Description
This is a simple little feature I was interested in for a while but never found. It creates a windows form that displays a countdown of the current bar in big letters. It also beeps at the begining/end of a bar. I don't know, I tried using third party timers but they have to be manually synced with the Servers time. This updates every second from the server so its never off.
Using Visual Studio would be better but I got it to work without it. I just updated it so you can set the form tto stay on top. You must add the System.Threading, System.Drawing and System.Windows.Forms libraries. You can use the algo as is or recreate the form in visual studio. You can download a free community version from Mircosoft. From cTrader's cBot menu, select "edit in visual studio". Then add the 2 classes and paste the code into the files that visual studio creates. Build and your all set.
using System;
using System.Drawing;
using cAlgo.API;
using System.Threading;
using System.Windows.Forms;
namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.FullAccess)]
public class myCandleCountDown : Robot
{
[Parameter("Alert On", DefaultValue = true)]
public bool paramAlertOn { get; set; }
[Parameter("Always On Top", DefaultValue = true)]
public bool paramOnTop { get; set; }
private Thread _thread;
private frmCandleCountdown _counter;
const string alertFile = @"C:\Users\user\Documents\cAlgo\Sources\Robots\CountdownTimer.wav";
protected override void OnStart()
{
Timer.Start(1);
_counter = new frmCandleCountdown(this, paramOnTop);
_thread = new Thread(() =>{ _counter.ShowDialog(); });
_thread = new Thread(() => { Application.Run(_counter); });
_thread.SetApartmentState(ApartmentState.STA);
_thread.Start();
}
protected override void OnTimer()
{
int cdMinutes = 14 - Time.Minute % 15;
int cdSeconds = 59 - Time.Second;
_thread = new Thread(() =>{ _counter.UpdateCounter(cdMinutes.ToString("00")+":"+cdSeconds.ToString("00"));});
_thread.SetApartmentState(ApartmentState.STA);
_thread.Start();
if (cdMinutes==0 && cdSeconds==0 && paramAlertOn)
{
System.Media.SoundPlayer player = new System.Media.SoundPlayer(alertFile);
player.Play();
}
}
protected override void OnStop()
{
_thread = new Thread(() => {_counter.Close();});
_thread.SetApartmentState(ApartmentState.STA);
_thread.Start();
}
}
public partial class frmCandleCountdown : Form
{
private const string resourcePath = @"C:\Users\user\Documents\cAlgo\Sources\Robots\myCandleCountDown\myCandleCountDown\Resources\";
myCandleCountDown caller;
bool alwaysOnTop = true;
public frmCandleCountdown(myCandleCountDown _caller, bool _alwaysOnTop)
{
alwaysOnTop = _alwaysOnTop;
caller = _caller;
InitializeComponent();
}
public void UpdateCounter(string _text)
{
lblCounter.Text = _text;
}
private void frmCandleCountdown_FormClosed(object sender, FormClosedEventArgs e)
{
caller.Stop();
}
private void frmCandleCountdown_Load(object sender, EventArgs e)
{
this.Icon = new Icon(resourcePath+"CandleCountdown.ico");
this.TopMost = alwaysOnTop;
}
}
partial class frmCandleCountdown
{
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.IContainer components = null;
/// <summary>
/// Clean up any resources being used.
/// </summary>
/// <param name="disposing">true if managed resources should be disposed; otherwise, false.</param>
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.lblCounter = new System.Windows.Forms.Label();
this.SuspendLayout();
//
// lblCounter
//
this.lblCounter.Dock = System.Windows.Forms.DockStyle.Top;
this.lblCounter.Font = new System.Drawing.Font("Microsoft Sans Serif", 150F, System.Drawing.FontStyle.Bold);
this.lblCounter.Location = new System.Drawing.Point(0, 0);
this.lblCounter.Name = "lblCounter";
this.lblCounter.Size = new System.Drawing.Size(726, 232);
this.lblCounter.TabIndex = 0;
this.lblCounter.Text = "00:00";
this.lblCounter.TextAlign = System.Drawing.ContentAlignment.MiddleCenter;
//
// frmCandleCountdown
//
this.AutoScaleDimensions = new System.Drawing.SizeF(96F, 96F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Dpi;
this.AutoSizeMode = System.Windows.Forms.AutoSizeMode.GrowAndShrink;
this.BackColor = System.Drawing.SystemColors.Window;
this.ClientSize = new System.Drawing.Size(726, 232);
this.Controls.Add(this.lblCounter);
this.Font = new System.Drawing.Font("Microsoft Sans Serif", 15.75F, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((byte)(0)));
this.FormBorderStyle = System.Windows.Forms.FormBorderStyle.Fixed3D;
this.Name = "frmCandleCountdown";
this.StartPosition = System.Windows.Forms.FormStartPosition.CenterParent;
this.Text = "Candle Count Down";
this.TopMost = alwaysOnTop;
this.FormClosed += new System.Windows.Forms.FormClosedEventHandler(this.frmCandleCountdown_FormClosed);
this.Load += new System.EventHandler(this.frmCandleCountdown_Load);
this.ResumeLayout(false);
}
#endregion
private System.Windows.Forms.Label lblCounter;
}
}
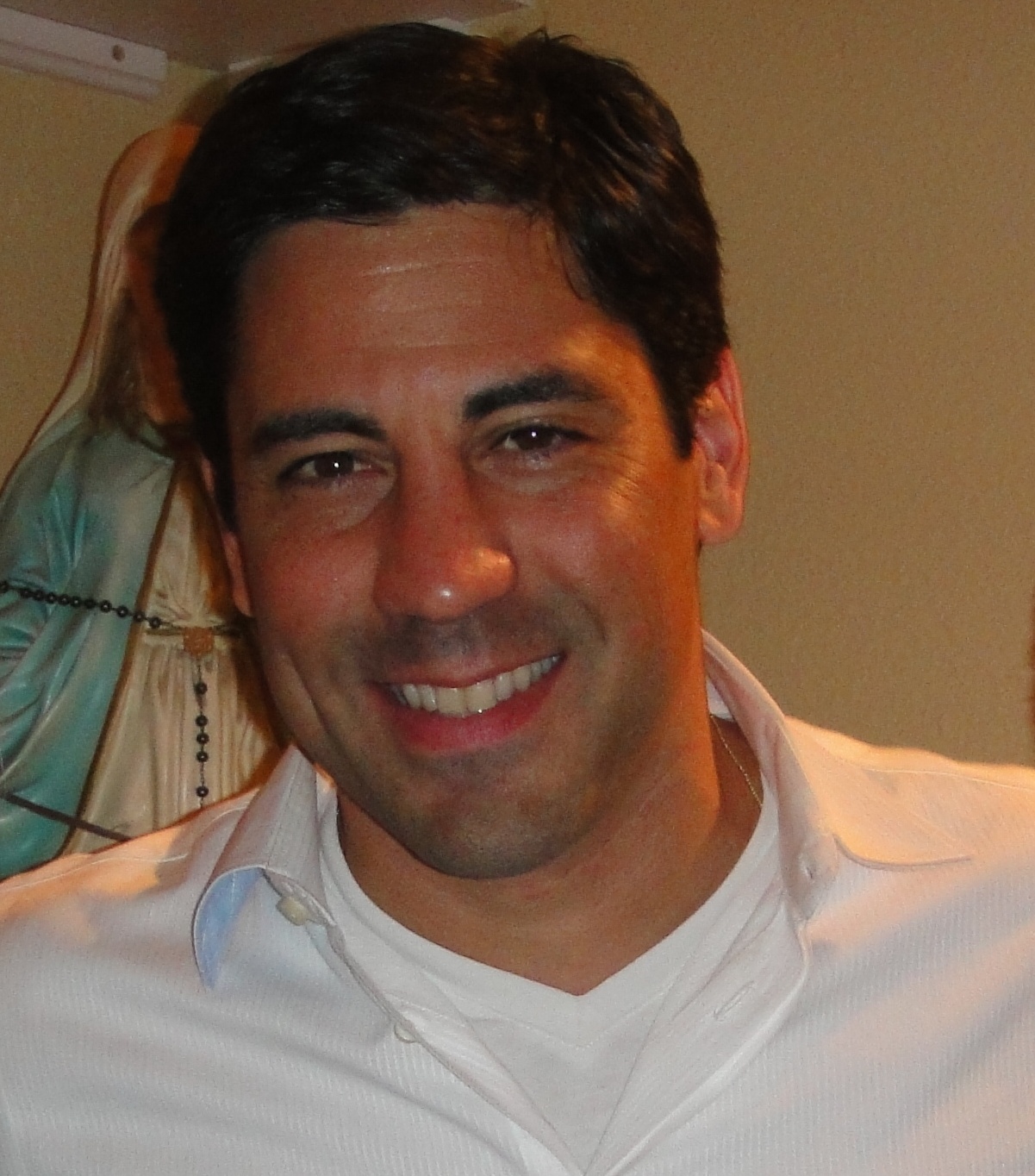
lec0456
Joined on 14.11.2012
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: New cBot (2).algo
- Rating: 5
- Installs: 2203
- Modified: 13/10/2021 09:54
Comments
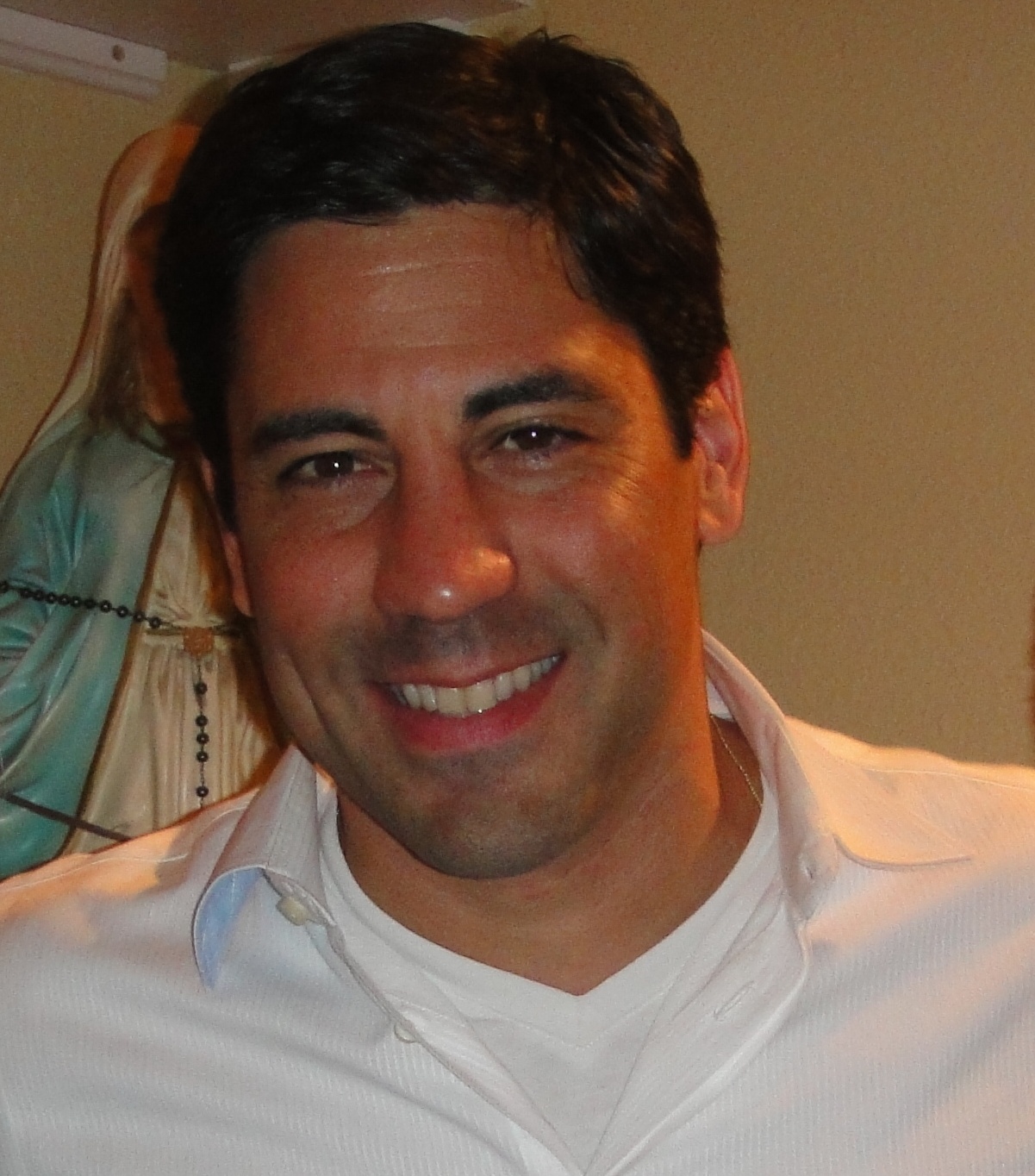
I got the cBot to build with the source code. It seems like that function will not work if you have other visual studio objects in separate files. So, i put them all into one file and it worked.
Looks Great ,
Just make it a lot bigger so it covers the WHOLE chart!
Instead of fixing Ctrader,lets run 100,s of BOTS all falling over themselves,viaing for 100% access rights to your account.Fix the standard time to show actual countdown time .2 H can actually mean 2 hrs 40 mins etc etc.Tradingview is the Benchmark.