Description
Follow my cTrader Telegram group at https://t.me/cTraderCommunity; it's a new community but it will grow fast, plus everyone can talk about cTrader indicators and algorithm without restrictions, though it is not allowed to spam commercial indicators to sell them.
As requested, I made the Absolute Strength Indicator.
Mode = 1, RSI-Like computation
Mode = 2, Stoch-Like computation
For anything, contact me by commenting below or by joining the telegram group linked above
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = false, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class AbsoluteStrength : Indicator
{
[Parameter("Periods", DefaultValue = 14)]
public int per { get; set; }
[Parameter("Smoothing", DefaultValue = 4)]
public int smt { get; set; }
[Parameter("Mode", DefaultValue = 1)]
public int mode { get; set; }
[Output("Bulls", Color = Colors.DarkGreen)]
public IndicatorDataSeries BullsSma { get; set; }
[Output("Bears", Color = Colors.Red)]
public IndicatorDataSeries BearsSma { get; set; }
private IndicatorDataSeries bulls, bears;
private ExponentialMovingAverage avgBulls, avgBears, smtBulls, smtBears;
protected override void Initialize()
{
mode = mode > 2 ? 2 : mode < 1 ? 1 : mode;
bulls = CreateDataSeries();
bears = CreateDataSeries();
avgBulls = Indicators.ExponentialMovingAverage(bulls, per);
avgBears = Indicators.ExponentialMovingAverage(bears, per);
smtBulls = Indicators.ExponentialMovingAverage(avgBulls.Result, smt);
smtBears = Indicators.ExponentialMovingAverage(avgBears.Result, smt);
}
public override void Calculate(int index)
{
bulls[index] = mode == 1 ? 0.5 * (Math.Abs(MarketSeries.Close[index] - MarketSeries.Close[index - 1]) + (MarketSeries.Close[index] - MarketSeries.Close[index - 1])) : MarketSeries.Close[index] - MarketSeries.Low.Minimum(per);
bears[index] = mode == 1 ? 0.5 * (Math.Abs(MarketSeries.Close[index] - MarketSeries.Close[index - 1]) - (MarketSeries.Close[index] - MarketSeries.Close[index - 1])) : MarketSeries.High.Maximum(per) - MarketSeries.Close[index];
BullsSma[index] = smtBulls.Result[index];
BearsSma[index] = smtBears.Result[index];
}
}
}
cysecsbin.01
Joined on 10.11.2018 Blocked
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Absolute Strength.algo
- Rating: 5
- Installs: 2323
Comments
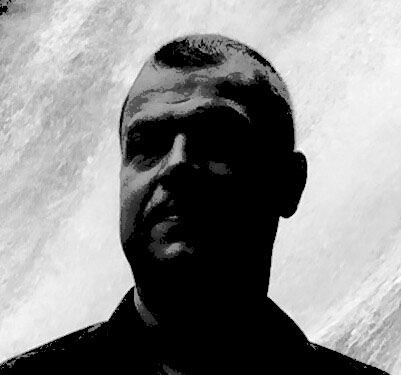
Thanks for sharing.
I noticed that the mode parameter was not working correctly, and Mode =2 was is not showing
All you need to do is just void this line
mode = mode > 2 ? 2 : mode < 1 ? 1 : mode;
as:
// mode = mode > 2 ? 2 : mode < 1 ? 1 : mode;
Now both modes are showing
Below is the C# version..
public class DirectionalVolatility : Indicator
{
public DirectionalVolatility()
{
IndicatorName = "Directional volatility";
PossibleSlots = SlotTypes.OpenFilter | SlotTypes.CloseFilter;
SeparatedChart = true;
IndicatorAuthor = "Footon";
IndicatorVersion = "2.0";
IndicatorDescription = "Footon's indi corner: custom indicators for FSB and FST.";
}
public override void Initialize(SlotTypes slotType)
{
SlotType = slotType;
// The ComboBox parameters
IndParam.ListParam[0].Caption = "Logic";
IndParam.ListParam[0].ItemList = new string[]
{
"The line rises",
"The line falls",
"The line changes its direction upward",
"The line changes its direction downward",
"The line crosses the Signal line upward",
"The line crosses the Signal line downward",
"The line is higher than the Signal line",
"The line is lower than the Signal line"
};
IndParam.ListParam[0].Index = 0;
IndParam.ListParam[0].Text = IndParam.ListParam[0].ItemList[IndParam.ListParam[0].Index];
IndParam.ListParam[0].Enabled = true;
IndParam.ListParam[0].ToolTip = "Logic of application of the indicator.";
IndParam.ListParam[1].Caption = "Smoothing method";
IndParam.ListParam[1].ItemList = Enum.GetNames(typeof(MAMethod));
IndParam.ListParam[1].Index = (int)MAMethod.Simple;
IndParam.ListParam[1].Text = IndParam.ListParam[1].ItemList[IndParam.ListParam[1].Index];
IndParam.ListParam[1].Enabled = true;
IndParam.ListParam[1].ToolTip = "The method used for smoothing.";
IndParam.NumParam[0].Caption = "period1";
IndParam.NumParam[0].Value = 5;
IndParam.NumParam[0].Min = 1;
IndParam.NumParam[0].Max = 200;
IndParam.NumParam[0].Enabled = true;
IndParam.NumParam[0].ToolTip = "The smoothing period ";
IndParam.NumParam[1].Caption = "period2";
IndParam.NumParam[1].Value = 3;
IndParam.NumParam[1].Min = 1;
IndParam.NumParam[1].Max = 200;
IndParam.NumParam[1].Enabled = true;
IndParam.NumParam[1].ToolTip = "The smoothing period ";
// The CheckBox parameters
IndParam.CheckParam[0].Caption = "Use previous bar value";
IndParam.CheckParam[0].Enabled = true;
IndParam.CheckParam[0].ToolTip = "Use the indicator value from the previous bar.";
return;
}
public override void Calculate(IDataSet dataSet)
{
DataSet = dataSet;
// Reading the parameters
MAMethod maMethod = (MAMethod)IndParam.ListParam[1].Index;
int iPeriod = (int)IndParam.NumParam[0].Value;
int iPeriod2 = (int)IndParam.NumParam[1].Value;
int iPrvs = IndParam.CheckParam[0].Checked ? 1 : 0;
// Calculation
int iFirstBar = iPeriod + 3;
double[] range = new double[Bars];
double[] distance = new double[Bars];
double[] adSTDV = new double[Bars];
for (int iBar = 1; iBar < Bars; iBar++)
{
range[iBar] = High[iBar] - Low[iBar];
distance[iBar] = Math.Abs(Open[iBar] - Close[iBar]);
}
for (int iBar = iPeriod; iBar < Bars; iBar++)
{
double dSum = 0;
for (int index = 0; index < iPeriod; index++)
{
double fDelta = (range[iBar - index] - distance[iBar]);
dSum += fDelta * fDelta;
}
adSTDV[iBar] = Math.Sqrt(dSum / iPeriod);
}
double[] signal = MovingAverage(iPeriod2, 0, maMethod, adSTDV);
// Saving the components
Component = new IndicatorComp[4];
Component[0] = new IndicatorComp();
Component[0].CompName = "Main Line";
Component[0].DataType = IndComponentType.IndicatorValue;
Component[0].ChartType = IndChartType.Line;
Component[0].ChartColor = Color.Green;
Component[0].FirstBar = iFirstBar;
Component[0].Value = adSTDV;
Component[1] = new IndicatorComp();
Component[1].CompName = "Signal line";
Component[1].DataType = IndComponentType.IndicatorValue;
Component[1].ChartType = IndChartType.Line;
Component[1].ChartColor = Color.Red;
Component[1].FirstBar = iFirstBar;
Component[1].Value = signal;
Component[2] = new IndicatorComp();
Component[2].ChartType = IndChartType.NoChart;
Component[2].FirstBar = iFirstBar;
Component[2].Value = new double[Bars];
Component[3] = new IndicatorComp();
Component[3].ChartType = IndChartType.NoChart;
Component[3].FirstBar = iFirstBar;
Component[3].Value = new double[Bars];
// Sets the Component's type
if (SlotType == SlotTypes.OpenFilter)
{
Component[2].DataType = IndComponentType.AllowOpenLong;
Component[2].CompName = "Is long entry allowed";
Component[3].DataType = IndComponentType.AllowOpenShort;
Component[3].CompName = "Is short entry allowed";
}
else if (SlotType == SlotTypes.CloseFilter)
{
Component[2].DataType = IndComponentType.ForceCloseLong;
Component[2].CompName = "Close out long position";
Component[3].DataType = IndComponentType.ForceCloseShort;
Component[3].CompName = "Close out short position";
}
switch (IndParam.ListParam[0].Text)
{
case "The line rises":
OscillatorLogic(iFirstBar, iPrvs, adSTDV, 0, 0, ref Component[2], ref Component[3], IndicatorLogic.The_indicator_rises);
break;
case "The line falls":
OscillatorLogic(iFirstBar, iPrvs, adSTDV, 0, 0, ref Component[2], ref Component[3], IndicatorLogic.The_indicator_falls);
break;
case "The line is higher than level":
OscillatorLogic(iFirstBar, iPrvs, adSTDV, 0, 0, ref Component[2], ref Component[3], IndicatorLogic.The_indicator_is_higher_than_the_level_line);
break;
case "The line is lower than level":
OscillatorLogic(iFirstBar, iPrvs, adSTDV, 0, 0, ref Component[2], ref Component[3], IndicatorLogic.The_indicator_is_lower_than_the_level_line);
break;
case "The line crosses the level line upward":
OscillatorLogic(iFirstBar, iPrvs, adSTDV, 0, 0, ref Component[2], ref Component[3], IndicatorLogic.The_indicator_crosses_the_level_line_upward);
break;
case "The line crosses the level line downward":
OscillatorLogic(iFirstBar, iPrvs, adSTDV, 0, 0, ref Component[2], ref Component[3], IndicatorLogic.The_indicator_crosses_the_level_line_downward);
break;
case "The line changes its direction upward":
OscillatorLogic(iFirstBar, iPrvs, adSTDV, 0, 0, ref Component[2], ref Component[3], IndicatorLogic.The_indicator_changes_its_direction_upward);
break;
case "The line changes its direction downward":
OscillatorLogic(iFirstBar, iPrvs, adSTDV, 0, 0, ref Component[2], ref Component[3], IndicatorLogic.The_indicator_changes_its_direction_downward);
break;
case "The line crosses the Signal line upward":
IndicatorCrossesAnotherIndicatorUpwardLogic(iFirstBar, iPrvs, adSTDV, signal, ref Component[2], ref Component[3]);
break;
case "The line crosses the Signal line downward":
IndicatorCrossesAnotherIndicatorDownwardLogic(iFirstBar, iPrvs, adSTDV, signal, ref Component[2], ref Component[3]);
break;
case "The line is higher than the Signal line":
IndicatorIsHigherThanAnotherIndicatorLogic(iFirstBar, iPrvs, adSTDV, signal, ref Component[2], ref Component[3]);
break;
case "The line is lower than the Signal line":
IndicatorIsLowerThanAnotherIndicatorLogic(iFirstBar, iPrvs, adSTDV, signal, ref Component[2], ref Component[3]);
break;
default:
break;
}
return;
}
///
/// Sets the indicator logic description
///
public override void SetDescription()
{
EntryFilterLongDescription = ToString() + "; the line ";
EntryFilterShortDescription = ToString() + "; the line ";
ExitFilterLongDescription = ToString() + "; the line ";
ExitFilterShortDescription = ToString() + "; the line ";
switch (IndParam.ListParam[0].Text)
{
case "The line rises":
EntryFilterLongDescription += "rises";
EntryFilterShortDescription += "falls";
ExitFilterLongDescription += "rises";
ExitFilterShortDescription += "falls";
break;
case "The line falls":
EntryFilterLongDescription += "falls";
EntryFilterShortDescription += "rises";
ExitFilterLongDescription += "falls";
ExitFilterShortDescription += "rises";
break;
case "The line is higher than level":
EntryFilterLongDescription += "is higher than the level line";
EntryFilterShortDescription += "is lower than the level line";
ExitFilterLongDescription += "is higher than the level line";
ExitFilterShortDescription += "is lower than the level line";
break;
case "The line is lower than level":
EntryFilterLongDescription += "is lower than the level line";
EntryFilterShortDescription += "is higher than the level line";
ExitFilterLongDescription += "is lower than the level line";
ExitFilterShortDescription += "is higher than the level line";
break;
case "The line crosses the level line upward":
EntryFilterLongDescription += "crosses the level line upward";
EntryFilterShortDescription += "crosses the level line downward";
ExitFilterLongDescription += "crosses the level line upward";
ExitFilterShortDescription += "crosses the level line downward";
break;
case "The line crosses the level line downward":
EntryFilterLongDescription += "crosses the level line downward";
EntryFilterShortDescription += "crosses the level line upward";
ExitFilterLongDescription += "crosses the level line downward";
ExitFilterShortDescription += "crosses the level line upward";
break;
case "The line changes its direction upward":
EntryFilterLongDescription += "changes its direction upward";
EntryFilterShortDescription += "changes its direction downward";
ExitFilterLongDescription += "changes its direction upward";
ExitFilterShortDescription += "changes its direction downward";
break;
case "The line changes its direction downward":
EntryFilterLongDescription += "changes its direction downward";
EntryFilterShortDescription += "changes its direction upward";
ExitFilterLongDescription += "changes its direction downward";
ExitFilterShortDescription += "changes its direction upward";
break;
case "The line is higher than the Signal line":
EntryFilterLongDescription += "is higher than the Signal line";
EntryFilterShortDescription += "is lower than the Signal line";
ExitFilterLongDescription += "is higher than the Signal line";
ExitFilterShortDescription += "is lower than the Signal line";
break;
case "The line is lower than the Signal line":
EntryFilterLongDescription += "is lower than the Signal line";
EntryFilterShortDescription += "is higher than the Signal line";
ExitFilterLongDescription += "is lower than the Signal line";
ExitFilterShortDescription += "is higher than the Signal line";
break;
case "The line crosses the Signal line upward":
EntryFilterLongDescription += "crosses the Signal line upward";
EntryFilterShortDescription += "crosses the Signal line downward";
ExitFilterLongDescription += "crosses the Signal line upward";
ExitFilterShortDescription += "crosses the Signal line downward";
break;
case "The line crosses the Signal line downward":
EntryFilterLongDescription += "crosses the Signal line downward";
EntryFilterShortDescription += "crosses the Signal line upward";
ExitFilterLongDescription += "crosses the Signal line downward";
ExitFilterShortDescription += "crosses the Signal line upward";
break;
default:
break;
}
return;
}
///
/// Indicator to string
///
public override string ToString()
{
string sString = IndicatorName +
(IndParam.CheckParam[0].Checked ? "* (" : " (") +
IndParam.NumParam[0].ValueToString + ")"; // period
return sString;
}
}
}
Hello,
I found this gem that works like an absolute strength indicator. But the mq4 converter is buggy. Is there a way to turn this indicator into calgo. Thanks in advance.
class Directionalvolatility : public Indicator { public: Directionalvolatility(SlotTypes slotType) { SlotType=slotType; IndicatorName="Directional volatility"; WarningMessage = ""; IsAllowLTF = true; ExecTime = ExecutionTime_DuringTheBar; IsSeparateChart = true; IsDiscreteValues = false; IsDeafultGroupAll = false; } virtual void Calculate(DataSet &dataSet); }; //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ void Directionalvolatility::Calculate(DataSet &dataSet) { Data=GetPointer(dataSet); // Reading the parameters MAMethod maMethod=(MAMethod) ListParam[1].Index; int period=(int) NumParam[0].Value; int period2=(int) NumParam[1].Value; int prvs=CheckParam[0].Checked ? 1 : 0; // Calculation int firstBar=period+3; double range[]; ArrayResize(range,Data.Bars); ArrayInitialize(range, 0); double distance[]; ArrayResize(distance,Data.Bars); ArrayInitialize(distance, 0); double adStdv[]; ArrayResize(adStdv,Data.Bars); ArrayInitialize(adStdv, 0); for(int bar=1; bar<Data.Bars; bar++) { range[bar]=Data.High[bar]-Data.Low[bar]; distance[bar]=MathAbs(Data.Open[bar]-Data.Close[bar]); } for(int bar=period; bar<Data.Bars; bar++) { double sum=0; for(int index=0; index<period; index++) { double delta=(range[bar-index]-distance[bar]); sum+=delta*delta; } adStdv[bar]=MathSqrt(sum/period); } double signal[]; MovingAverage(period2,0,maMethod,adStdv,signal); // Saving the components ArrayResize(Component[0].Value,Data.Bars); Component[0].CompName = "Main Line"; Component[0].DataType = IndComponentType_IndicatorValue; Component[0].FirstBar = firstBar; ArrayCopy(Component[0].Value,adStdv); ArrayResize(Component[1].Value,Data.Bars); Component[1].CompName = "Signal line"; Component[1].DataType = IndComponentType_IndicatorValue; Component[1].FirstBar = firstBar; ArrayCopy(Component[1].Value,signal); ArrayResize(Component[2].Value,Data.Bars); Component[2].FirstBar=firstBar; ArrayResize(Component[3].Value,Data.Bars); Component[3].FirstBar=firstBar; // Sets the Component's type if(SlotType==SlotTypes_OpenFilter) { Component[2].DataType = IndComponentType_AllowOpenLong; Component[2].CompName = "Is long entry allowed"; Component[3].DataType = IndComponentType_AllowOpenShort; Component[3].CompName = "Is short entry allowed"; } else if(SlotType==SlotTypes_CloseFilter) { Component[2].DataType = IndComponentType_ForceCloseLong; Component[2].CompName = "Close out long position"; Component[3].DataType = IndComponentType_ForceCloseShort; Component[3].CompName = "Close out short position"; } if(ListParam[0].Text=="The line rises") OscillatorLogic(firstBar,prvs,adStdv,0,0,Component[2],Component[3],IndicatorLogic_The_indicator_rises); else if(ListParam[0].Text=="The line falls") OscillatorLogic(firstBar,prvs,adStdv,0,0,Component[2],Component[3],IndicatorLogic_The_indicator_falls); else if(ListParam[0].Text=="The line is higher than level") OscillatorLogic(firstBar,prvs,adStdv,0,0,Component[2],Component[3],IndicatorLogic_The_indicator_is_higher_than_the_level_line); else if(ListParam[0].Text=="The line is lower than level") OscillatorLogic(firstBar,prvs,adStdv,0,0,Component[2],Component[3],IndicatorLogic_The_indicator_is_lower_than_the_level_line); else if(ListParam[0].Text=="The line crosses the level line upward") OscillatorLogic(firstBar,prvs,adStdv,0,0,Component[2],Component[3],IndicatorLogic_The_indicator_crosses_the_level_line_upward); else if(ListParam[0].Text=="The line crosses the level line downward") OscillatorLogic(firstBar,prvs,adStdv,0,0,Component[2],Component[3],IndicatorLogic_The_indicator_crosses_the_level_line_downward); else if(ListParam[0].Text=="The line changes its direction upward") OscillatorLogic(firstBar,prvs,adStdv,0,0,Component[2],Component[3],IndicatorLogic_The_indicator_changes_its_direction_upward); else if(ListParam[0].Text=="The line changes its direction downward") OscillatorLogic(firstBar,prvs,adStdv,0,0,Component[2],Component[3],IndicatorLogic_The_indicator_changes_its_direction_downward); else if(ListParam[0].Text=="The line crosses the Signal line upward") IndicatorCrossesAnotherIndicatorUpwardLogic(firstBar,prvs,adStdv,signal,Component[2],Component[3]); else if(ListParam[0].Text=="The line crosses the Signal line downward") IndicatorCrossesAnotherIndicatorDownwardLogic(firstBar,prvs,adStdv,signal,Component[2],Component[3]); else if(ListParam[0].Text=="The line is higher than the Signal line") IndicatorIsHigherThanAnotherIndicatorLogic(firstBar,prvs,adStdv,signal,Component[2],Component[3]); else if(ListParam[0].Text=="The line is lower than the Signal line") IndicatorIsLowerThanAnotherIndicatorLogic(firstBar,prvs,adStdv,signal,Component[2],Component[3]); }
Thank you so much for making this. And btw, I see you wanted to engage with traders using chat. May I suggest that if you are willing to use Discord instead of Telegram. Not to say anything bad about it but Telegram is like old you know.
... you can also replace/add to avoid wrong mode values: