Description
Follow my cTrader Telegram group at https://t.me/cTraderCommunity; it's a new community but it will grow fast, plus everyone can talk about cTrader indicators and algorithm without restrictions, though it is not allowed to spam commercial indicators to sell them.
This is a simple indicator that colors candles according to the selected Moving Average.
For any bug or assistance, comment or ask me directly on the telegram group linked above
Enjoy
using System;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo.Indicators
{
[Indicator(IsOverlay = true, AccessRights = AccessRights.None)]
public class ColorMA : Indicator
{
[Parameter("Periods", DefaultValue = 24)]
public int period { get; set; }
[Parameter("MA Type")]
public MovingAverageType maType { get; set; }
[Output("Main", Color = Colors.Cyan)]
public IndicatorDataSeries Result { get; set; }
private SimpleMovingAverage sma;
private ExponentialMovingAverage ema;
private TimeSeriesMovingAverage ts;
private WeightedMovingAverage wma;
private Vidya vid;
private TriangularMovingAverage trn;
private WellesWilderSmoothing wds;
private int thc;
protected override void Initialize()
{
Thickness();
if (maType == MovingAverageType.Simple)
sma = Indicators.SimpleMovingAverage(MarketSeries.Close, period);
else if (maType == MovingAverageType.Exponential)
ema = Indicators.ExponentialMovingAverage(MarketSeries.Close, period);
else if (maType == MovingAverageType.TimeSeries)
ts = Indicators.TimeSeriesMovingAverage(MarketSeries.Close, period);
else if (maType == MovingAverageType.Weighted)
wma = Indicators.WeightedMovingAverage(MarketSeries.Close, period);
else if (maType == MovingAverageType.VIDYA)
vid = Indicators.Vidya(MarketSeries.Close, period, 0.65);
else if (maType == MovingAverageType.Triangular)
trn = Indicators.TriangularMovingAverage(MarketSeries.Close, period);
else if (maType == MovingAverageType.WilderSmoothing)
wds = Indicators.WellesWilderSmoothing(MarketSeries.Close, period);
Chart.ZoomChanged += OnChartZoomChanged;
}
void OnChartZoomChanged(ChartZoomEventArgs obj)
{
Thickness();
for (int i = 0; i < Chart.BarsTotal; i++)
{
Chart.RemoveObject("smaColorBody" + i);
Calculate(i);
}
}
public override void Calculate(int index)
{
if (maType == MovingAverageType.Simple)
Result[index] = sma.Result[index];
else if (maType == MovingAverageType.Exponential)
Result[index] = ema.Result[index];
else if (maType == MovingAverageType.TimeSeries)
Result[index] = ts.Result[index];
else if (maType == MovingAverageType.Weighted)
Result[index] = wma.Result[index];
else if (maType == MovingAverageType.VIDYA)
Result[index] = vid.Result[index];
else if (maType == MovingAverageType.Triangular)
Result[index] = trn.Result[index];
else if (maType == MovingAverageType.WilderSmoothing)
Result[index] = wds.Result[index];
Chart.DrawTrendLine("smaColorHL" + index, index, MarketSeries.High[index], index, MarketSeries.Low[index], MarketSeries.High[index] < Result[index] ? Color.Red : MarketSeries.Low[index] > Result[index] ? Color.LawnGreen : Color.Orange);
Chart.DrawTrendLine("smaColorBody" + index, index, MarketSeries.Close[index], index, MarketSeries.Open[index], MarketSeries.High[index] < Result[index] ? Color.Red : MarketSeries.Low[index] > Result[index] ? Color.LawnGreen : Color.Orange, thc);
}
private void Thickness()
{
if (Chart.Zoom == 0)
{
thc = 1;
}
if (Chart.Zoom == 1)
{
thc = 2;
}
if (Chart.Zoom == 2)
{
thc = 3;
}
if (Chart.Zoom == 3)
{
thc = 5;
}
if (Chart.Zoom == 4)
{
thc = 11;
}
if (Chart.Zoom == 5)
{
thc = 25;
}
return;
}
}
}
cysecsbin.01
Joined on 10.11.2018 Blocked
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Color MA.algo
- Rating: 5
- Installs: 2413
- Modified: 13/10/2021 09:54
Comments
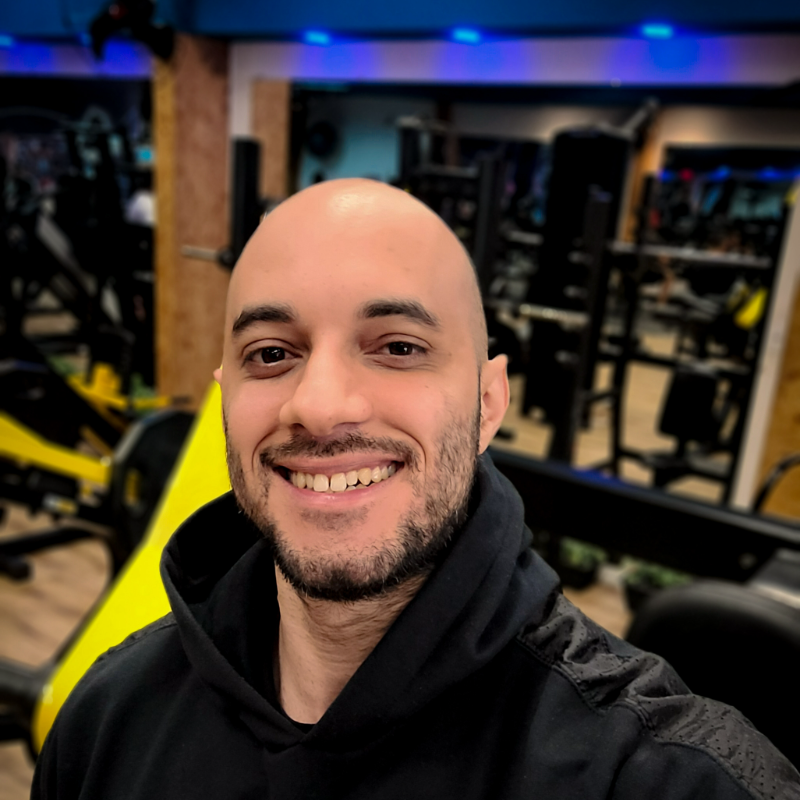
Good morning cysecsbin.01
I really liked and started using your Color MA but is there any way to put the sell bar with no fill but keeping the color condition?
I believe it's gonna help to translate market's behavior using your indicator for better.
Thanks and have a good day.
Nice! Metal trading brokers could use this simple indicator that colors candles according to the selected Moving Average.