "for (var i = index - end; i =< index - start; i++)"
T3 Dynamics indicator attempts to measure T3 acceleration and velocity within the lookback period.
Histogram: Acceleration
Line: Velocity
--- ! You should first be familiar with Tilson T3 indicator logic and behaviour before you try to use this indicator ! ---
References:
T3 Moving Average [https://ctrader.com/algos/indicators/show/2044. ]
Dynamics calculation logic is the same as in MA Dynamics indicator https://ctrader.com/algos/indicators/show/2008
Follow cTrader Telegram group at https://t.me/cTraderCommunity; it's a new community but it will grow fast, plus everyone can talk about cTrader indicators and algorithm without restrictions, though it is not allowed to spam commercial indicators to sell them. There's also a Discord Server now @ https://discord.gg/5GAPMtp
// ------------------------------------------------------------------------------------
// About T3
// ------------------------------------------------------------------------------------
// T3 MA is a weighted sum of single EMA, double EMA, triple EMA etc
// Original indicator Developed by Tim Tillson
// Color & Min Threshold % features added by @Fibonacci2011 (Telegarm)
// ------------------------------------------------------------------------------------
//
// The Triple Exponential Moving Average (T3) developed by Tim Tillson attempts to offers
// a moving average with better smoothing then traditional exponential moving average.
// It incorporates a smoothing technique which allows it to plot curves more gradual than
// ordinary moving averages and with a smaller lag.
// Its smoothness is derived from the fact that it is a weighted sum of a single EMA,
// is formed, the price action will stay above or below the trend during most of its
// progression and will hardly be touched by any swings. Thus, a confirmed penetration of
// the T3 MA and the lack of a following reversal often indicates the end of a trend.
//
// Added up & down colors and a Min Treshold % factor to filter out T3 “flat” periods.
// Min Treshold % calculates the minimum accepter slope % change between start & stop bars.
//
// ------------------------------------------------------------------------------------
// About T3 Dynamics
// ------------------------------------------------------------------------------------
// T3 Dynamics indicator attemps to measure T3 Acceleration and velocity within the lookback period
//
// References:
//
// T3 Moving Average https://ctrader.com/algos/indicators/show/2044.
// Dynamics calculation logic same as in MA Dynamics https://ctrader.com/algos/indicators/show/2008
//
// ------------------------------------------------------------------------------------
// ------------------------------------------------------------------------------------
using System;
using System.Linq;
using System.Text;
using System.Threading;
using System.Collections.Generic;
using System.Reflection;
using System.Globalization;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
using System.IO;
namespace cAlgo.Indicators
{
[Levels(0)]
[Indicator(IsOverlay = false, ScalePrecision = 1, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class T3Dynamics : Indicator
{
[Parameter()]
public DataSeries Source { get; set; }
[Parameter("T3 Period ", DefaultValue = 14)]
public int Period { get; set; }
[Parameter("T3 Volume Factor ", DefaultValue = 0.7)]
public double Volume_Factor { get; set; }
[Parameter("T3 Min Threshold % ", DefaultValue = 0.0)]
public double minthr { get; set; }
[Parameter("T3 Start Bar ", DefaultValue = 1)]
public int startbar { get; set; }
[Parameter("T3 Stop Bar ", DefaultValue = 2)]
public int stopbar { get; set; }
// ============ VELOCITY & ACCELERATION ==============
[Parameter("Vel. & Acc. LookBack", DefaultValue = 20)]
public int Lookback { get; set; }
[Parameter("Method", DefaultValue = MovingAverageType.Exponential)]
public MovingAverageType MAType { get; set; }
public DataSeries MASource { get; set; }
[Output("Velocity", LineColor = "Cyan")]
public IndicatorDataSeries Vel { get; set; }
[Output("Acceleration", LineColor = "83FFFF01", PlotType = PlotType.Histogram)]
public IndicatorDataSeries Acc { get; set; }
// ================== LEVELS =========================
[Parameter("TLevel 1 ", DefaultValue = 0)]
public int Level1 { get; set; }
[Output("Level 1", Color = Colors.Gold, Thickness = 1, PlotType = PlotType.Points)]
public IndicatorDataSeries level1 { get; set; }
[Output("Level -1", Color = Colors.Gold, Thickness = 1, PlotType = PlotType.Points)]
public IndicatorDataSeries mlevel1 { get; set; }
[Output("ZeroLine", Color = Colors.Honeydew)]
public IndicatorDataSeries zero { get; set; }
// =============================================
private ExponentialMovingAverage ema1;
private ExponentialMovingAverage ema2;
private ExponentialMovingAverage ema3;
private ExponentialMovingAverage ema4;
private ExponentialMovingAverage ema5;
private ExponentialMovingAverage ema6;
private MovingAverage MA;
int lastindex = 0;
protected override void Initialize()
{
MA = Indicators.MovingAverage(Source, Period, MAType);
if (RunningMode == RunningMode.RealTime)
IndicatorArea.DrawHorizontalLine("0", 0, Color.FromHex("78FFFFFF"));
ema1 = Indicators.ExponentialMovingAverage(Source, Period);
ema2 = Indicators.ExponentialMovingAverage(ema1.Result, Period);
ema3 = Indicators.ExponentialMovingAverage(ema2.Result, Period);
ema4 = Indicators.ExponentialMovingAverage(ema3.Result, Period);
ema5 = Indicators.ExponentialMovingAverage(ema4.Result, Period);
ema6 = Indicators.ExponentialMovingAverage(ema5.Result, Period);
}
public override void Calculate(int index)
{
if (IsLastBar)
{
if (index != lastindex)
lastindex = index;
else
return;
}
var val = maketema(index);
var avg = averageslope(startbar, stopbar, index);
Vel[index] = (maketema(index) - maketema(index - Lookback)) / Symbol.PipSize;
Acc[index] = Vel[index] - Vel[index - Lookback];
level1[index] = Level1;
mlevel1[index] = -Level1;
}
//===========================================================================================
// FUNCTIONS CALCULATION
//===========================================================================================
double maketema(int index)
{
double b, b2, b3, c1, c2, c3, c4;
b = Volume_Factor;
b2 = Math.Pow(b, 2);
// Volume Factor Squared
b3 = Math.Pow(b, 3);
// Volume Factor Cubed
c1 = -b3;
c2 = 3 * b2 + 3 * b3;
c3 = -6 * b2 - 3 * b - 3 * b3;
c4 = 1 + 3 * b + b3 + 3 * b2;
var result = c1 * ema6.Result[index] + c2 * ema5.Result[index] + c3 * ema4.Result[index] + c4 * ema3.Result[index];
return result;
}
double averageslope(int start, int end, int index)
{
var sum = 0.0;
var count = 0;
for (var i = index - end; i < index - start; i++)
{
var p0 = maketema(i + 1);
var p1 = maketema(i);
var per = (p0 - p1) / p0 * 100;
sum += per;
count++;
}
return sum / count;
}
}
}
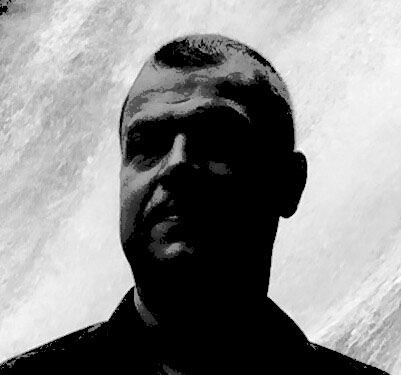
jani
Joined on 05.04.2019
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: T3 Dynamics.algo
- Rating: 0
- Installs: 1600
- Modified: 13/10/2021 09:54
Divergence alert is good idea. Possible ?
Similar to:
https://ctrader.com/algos/indicators/show/1666