Description
Shows: total gain/dd, todays gain/dd, amount at risk, locked profits (SL in Profit), floating pnl, net short/long(0.02 long + 0.02 short = net no position, good when hedging).
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class ExposureInfo : Indicator
{
protected override void Initialize()
{
}
public override void Calculate(int index)
{
double gain = 0;
double gainToday = 0;
double totalGain = 0;
double totalGainToday = 0;
double exposurePips = 0;
double exposureEuro = 0;
double netProfit = 0;
double balanceExposure = 0;
double profitExposure = 0;
double netVolume = 0;
double netPips = 0;
foreach (var p in History)
{
gain += p.NetProfit;
if (p.ClosingTime.DayOfYear == Time.DayOfYear)
{
gainToday += p.NetProfit;
}
}
foreach (var p in Positions)
{
if (this.Symbol.Code == p.SymbolCode && p.TradeType == TradeType.Buy)
{
exposurePips += Math.Round((p.StopLoss.Value - p.EntryPrice) / Symbol.PipSize, 1);
netProfit += p.NetProfit;
netPips += p.Pips;
exposureEuro = Math.Round(exposurePips * (Symbol.PipValue * 100000) * p.Quantity, 2);
balanceExposure = Math.Round(exposureEuro / (Account.Balance - exposureEuro) * 100, 2);
profitExposure = Math.Round(netProfit / (Account.Balance - netProfit) * 100, 2);
netVolume += p.Quantity;
}
if (this.Symbol.Code == p.SymbolCode && p.TradeType == TradeType.Sell)
{
exposurePips += Math.Round((p.EntryPrice - p.StopLoss.Value) / Symbol.PipSize, 1);
netProfit += p.NetProfit;
netPips += p.Pips;
exposureEuro = Math.Round(exposurePips * (Symbol.PipValue * 100000) * p.Quantity, 2);
balanceExposure = Math.Round(exposureEuro / (Account.Balance - exposureEuro) * 100, 2);
profitExposure = Math.Round(netProfit / (Account.Balance - netProfit) * 100, 2);
netVolume -= p.Quantity;
}
}
if (exposurePips > 0)
{
string s = string.Format("\n\nLocked Profit: {0} pips / {1} {2} / {3}%", exposurePips, exposureEuro, Account.Currency, balanceExposure);
ChartObjects.DrawText("Exposure", s, StaticPosition.TopLeft, Colors.Lime);
}
else if (exposurePips < 0)
{
string s = string.Format("\n\nCurrent Risk: {0} pips / {1} {2} / {3}%", exposurePips, exposureEuro, Account.Currency, balanceExposure);
ChartObjects.DrawText("Exposure", s, StaticPosition.TopLeft, Colors.Red);
}
else
{
ChartObjects.DrawText("Exposure", "\n\nN/A", StaticPosition.TopLeft, Colors.Yellow);
}
if (netProfit > 0)
{
string s = string.Format("\n\n\nNet Profit: {0} pips / {1} {2} / {3}%", netPips, netProfit, Account.Currency, profitExposure);
ChartObjects.DrawText("Profit", s, StaticPosition.TopLeft, Colors.Lime);
}
else if (netProfit < 0)
{
string s = string.Format("\n\n\nNet Loss: {0} pips / {1} {2} / {3}%", netPips, netProfit, Account.Currency, profitExposure);
ChartObjects.DrawText("Profit", s, StaticPosition.TopLeft, Colors.Red);
}
else
{
ChartObjects.DrawText("Profit", "\n\n\nN/A", StaticPosition.TopLeft, Colors.Yellow);
}
if (netVolume > 0)
{
string s = string.Format("\n\n\n\nNet Volume: {0}", Math.Round(netVolume, 3));
ChartObjects.DrawText("NetVolume", s, StaticPosition.TopLeft, Colors.Lime);
}
else if (netVolume < 0)
{
string s = string.Format("\n\n\n\nNet Volume: {0}", Math.Round(netVolume, 3));
ChartObjects.DrawText("NetVolume", s, StaticPosition.TopLeft, Colors.Red);
}
else
{
ChartObjects.DrawText("NetVolume", "\n\n\n\nN/A", StaticPosition.TopLeft, Colors.Yellow);
}
totalGain = Math.Round((gain / (Account.Balance - gain)) * 100, 3);
if (gainToday != 0)
{
totalGainToday = Math.Round((gainToday / (Account.Balance - gainToday)) * 100, 3);
}
string text = string.Format("Total gain: {0,0}% \nToday gain: {1,0}%", Math.Round(totalGain, 2), Math.Round(totalGainToday, 2));
ChartObjects.DrawText("Account Text", text, StaticPosition.TopLeft, Colors.White);
}
}
}
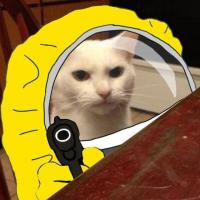
2bnnp
Joined on 12.02.2019
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Symbol_Exposure.algo
- Rating: 5
- Installs: 1803
- Modified: 13/10/2021 09:54
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.