Description
Syncronize timeframe between different charts. Support different chart groups.
Instructions:
Add this indicator to all the charts that you want to have the same timeframe. If you want to have more than one group, you should set the Group parameter.
When changing timeframe on one chart, all charts with the same group will be switched to the same timeframe.
Using the existing feature in cTrader to link charts by the symbol, you can setup workspace like this:
Test
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
using System.Collections.Generic;
namespace cAlgo
{
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class TimeframeSync : Indicator
{
[Parameter(DefaultValue = "Sync")]
public string Group { get; set; }
[Parameter("Show group name", DefaultValue = true)]
public bool ShowGroupName { get; set; }
protected override void Initialize()
{
if (Group == null)
return;
CommonTimeframeProvider.InitChart(Group, this);
if (ShowGroupName)
Chart.DrawStaticText("syncGroupText", Group, VerticalAlignment.Top, HorizontalAlignment.Left, Chart.ColorSettings.ForegroundColor);
}
public override void Calculate(int index)
{
}
}
public static class CommonTimeframeProvider
{
private static Dictionary<string, List<Indicator>> _groups = new Dictionary<string, List<Indicator>>();
private static object _lock = new object();
public static void InitChart(string @group, Indicator indicator)
{
lock (_lock)
{
List<Indicator> indicators;
if (!_groups.TryGetValue(@group, out indicators))
{
indicators = new List<Indicator>();
_groups[@group] = indicators;
}
var charts = indicators.ToArray();
var timeframe = indicator.TimeFrame;
foreach (var anotherIndicator in charts)
{
if (timeframe != anotherIndicator.TimeFrame)
{
indicators.Remove(anotherIndicator);
anotherIndicator.BeginInvokeOnMainThread(() => anotherIndicator.Chart.TryChangeTimeFrame(timeframe));
}
}
indicators.Add(indicator);
}
}
}
}
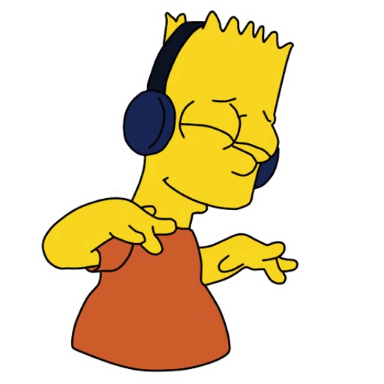
bart1
Joined on 03.08.2017
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: TimeframeSync.algo
- Rating: 5
- Installs: 2187
- Modified: 13/10/2021 09:54
Comments
run this code on ctrader, will work with the latest version :
using System.Collections.Generic;
using cAlgo.API;
using cAlgo.API.Internals;
namespace cAlgo
{
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class TimeframeSync : Indicator
{
private static Dictionary<string, List<Indicator>> _groups = new Dictionary<string, List<Indicator>>();
private static object _lock = new object();
[Parameter(DefaultValue = "Sync")]
public string Group { get; set; }
[Parameter("Show Group Name", DefaultValue = true)]
public bool ShowGroupName { get; set; }
protected override void Initialize()
{
CommonTimeframeProvider.InitChart(Group, this);
if (ShowGroupName)
Chart.DrawStaticText("syncGroupText", Group, VerticalAlignment.Top, HorizontalAlignment.Left, Chart.ColorSettings.ForegroundColor);
}
public override void Calculate(int index)
{
}
public static class CommonTimeframeProvider
{
public static void InitChart(string group, Indicator indicator)
{
lock (_lock)
{
List<Indicator> indicators;
if (!_groups.TryGetValue(group, out indicators))
{
indicators = new List<Indicator>();
_groups[group] = indicators;
}
var charts = indicators.ToArray();
var timeframe = indicator.TimeFrame;
foreach (var anotherIndicator in charts)
{
if (timeframe != anotherIndicator.TimeFrame)
{
indicators.Remove(anotherIndicator);
anotherIndicator.BeginInvokeOnMainThread(() => anotherIndicator.Chart.TryChangeTimeFrame(timeframe));
}
}
indicators.Add(indicator);
}
}
}
}
}
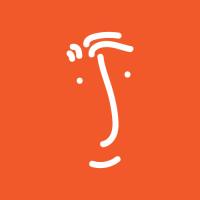
Hi Bart1,
after the latest update to cTrader 4.2 the indicator stopped working properly. I have 12 charts on one screen and if I try to change the TF, it switches it only on 2 charts and the rest 10 charts stays unchanged. I'm using Pepperstone cTrader which was already updated to 4.2 version.
Could you please have a look at it?
Thank you.
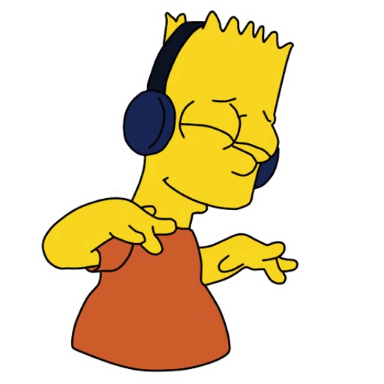
Test
Obrigado! Vai ajudar muito!