Description
Moving Average created by Arnaud Legoux / Dimitrios Kouzis Loukas.
Formula:
Chartshot:
For more info visit: https://web.archive.org/web/20120324082059/http://www.arnaudlegoux.com/wp-content/uploads/2011/03/ALMA-Arnaud-Legoux-Moving-Average.pdf
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class ALMA : Indicator
{
[Parameter()]
public DataSeries Source { get; set; }
[Parameter(DefaultValue = 9)]
public int WINDOW { get; set; }
[Parameter(DefaultValue = 6)]
public int SIGMA { get; set; }
[Parameter(DefaultValue = 0.85)]
public double OFFSET { get; set; }
[Output("Main")]
public IndicatorDataSeries Result { get; set; }
protected override void Initialize()
{
}
public override void Calculate(int index)
{
double eq = 0, Wtd = 0, WtdSum = 0, WtdCum = 0;
for (int i = 0; i < WINDOW - 1; i++)
{
eq = -1 * (Math.Pow(i - OFFSET, 2) / Math.Pow(SIGMA, 2));
Wtd = Math.Exp(eq);
WtdSum = WtdSum + Wtd * Source.Last(i);
WtdCum = WtdCum + Wtd;
Result[index] = WtdSum / WtdCum;
}
}
}
}
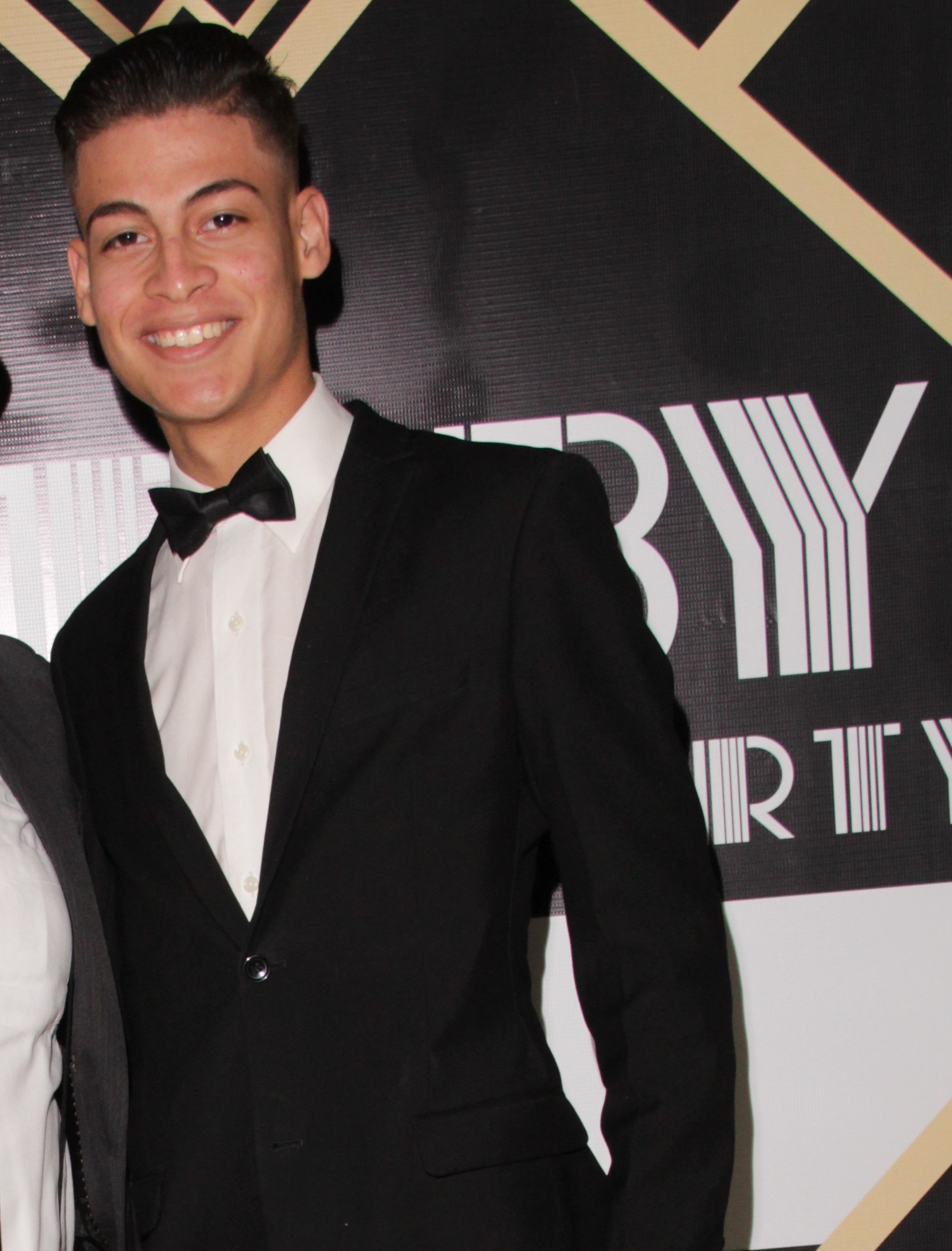
eivaremir
Joined on 08.03.2019
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: ALMA.algo
- Rating: 0
- Installs: 1779
Comments
The indicator is shifted to the left. I compared with tradingview ALMA. Here is my version, it is identical to the one on tradingview. I was writing code for my platform. But as an example it is clear.
_sizeWindws = CreateParameterInt("Size wondows", 9);
_sigma = CreateParameterInt("Sigma", 6);
_offset = CreateParameterDecimal("Offset", 0.85m);
_candlePriceClose = CreateParameterStringCollection("Price close candle", "Close",Entity.CandlePointsArray);
decimal GetAlma(List<Candle> candles, int index)
{
decimal result = 0;
double m = (double)_offset.ValueDecimal * ((double)_sizeWindws.ValueInt - 1);
double s = _sizeWindws.ValueInt / _sigma.ValueInt;
double weight = 0;
for (int i = 0; i < _sizeWindws.ValueInt ; i++)
{
weight = Math.Exp(-((i - m) * (i - m)) / (2 * s * s));
_numerator.Values[index] = _numerator.Values[index] + ((decimal)weight * candles[index - _sizeWindws.ValueInt + 1 + i].GetPoint(_candlePriceClose.ValueString));
_denominator.Values[index] = _denominator.Values[index] + (decimal)weight;
}
result = _numerator.Values[index] / _denominator.Values[index];
return result;
}
Thanks for your contribution, saved my hours
also there is a data type error in my code. change to decimal