Description
The Inverse Fisher Transform version of RSI indicator created by John Ehlers. The purpose of this indicator is to help with determining the turn points on the market and improve timing decisions. Signals are more clear and unequivocal thanks to smoothing function and logarithmic equation (this method can be applied to most of the oscillator-type indicators).
BUY when the indicator crosses over -0.5 or crosses over 0.5 if it has not previously crossed over -0.5.
SELL when the indicator crosses under 0.5 or crosses under -0.5 if it has not previously crossed under 0.5.
using System;
using cAlgo.API;
using cAlgo.API.Indicators;
namespace cAlgo.Indicators
{
[Indicator(IsOverlay = false, AccessRights = AccessRights.None)]
public class InverseFisher : Indicator
{
[Parameter(DefaultValue = 5, MinValue = 4)]
public int Length { get; set; }
[Output("InverseFisher", Color = Colors.OrangeRed, Thickness = 2)]
public IndicatorDataSeries invfisher { get; set; }
private RelativeStrengthIndex rsi;
private IndicatorDataSeries value1;
private IndicatorDataSeries smooth;
protected override void Initialize()
{
rsi = Indicators.RelativeStrengthIndex(MarketSeries.Close, Length);
value1 = CreateDataSeries();
smooth = CreateDataSeries();
}
public override void Calculate(int index)
{
double rsiL = rsi.Result[index];
double rsiH = rsi.Result[index];
for (int i = index - Length + 1; i <= index; i++)
{
if (rsiH < rsi.Result[i])
{
rsiH = rsi.Result[i];
}
if (rsiL > rsi.Result[i])
{
rsiL = rsi.Result[i];
}
}
if (rsiH != rsiL)
{
value1[index] = 0.1 * (rsi.Result[index] - 50);
smooth[index] = (value1[index] + 2 * value1[index - 1] + 2 * value1[index - 2] + value1[index - 3]) / 6;
}
invfisher[index] = (Math.Exp(2 * smooth[index]) - 1) / (Math.Exp(2 * smooth[index]) + 1);
}
}
}
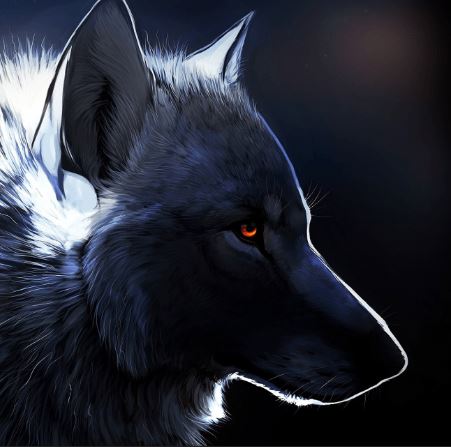
cwik_m
Joined on 26.06.2018
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: InverseFisher.algo
- Rating: 0
- Installs: 2242
- Modified: 13/10/2021 09:54
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.