Description
3x Weighted then Smoothed MACD with multiple price point options
Price Points are
1 = Close
2 = Median
3 = Weighted
4 = Typical
using System;
using cAlgo.API;
using cAlgo.API.Indicators;
using System.Dynamic;
namespace cAlgo.Indicators
{
[Indicator(ScalePrecision = 5, AccessRights = AccessRights.None)]
public class HMACD : Indicator
{
private HullMovingAverage _hmaLong;
private HullMovingAverage _hmaShort;
private HullMovingAverage _hmaSignal;
private HullMovingAverage _hmaLong2;
private HullMovingAverage _hmaShort2;
[Parameter("Long Cycle", DefaultValue = 26)]
public int LongCycle { get; set; }
[Parameter("Short Cycle", DefaultValue = 12)]
public int ShortCycle { get; set; }
[Parameter("Signal Periods", DefaultValue = 9)]
public int SignalPeriods { get; set; }
[Output("Histogram", Color = Colors.Purple, PlotType = PlotType.Histogram)]
public IndicatorDataSeries Histogram { get; set; }
[Output("HMACD", Color = Colors.Green)]
public IndicatorDataSeries Hmacd { get; set; }
[Output("Signal", Color = Colors.Red, LineStyle = LineStyle.Lines)]
public IndicatorDataSeries Signal { get; set; }
[Parameter("Price Point ( 1 = Close Price, 2 = Median Price, 3 = Weighted Close, 4 Typical Price", DefaultValue = 1)]
public int PricePoint { get; set; }
private IndicatorDataSeries _reducedLagHullShort;
private IndicatorDataSeries _reducedLagHullLong;
private TypicalPrice _tp;
private WeightedClose _wc;
private MedianPrice _mp;
protected override void Initialize()
{
_tp = Indicators.TypicalPrice();
_wc = Indicators.WeightedClose();
_mp = Indicators.MedianPrice();
_reducedLagHullShort = CreateDataSeries();
_reducedLagHullLong = CreateDataSeries();
_hmaLong = Indicators.GetIndicator<HullMovingAverage>(ActualPricePoint, LongCycle);
_hmaLong2 = Indicators.GetIndicator<HullMovingAverage>(_hmaLong.Result, LongCycle);
_hmaShort = Indicators.GetIndicator<HullMovingAverage>(ActualPricePoint, ShortCycle);
_hmaShort2 = Indicators.GetIndicator<HullMovingAverage>(_hmaShort.Result, ShortCycle);
_hmaSignal = Indicators.GetIndicator<HullMovingAverage>(Hmacd, SignalPeriods);
}
private DataSeries ActualPricePoint
{
get
{
switch (PricePoint)
{
case 1:
return MarketSeries.Close;
case 2:
return _mp.Result;
case 3:
return _wc.Result;
case 4:
return _tp.Result;
}
return MarketSeries.Close;
}
}
public override void Calculate(int index)
{
_reducedLagHullShort[index] = _hmaShort.Result[index] * 2 - _hmaShort2.Result[index];
_reducedLagHullLong[index] = _hmaLong.Result[index] * 2 - _hmaLong2.Result[index];
Hmacd[index] = _reducedLagHullShort[index] - _reducedLagHullLong[index];
Signal[index] = _hmaSignal.Result[index];
Histogram[index] = Hmacd[index] - Signal[index];
}
}
}
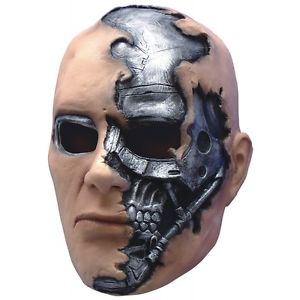
shlbnd.ms
Joined on 10.06.2016
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: HMACD.algo
- Rating: 0
- Installs: 3259
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.