Description
A combination of Commodity Channel Index , Bollinger Bands and Moving Averages.
This is my modification of the original Traders Dynamic Index https://ctrader.com/algos/indicators/show/215 (Thanks go to fzlogic !!). Original version uses RSI as the base indicator, I use CCI instead. Two MAs and BB values are calculated from CCI.
CCI is a bit more responsive than RSI, so it is recommended to use higher period values than you would use in RSI. With CCI you get more signals which naturally result also more false signals.
Traditional Strategy:
Buy: The Price line is above the Signal line and above the middle Bollinger Band line.
Sell: The Price line is below the Signal line and below the middle Bollinger Band line.
--------------
Mean Reversion & Reversal Strategies:
Buy: Look for MAs lower-level crossing as in any normal oscillator oversold strategy.
Sell: Look for MAs upper-level crossing as in any normal oscillator overbought strategy.
Extra confirmation with slower MAs diverging from faster and pointing toward trend direction.
--------------
More Advanced Strategy:
Look CCI to be below 0-level and below BB midline also look for weak CCI retrace where the price is not following CCI retrace - sell into this weakness. Use the opposite logic for buy.
Also look for Price and Signal line to refuse BB & 0-level crossing, look to trade with BB-middle trend.
Follow cTrader Telegram group at https://t.me/cTraderCommunity; it's a new community but it will grow fast, plus everyone can talk about cTrader indicators and algorithm without restrictions, though it is not allowed to spam commercial indicators to sell them. There's also a Discord Server now @ https://discord.gg/5GAPMtp
using System;
using cAlgo.API;
using cAlgo.API.Indicators;
namespace cAlgo.Indicators
{
[Levels(-200, -100, 0, 100, 200)]
[Indicator(AccessRights = AccessRights.None)]
public class TradersDynamicIndex_CCI : Indicator
{
private CommodityChannelIndex _CCI;
private MovingAverage _price;
private MovingAverage _signal;
private BollingerBands _bollingerBands;
[Parameter()]
public DataSeries Source { get; set; }
[Parameter("CCI Period", DefaultValue = 20)]
public int CCIPeriod { get; set; }
[Parameter("MA on CCI Price Period", DefaultValue = 2)]
public int PricePeriod { get; set; }
[Parameter("MA on CCI Signal Period", DefaultValue = 7)]
public int SignalPeriod { get; set; }
[Parameter("BB Period", DefaultValue = 34)]
public int Volatility { get; set; }
[Parameter("BB Adjustment", DefaultValue = 3)]
public int adjustment { get; set; }
[Parameter("BB Standard Deviations", DefaultValue = 1.8, Step = 0.1)]
public double StDev { get; set; }
[Parameter("Price Ma Type", DefaultValue = MovingAverageType.Simple)]
public MovingAverageType PriceMaType { get; set; }
[Parameter("Signal Ma Type", DefaultValue = MovingAverageType.Simple)]
public MovingAverageType SignalMaType { get; set; }
[Output("Upper Band", Color = Colors.Aquamarine)]
public IndicatorDataSeries Up { get; set; }
[Output("Lower Band", Color = Colors.Aquamarine)]
public IndicatorDataSeries Down { get; set; }
[Output("Middle Band", Color = Colors.Orange, Thickness = 2)]
public IndicatorDataSeries Middle { get; set; }
[Output("Price", Color = Colors.Green, Thickness = 2)]
public IndicatorDataSeries PriceSeries { get; set; }
[Output("Signal", Color = Colors.Red, Thickness = 2)]
public IndicatorDataSeries SignalSeries { get; set; }
//----------------------------------------------
[Output("Level 1", Color = Colors.Gold, Thickness = 1, PlotType = PlotType.Points)]
public IndicatorDataSeries level1 { get; set; }
[Output("Level -1", Color = Colors.Gold, Thickness = 1, PlotType = PlotType.Points)]
public IndicatorDataSeries mlevel1 { get; set; }
[Output("Level 2", Color = Colors.Gold, Thickness = 1, PlotType = PlotType.Points)]
public IndicatorDataSeries level2 { get; set; }
[Output("Level -2", Color = Colors.Gold, Thickness = 1, PlotType = PlotType.Points)]
public IndicatorDataSeries mlevel2 { get; set; }
[Output("ZeroLine", Color = Colors.Honeydew)]
public IndicatorDataSeries zero { get; set; }
protected override void Initialize()
{
_CCI = Indicators.CommodityChannelIndex(CCIPeriod);
_bollingerBands = Indicators.BollingerBands(_CCI.Result, Volatility, StDev, MovingAverageType.Simple);
_price = Indicators.MovingAverage(_CCI.Result, PricePeriod, PriceMaType);
_signal = Indicators.MovingAverage(_CCI.Result, SignalPeriod, SignalMaType);
}
public override void Calculate(int index)
{
Up[index] = _bollingerBands.Top[index];
Down[index] = _bollingerBands.Bottom[index];
Middle[index] = _bollingerBands.Main[index];
PriceSeries[index] = _price.Result[index];
SignalSeries[index] = _signal.Result[index];
level2[index] = 200;
level1[index] = 100;
zero[index] = 0;
mlevel1[index] = -100;
mlevel2[index] = -200;
}
}
}
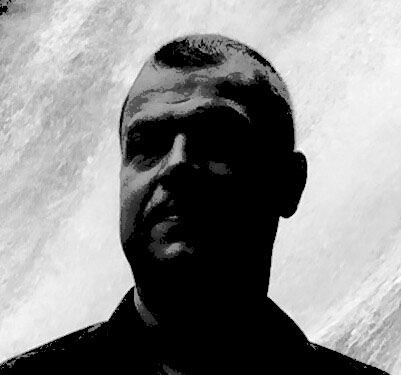
jani
Joined on 05.04.2019
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Traders Dynamic Index (CCI).algo
- Rating: 0
- Installs: 1925