Description
Robot places buy and sell STOP orders at specific time. Supports TP, SL, OCO and expiration time.
Parameters:
- News Hour - Hour when news will be published (your local time)
- News Minute - Minute when news will be published (your local time)
- Pips away - The number of pips away from the current market price where the pending buy and sell orders will be placed.
- Take Profit - Take Profit in pips for each order
- Stop Loss - Stop Loss in pips for each order
- Volume - trading volume
- Seconds before - Seconds Before News when robot will place Pending Orders
- Seconds timeout - Seconds After News when Pending Orders will be deleted
- One Cancels Other - If "Yes" then when one order will be filled, another order will be deleted
News Robot PRO with Trailing Stop and Slippage Control:
using System;
using System.Linq;
using System.Text;
using cAlgo.API;
namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class NewsRobot : Robot
{
[Parameter("News Hour", DefaultValue = 14, MinValue = 0, MaxValue = 23)]
public int NewsHour { get; set; }
[Parameter("News Minute", DefaultValue = 30, MinValue = 0, MaxValue = 59)]
public int NewsMinute { get; set; }
[Parameter("Pips away", DefaultValue = 10)]
public int PipsAway { get; set; }
[Parameter("Take Profit", DefaultValue = 50)]
public int TakeProfit { get; set; }
[Parameter("Stop Loss", DefaultValue = 10)]
public int StopLoss { get; set; }
[Parameter("Volume", DefaultValue = 100000, MinValue = 10000)]
public int Volume { get; set; }
[Parameter("Seconds Before", DefaultValue = 10, MinValue = 1)]
public int SecondsBefore { get; set; }
[Parameter("Seconds Timeout", DefaultValue = 10, MinValue = 1)]
public int SecondsTimeout { get; set; }
[Parameter("One Cancels Other")]
public bool Oco { get; set; }
[Parameter("ShowTimeLeftNews", DefaultValue = false)]
public bool ShowTimeLeftToNews { get; set; }
[Parameter("ShowTimeLeftPlaceOrders", DefaultValue = true)]
public bool ShowTimeLeftToPlaceOrders { get; set; }
private bool _ordersCreated;
private DateTime _triggerTimeInServerTimeZone;
private const string Label = "News Robot";
protected override void OnStart()
{
Positions.Opened += OnPositionOpened;
Timer.Start(1);
var triggerTimeInLocalTimeZone = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day, NewsHour, NewsMinute, 0);
if (triggerTimeInLocalTimeZone < DateTime.Now)
triggerTimeInLocalTimeZone = triggerTimeInLocalTimeZone.AddDays(1);
_triggerTimeInServerTimeZone = TimeZoneInfo.ConvertTime(triggerTimeInLocalTimeZone, TimeZoneInfo.Local, TimeZone);
}
protected override void OnTimer()
{
var remainingTime = _triggerTimeInServerTimeZone - Server.Time;
DrawRemainingTime(remainingTime);
if (!_ordersCreated)
{
var sellOrderTargetPrice = Symbol.Bid - PipsAway * Symbol.PipSize;
ChartObjects.DrawHorizontalLine("sell target", sellOrderTargetPrice, Colors.Red, 1, LineStyle.DotsVeryRare);
var buyOrderTargetPrice = Symbol.Ask + PipsAway * Symbol.PipSize;
ChartObjects.DrawHorizontalLine("buy target", buyOrderTargetPrice, Colors.Blue, 1, LineStyle.DotsVeryRare);
if (Server.Time <= _triggerTimeInServerTimeZone && (_triggerTimeInServerTimeZone - Server.Time).TotalSeconds <= SecondsBefore)
{
_ordersCreated = true;
var expirationTime = _triggerTimeInServerTimeZone.AddSeconds(SecondsTimeout);
PlaceStopOrder(TradeType.Sell, Symbol, Volume, sellOrderTargetPrice, Label, StopLoss, TakeProfit, expirationTime);
PlaceStopOrder(TradeType.Buy, Symbol, Volume, buyOrderTargetPrice, Label, StopLoss, TakeProfit, expirationTime);
ChartObjects.RemoveObject("sell target");
ChartObjects.RemoveObject("buy target");
}
}
if (_ordersCreated && !PendingOrders.Any(o => o.Label == Label))
{
Print("Orders expired");
Stop();
}
}
private void DrawRemainingTime(TimeSpan remainingTimeToNews)
{
if (ShowTimeLeftToNews)
{
if (remainingTimeToNews > TimeSpan.Zero)
{
ChartObjects.DrawText("countdown1", "Time left to news: " + FormatTime(remainingTimeToNews), StaticPosition.TopLeft);
}
else
{
ChartObjects.RemoveObject("countdown1");
}
}
if (ShowTimeLeftToPlaceOrders)
{
var remainingTimeToOrders = remainingTimeToNews - TimeSpan.FromSeconds(SecondsBefore);
if (remainingTimeToOrders > TimeSpan.Zero)
{
ChartObjects.DrawText("countdown2", "Time left to place orders: " + FormatTime(remainingTimeToOrders), StaticPosition.TopRight);
}
else
{
ChartObjects.RemoveObject("countdown2");
}
}
}
private static StringBuilder FormatTime(TimeSpan remainingTime)
{
var remainingTimeStr = new StringBuilder();
if (remainingTime.TotalHours >= 1)
remainingTimeStr.Append((int)remainingTime.TotalHours + "h ");
if (remainingTime.TotalMinutes >= 1)
remainingTimeStr.Append(remainingTime.Minutes + "m ");
if (remainingTime.TotalSeconds > 0)
remainingTimeStr.Append(remainingTime.Seconds + "s");
return remainingTimeStr;
}
private void OnPositionOpened(PositionOpenedEventArgs args)
{
var position = args.Position;
if (position.Label == Label && position.SymbolCode == Symbol.Code)
{
if (Oco)
{
foreach (var order in PendingOrders)
{
if (order.Label == Label && order.SymbolCode == Symbol.Code)
{
CancelPendingOrderAsync(order);
}
}
}
Stop();
}
}
}
}
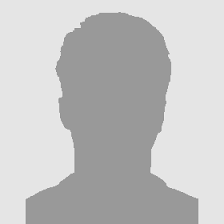
algotrader
Joined on 12.11.2012
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: News Robot.algo
- Rating: 3.67
- Installs: 14985
Comments
New ver ?
hi it is posible to place this order by hand ???not on specific time ?
Can someone help me to add a Slippage control. Like to set maximum negative slippage allowed for a triggered order.
ALGO Turn to me its urgent
EA is not work it starts take order 5 secondes later
it was closed all orders
how can be possible
Also why we are waiting every time allmost 21 hours ?
HELP ME
Hi.I have 2 questions about your EA first one is How can i change UTC for my Republic of Turkey(we are using UTC+3)And seconde question is when i tried to use this code the system gave eror how can i fix ?
Trade.CreateBuyStopOrder(Symbol, Volume, buyOrderTargetPrice, buyOrderTargetPrice - StopLoss * Symbol.PipSize, buyOrderTargetPrice+ TakeProfit * Symbol.PipSize, expirationTime);PlaceStopOrder(TradeType.Buy, Symbol, Volume, buyOrderTargetPrice, Label, StopLoss, TakeProfit, expirationTime);
What can I do to buy the News Robot PRO with Trailing Stop and Slippage Control?
Thanks.
Hello!
Great work!
Can you help me, please , to change this bot to work just for BUY or SELL orders , NOT for BOTH in the same time!?
Thank you for your time and help!!!
Have a nice day and STAY GREEN!!!
Hey Algotrader,
I love this bot it works smooth and perfect.
How much do i need to pay you to offer us the same one with money management and trailing Stop?
nice greeds
Allgotrader,
I love this cbot.
Can you please add this to trailing stop.
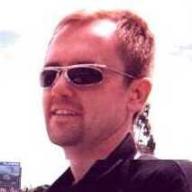
Is it possible, to set both, a move to break even (+pips), and thereafter have a trailing stop after specified number of minutes? the parameters would not be the same for the two. in cTrader you can only set one or the other, but most of the time I have to go back and set a trailing (with different parameters) once break-even has been locked in.
Because of high volatility, I would not want a trailing stop in the first few minutes, so I am not thrown out prematurely. Once things settle down, than let it trail. For example, after 3 minutes, or 5 minutes, commence a trailing stop.
For example: Move to break-even plus 10 after a 50 pip gain, then trail for 10 after the 5 minutes mark. (that would allow for the fast fluctuations while protecting against a loss, and later capture an additional move)
Thank you man!!!!!
Amzing!!
Thank you sir
Hi Im New to Ctrade Can Anyone tell me what should I do to setup this Bot because Im back testing it and showing no results for almost 2 H
thanks
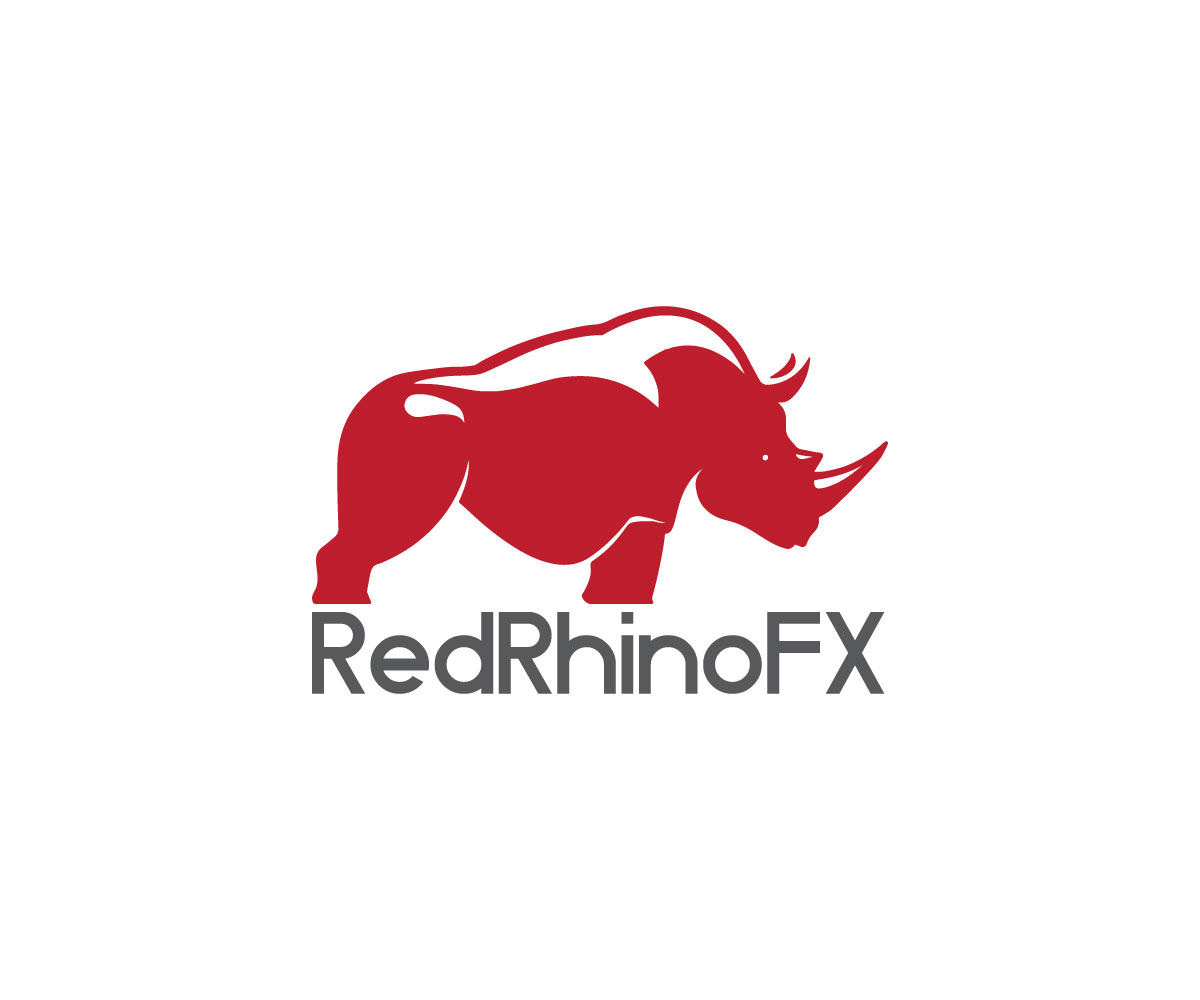
You can use trade copier from ctrader to your mt4, but I don't see the reason to do so because this platform is better for news trading.
@Algotrader.
First, thanks for your job. :)
Then, can I ask you something...What and where should I write in your source code to have a daily repeat of the action?
For example, if I want the same parameters repeat every day at 7pm? Like if I have the same news every day at the same hour?
I know it s sound unusual, but you can imagine that I have my reason. And I will share it if it works.
Thanks in advance.
Installed on two pairs and it executed a few buy and sell orders, none of which were successful. The log message is that they "expired". I use the default settings. Any hints?
by the way man thanks the other time for the help with the bot i when the 3th place on that contest thanks to your bot!!
hi one question again man this robot shoot the orders until it gets a server confirmation or do not?
have a nice day!
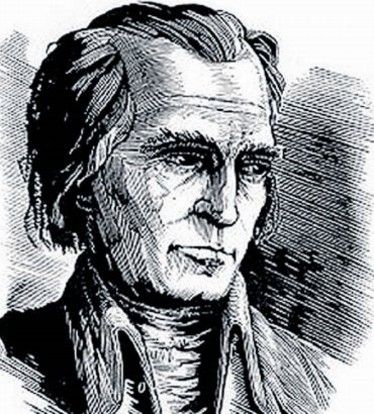
Anybody tell me please if I can use the News Robot on my MT4 platform? If it's possible how can I do that?
Hi there. I´m in Portugal so time zone is UTC+1. It wont work when I place this in the bot. Any ideas on the correct time zone configuration? Kind regards
I need help... The system makes the orders, but then deletes them immediately. What is up? How can I solve this problema?
BigStack,
I am running this on a SUPER micro account, I took 3$ up to 35$, then got a 22 pip slip on stop loss, and went back to 3$. One trade after that massive loss and it is back to 6$. So, I will continue my experiment until I am rich beyond my dreams (lol) or until I implode the account trading as much as I can at 1:500. Either way, I only risk 3$.
Tosabrown,
You are missing the point, they are PENDING orders, waiting for the initial move so you don't have to pick buy or sell. It sets both orders, and whichever is triggered gets the pips.
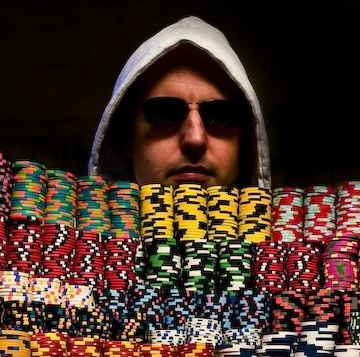
Results??
Hey Guys,
Jompa asked Dec 2012 for results. Would be my #1 question, too.
MyFXBook real money account? Or at least demo? Backtests?
thx
the only lines showing is the pip away lines,
Hello, algotrader, how do I set up this robot, it seems it unable to execute orders during news times
THIS ROBOT IS GARBAGE
What's the purpose of it when it hedges in two directions? You should have separated the buy and sell into separate robots. Or you could have made it into one robot but allowed the buy and sell to be changed by the user. I really don't see the purpose of a robot that initiates a trade in opposite directions by the same number of pips. The least you could have done is make the take profit pips adjustable on each side. I'm surprised no one one this thread brought these things up.
hi great im startimg to really love it but would be grat if you put a trailing stop or breakeven
it's also missing the day to trade field
Could you please add a custom label field to this?
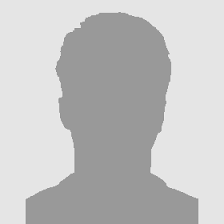
News Robot has been updated. Now you don't need to specify TimeZone. Robot also prints remaining time to chart.
hi to everyone im neewbie on the bot side and 2 years of trading wich i love it and i can tell you how great work you got here this is beautyfull people sharing there passion one cuestion about changing the time zone i know where to put it but i dont know what shut i write im form venezuela and is caracas time i will really thanks if someone cut helpme and else what framework and pair do you people of here find it more profitable thanks again
Sorry, but I don't see it working... Is this normal?
Hey, I tried different ways to write my time zone, but it doesn't seems to work.
*I live in Canada: Eastern Canadian time zone
Thanks a lot...I will let you know the outcome
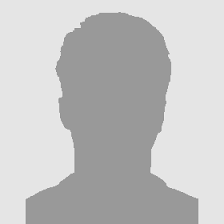
In Australia you need to specify one of these time zones: CenAustraliaStandardTime, EAustraliaStandardTime, WAustraliaStandardTime.
Hi there,
I'm from Australia (Sydney) and tried your robot but didn't trigger the trade. I tried to change the time zone to Australia and Eastern Australia time zone in the parameter but there is an error coming up which say "does not contain the definition for Australia or Eastern Australia".
thanks
Can you please tell me how I can add "Trailing Stop" to this Robot ,Thanks
you can reply to " salau31@yahoo.com " .
Hi algotrader,
Can you add Modify open price function to Robot?
Let's assume we want to open an order with 10pips away from current price, however there is not too much volatility and price just moves less than 10 pips. I think it'll better if we can modify our open price. I mean the open stop order price will constantly be modified and be 10 pips away from current market price, and our order will be filled in case PRICE MOVES EXCEED 10 pips.
Thanks.
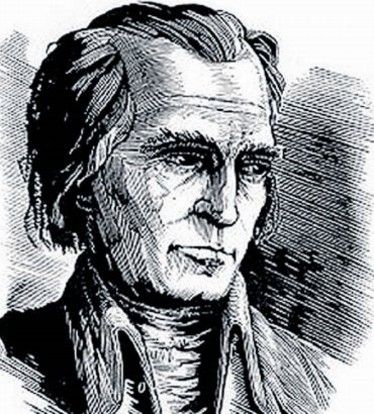
Hi guys,
Let me ask you, why are you proposing the cbots which are not finished. Just take the News Robot and try it in your demo account and you will see that without resolving the slippage problem it's absolutely unuseful and even harmless. I tried it just a few times and one time I got the slippage of 11 pips another time the slippage was 6 pips. Any trader knows that in the news trading a trade opened with more than 2 pips slippage usually (with probability of 90%) went against you.
Guys, please work out the News Robot adding the slippage parameter in or take it out from here. The News Robot as it stands is just seducing traders. Sorry for saying that but it's true.
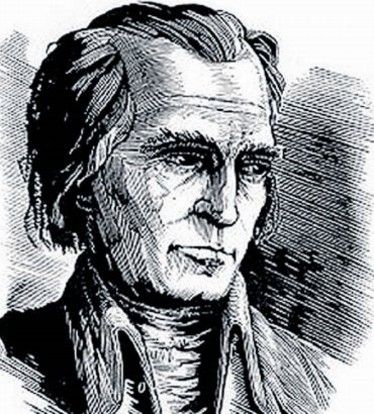
Hi,
If anyone knows programming language #C please let me know how to add a new parameter "Slippage" into the code. Unfortunately, I am not familiar with codes. As I wrote above the ''Slippage" parameter was extremely important in the news trading. Please guys help me. Thanks in advance.
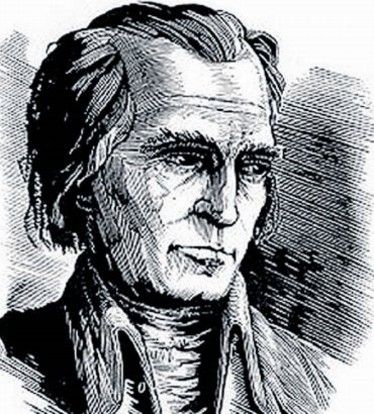
Dear algotrader,
Could you please change the Code adding a new Parameter there? When economical events come a market volatility is quite high thus the Slippage is a very important parameter in the News trading. So, in my opinion the Max. Slippage should be set. I suppose if the Slippage exceeds 3 or 4 pips the order shouldn't be filled. At least it would be fine to have such a possibility.
I used this bot with NZD/USD pair and got the slippage of 11 pips.
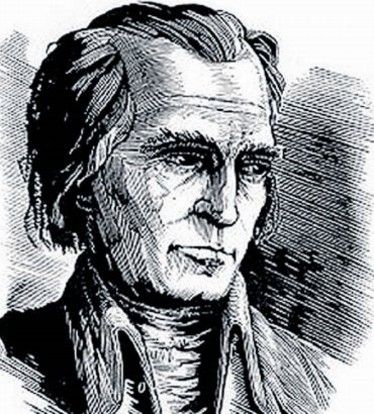
Hi,
I suppose some traders used this News Robot in their real account for quite long time. Please provide any comments on the reliability of this bot. Thanks.
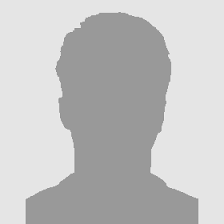
Instead of UTC+1 you need to specify the time zone name. For instance, CentralEuropeStandardTime.
please help, when i add my local time UTC+1 ,This is error message, Error CS0182: An attribute argument must be a constant expression, typeof expression or array creation expression of an attribute parameter type
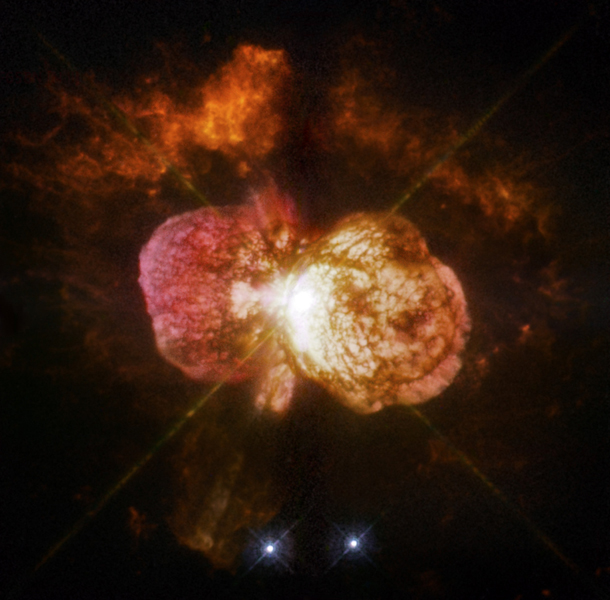
How to get news about ctrader? I use a demo account.
ex.:
- News Hour - Hour when news will be published
- News Minute - Minute when news will be published
etc.
Hi , I really want to say this is a really good EA code.
Thank you.
However, I just transferred from MT4, so I have got 2 questions:
1. If someone entered the WRONG time-to-run this robot, How could they cancel this appointment in cTrader platform?
2. Where is the Trailing Stops (Which means: If the news is NOT QUITE important , the candle - bar will moved-back. So I suppose we change the take-profit to as low as 30 pips, if the candle-bar of GBPUSD dropping-down quickly from 1.5830 to 1.5820 after news released, then come back to 1.5830; but now the position is still open, so what should we do without the Trailing Stops Parameter?
Thanks again for this EA.
I like this EA sooooo much!
Yours,
Yenchy
You can reply me via Email: Yenchy@yenchy.com
Cheers :)
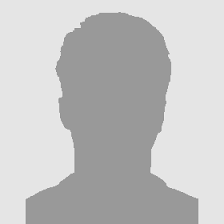
put TimeZone = TimeZones.NewZealandStandardTime
Hi there. Hey we are in New Zealand so time zone is UTC+13. It wont work when I place this in the bot. Any ideas on the correct time zone configuration? Cheers
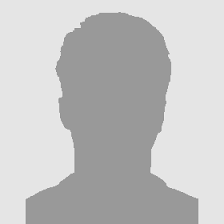
News Robot is updated
Hi, how can I correct the following 4 errors ("ist veraltet" means = "is outdated")
Please can you help me?
Error CS0618: "cAlgo.API.Internals.ITrade.CreateSellStopOrder(cAlgo.API.Internals.Symbol, int, double, double?, double?, System.DateTime?)" ist veraltet: "Use PlaceStopOrder instead"
Error CS0618: "cAlgo.API.Internals.ITrade.CreateBuyStopOrder(cAlgo.API.Internals.Symbol, int, double, double?, double?, System.DateTime?)" ist veraltet: "Use PlaceStopOrder instead"
Error CS0618: "cAlgo.API.Internals.ITrade.DeletePendingOrder(cAlgo.API.PendingOrder)" ist veraltet: "Use CancelPendingOrder instead"
Error CS0618: "cAlgo.API.Internals.ITrade.DeletePendingOrder(cAlgo.API.PendingOrder)" ist veraltet: "Use CancelPendingOrder instead"
all robots are working properly. but news trading robot not working. plz help me ;-(
Beijing time Chinese, should be in "EEuropeStandardTime" fill in what, please enlighten. Thank you.
New Trading API#1
[Spotware]posts: 185since: 23 Sep 2013
In the new version of cAlgo we added a new trading API. The main advantage of this new trading API is the ability to choose between Synchronous and Asynchronous trade methods. The previous API allowed asynchronous methods only and although it is rather flexible, it is difficult to deal with on occasion, because one needs to implement more methods in order to handle some situations.
Question: Is the "News Robot" updated referring to this news?
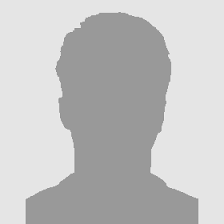
bukk530, MarketDepth.Updated event happens more often than OnTick event
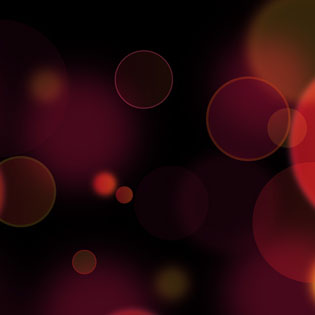
Nice done! Can you please explain me if there is a particular reason why you are using the MarketData Update event instead of the OnTick function? Thanks!
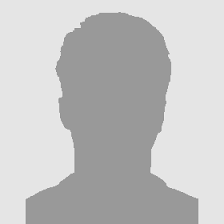
You can specify desired timezone in the code (see image #2)
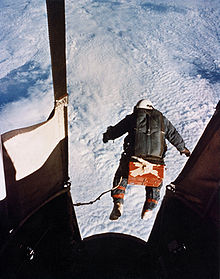
Is time calcualted from Ticks or Server/Local time?
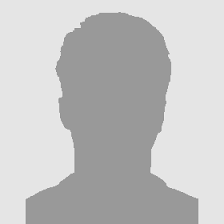
mrlilkkk, I've updated the description. Please look at the first image. Also now it is possible to specify time zone for the robot.
does the take profit set the specified amount of pips away from the market price at the time the orders are placed or the specified amount of pips from the order price?
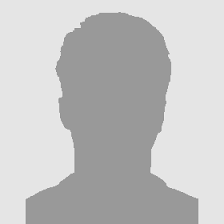
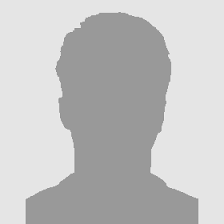
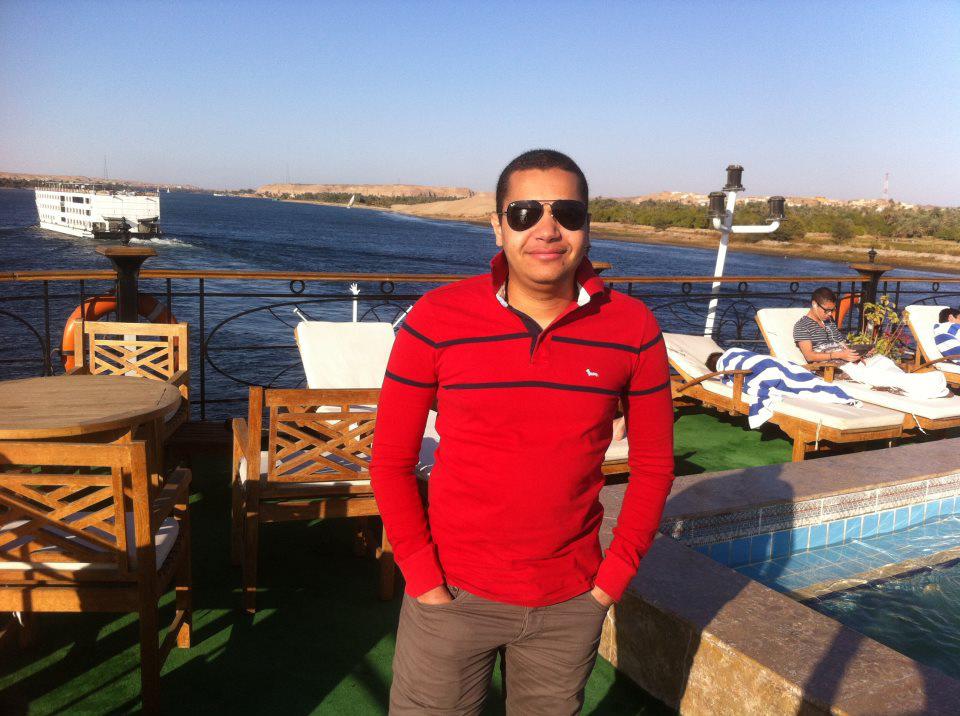
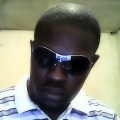
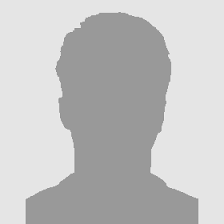
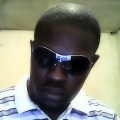
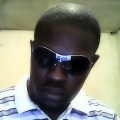
how to change to amount of lot to be open?