Description
Print OHLC and Tick Volume of selected bars to cBot log. Data can be copied to the clipboard from the log.
Ctrl + Click to print single bar
Ctrl + Mouse Select to print selected bars
using System;
using System.Linq;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class PrintBars : Robot
{
[Parameter("Fill Color", DefaultValue = "2F68D0F7")]
public string FillColor { get; set; }
ChartRectangle DragRectangle;
protected override void OnStart()
{
Chart.MouseDown += Chart_MouseDown;
Chart.MouseUp += Chart_MouseUp;
Chart.MouseMove += Chart_MouseMove;
}
private void Chart_MouseMove(ChartMouseEventArgs obj)
{
UpdateCoordincatesOnChart(obj.TimeValue);
if (DragRectangle == null)
return;
DragRectangle.Time2 = obj.TimeValue;
}
private void Chart_MouseDown(ChartMouseEventArgs obj)
{
if (obj.CtrlKey == false)
return;
Chart.IsScrollingEnabled = false;
DragRectangle = CreateDragRectangle(obj.TimeValue);
}
private void Chart_MouseUp(ChartMouseEventArgs obj)
{
Chart.IsScrollingEnabled = true;
if (DragRectangle != null)
{
PrintBarsToLog(DragRectangle.Time1, DragRectangle.Time2);
Chart.RemoveObject(DragRectangle.Name);
DragRectangle = null;
}
}
private ChartRectangle CreateDragRectangle(DateTime time)
{
var rect = Chart.DrawRectangle("DragRectangle", time, Chart.TopY, time, Chart.BottomY, FillColor);
rect.IsFilled = true;
return rect;
}
private void PrintBarsToLog(DateTime date1, DateTime date2)
{
var firstDate = date1 < date2 ? date1 : date2;
var lastDate = date1 >= date2 ? date1 : date2;
var firstBarIndex = MarketSeries.OpenTime.GetIndexByTime(firstDate);
var lastBarIndex = MarketSeries.OpenTime.GetIndexByTime(lastDate);
Print(" ");
for (int i = firstBarIndex; i <= lastBarIndex; i++)
{
PrintBar(i);
}
}
private void PrintBar(int barIndex)
{
var time = MarketSeries.OpenTime[barIndex].ToString("dd.MM.yy HH:mm:ss.fff");
var open = PriceToString(MarketSeries.Open[barIndex]);
var high = PriceToString(MarketSeries.High[barIndex]);
var low = PriceToString(MarketSeries.Low[barIndex]);
var close = PriceToString(MarketSeries.Close[barIndex]);
var volume = MarketSeries.TickVolume[barIndex].ToString();
var text = string.Format("{0} {1} {2} {3} {4} {5}", time, open, high, low, close, volume);
Print(text);
}
private string PriceToString(double price)
{
var format = "F" + Symbol.Digits.ToString();
return price.ToString(format);
}
private void UpdateCoordincatesOnChart(DateTime time)
{
var text = string.Format(time.ToString("dd.MM.yy HH:mm:ss.fff"));
Chart.DrawStaticText("Coordinates", text, VerticalAlignment.Top, HorizontalAlignment.Left, Chart.ColorSettings.ForegroundColor);
}
}
}
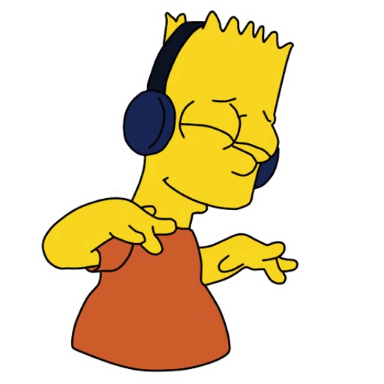
bart1
Joined on 03.08.2017
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Print Bars.algo
- Rating: 0
- Installs: 1566
- Modified: 13/10/2021 09:54
Warning! Running cBots downloaded from this section may lead to financial losses. Use them at your own risk.
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
AR
Wow, What an Outstanding post. I found this too much informatics. It is what I was seeking for. I would like to recommend you that please keep sharing such type of info.If possible, Thanks. Nembutal in vendita
Print Bars are essential tools for businesses and individuals seeking efficient and organized printing solutions. These bars provide a sturdy and reliable platform for holding print media during the printing process. Designed to accommodate various paper sizes, Print Bars ensure precise alignment and prevent paper slippage. Additionally, they can be enhanced with heavy duty packing tape for added stability, ensuring a seamless printing experience and professional-quality output.