Description
Uhmm.. this initially started as a project for myself to have a break-even level shown on the charts.
(i.e., when market moved against me - but I was sure of the trend, and opened another position - I wanted to know where my new break-even would be, as well as how much I would stand to lose / profit)
Unfortunately, over the 6-8 months of using this myself, different thoughts went into the code - it became slighty bigger than the original breakeven calculator :)
Currently it tracks your daily and weekly P&L against what it thinks you should've made, auto-draws fibs. Monitors and draws fib-based resistance/support levels, on a local and higher time-scales, and on a rare occasion might even give a BUY / SELL suggestion. Additionally, it does re-calculate further, based on the future orders you might have in the same instrument.
It will suggest position sizes, and where positions should be, maximum amount to invest, stop loss points, showing exactly how much you would lose in this or that scenario, and also has some preset take profit suggestions. it also draws the line, where you would stand to lose everything - for the brave risk takers.
The parameters should be left at default at 1st, and are:
- Risk: 1000 - 5000 (think about this as 10%-50% of money you would have left on your account if trade goes bad).
Default 2400 should be left, and increased to may be 4000 for a more conservative day trader
Aahh yes, this indicator works on all time-scales, but would be more beneficial for intraday stuff.
- Maximum balance: 100000 is the default value, ideally this should be x2 your current account value.
But it's probably best to start with x10 your current account value (this is basically the amount you would like to have).
These numbers are used in calculations of suggested take profit and stop loss points.as a side-note, I don't think intra-day trading is good for long term stuff, but if you do stick with indicator suggested stop losses, at least you will have some basic risk management.
you will probably need to adjust the timezone parameter, to your own timezone (line 8)
Anyway, play around; if you like it / find it usefull; give me a shout :)
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
namespace cAlgo.Indicators
{
[Indicator(IsOverlay = true, TimeZone = TimeZones.ArabianStandardTime, AutoRescale = true)]
public class SolidHorizon : Indicator
{
// Risk coefficient, higher number means lower risk, 1000 - high risk, 5000 - low risk (default 2400)
[Parameter("Risk coefficient", DefaultValue = 2400, MinValue = 1000, MaxValue = 5000)]
public int re { get; set; }
// Maximum account value for risk calculation (balance for optimum monthly trading should be half of this)
[Parameter("Maximum account balance", DefaultValue = 100000)]
public int rmax { get; set; }
private int trendperiod = 30 * 12;
private double e, v, ev, p, d, x, g, tp, pg, ttp,
wpt, wwp, wg, od, epmx, epmn, ox, tv, f50, f38,
f61, cp, maxv, ds, range, i, k, op, oe, ov,
oev, og, max, min, pips, opmx, opmn, ddcoef, rp;
private bool mainup, localup, immdup, bullrange, bearrange, bullsup, bearsup, bullres, bearres, brokesup,
brokeres;
private ExponentialMovingAverage ema50, ema200;
Colors tc, wc, rc;
Colors pColor = Colors.SlateBlue;
protected override void Initialize()
{
Positions.Closed += PositionClosed;
epmx = 0;
epmn = 100000;
ema50 = Indicators.ExponentialMovingAverage(MarketSeries.Close, 50 * 12);
ema200 = Indicators.ExponentialMovingAverage(MarketSeries.Close, 200 * 12);
}
private void PositionClosed(PositionClosedEventArgs args)
{
var pos = args.Position;
string pl = "position" + pos.EntryTime;
string pl2 = pl + "2";
ChartObjects.RemoveObject(pl);
ChartObjects.RemoveObject(pl2);
epmx = 0;
epmn = 100000;
}
public override void Calculate(int index)
{
e = 0;
v = 0;
oe = 0;
ov = 0;
tv = 0;
ddcoef = (100 / ((rmax - Account.Equity) / (re * 7.5)));
if (ddcoef < 1)
ddcoef = 1;
//ds = (int)(Account.Equity * 8 / 5000) * 5000;
ds = (int)(((rmax - Account.Equity) / re * Account.Equity / 5) / 5000) * 5000;
if (ds < 10000)
ds = 10000;
maxv = ds * 5;
max = MarketSeries.High.Maximum(MarketSeries.High.Count / 2);
min = MarketSeries.Low.Minimum(MarketSeries.Low.Count / 2);
range = max - min;
pips = range / 4;
f50 = (max - min) * 0.5;
f38 = (max - min) * 0.382;
f61 = (max - min) * 0.618;
ChartObjects.DrawHorizontalLine("mx", max, Colors.Orange, 1, LineStyle.Lines);
ChartObjects.DrawHorizontalLine("mn", min, Colors.Orange, 1, LineStyle.Lines);
ChartObjects.DrawHorizontalLine("f50", min + f50, Colors.OrangeRed, 1, LineStyle.DotsRare);
ChartObjects.DrawHorizontalLine("f38", min + f38, Colors.DarkOrange, 1, LineStyle.DotsVeryRare);
ChartObjects.DrawHorizontalLine("f61", min + f61, Colors.DarkOrange, 1, LineStyle.DotsVeryRare);
localtrend(index);
foreach (var position in Positions)
{
if (position.SymbolCode == Symbol.Code)
{
ev = position.Volume;
tv += ev;
if (position.TradeType == TradeType.Sell)
ev = ev * -1;
e = e + position.EntryPrice * ev;
v = v + ev;
if (position.EntryPrice >= epmx)
{
epmx = position.EntryPrice;
ChartObjects.RemoveObject("pmx");
}
if (position.EntryPrice <= epmn)
{
epmn = position.EntryPrice;
ChartObjects.RemoveObject("pmn");
}
}
}
p = e / v;
if (v == 0)
{
p = Symbol.Bid;
//v = (int)(Account.Equity * 8 / 5000) * 5000;
v = (int)ds;
}
if (Positions.Count > 0)
{
ov = v;
oe = e;
}
tp = 0;
wpt = 0;
wwp = 0;
ttp = Account.Equity / 25;
wwp = ttp * 3;
foreach (var ht in History)
{
if (ht.SymbolCode == Symbol.Code && ht.ClosingTime.Date == DateTime.Now.Date)
tp = tp + ht.NetProfit;
if (ht.SymbolCode == Symbol.Code && ht.ClosingTime.Date > DateTime.Now.AddDays(-7))
wpt = wpt + ht.NetProfit;
}
opmx = epmx;
opmn = epmn;
foreach (var order in PendingOrders)
{
if (order.SymbolCode == Symbol.Code)
{
oev = order.Volume;
if (order.TradeType == TradeType.Sell)
oev = oev * -1;
oe = oe + order.TargetPrice * oev;
ov = ov + oev;
if (order.TargetPrice >= opmx)
opmx = order.TargetPrice;
if (order.TargetPrice <= opmn)
opmn = order.TargetPrice;
}
}
op = oe / ov;
pg = ttp - tp;
wg = wwp - wpt;
//d = (Account.Equity / 20 * Symbol.PipSize / Symbol.PipValue) / v;
d = (Account.Equity / ddcoef * Symbol.PipSize / Symbol.PipValue) / v;
x = (Account.FreeMargin * Symbol.PipSize / Symbol.PipValue) / v;
g = (pg * Symbol.PipSize / Symbol.PipValue) / v;
//cp = g * (tv / (Account.Equity * 5 * 8));
cp = g * (tv / (ds * 5));
//od = (Account.Equity / 20 * Symbol.PipSize / Symbol.PipValue) / ov;
od = (Account.Equity / ddcoef * Symbol.PipSize / Symbol.PipValue) / ov;
ox = ((Account.FreeMargin + Account.UnrealizedNetProfit) * Symbol.PipSize / Symbol.PipValue) / ov;
og = (pg * Symbol.PipSize / Symbol.PipValue) / ov;
string be = "" + p.ToString("0.00000") + " (" + v / 1000 + ")";
string bg = "" + v;
string bo = "" + op.ToString("0.00000") + " (" + ov / 1000 + ")";
string rp = "" + (int)(pg - Account.UnrealizedNetProfit) * -1;
//string de = "" + (int)(Account.Equity / 20) * -1;
//string tl3 = "" + (int)(Account.Equity / 20 * 2) * -1;
//string tl4 = "" + (int)(Account.Equity / 20 * 3) * -1;
//string tl5 = "" + (int)(Account.Equity / 20 * 4) * -1;
string de = "" + (int)(Account.Equity / ddcoef) * -1;
string tl3 = "" + (int)(Account.Equity / ddcoef * 2) * -1;
string tl4 = "" + (int)(Account.Equity / ddcoef * 3) * -1;
string tl5 = "" + (int)(Account.Equity / ddcoef * 4) * -1;
string dp = "" + (int)pg;
string wp = "" + (int)(wg * -1 + Account.UnrealizedNetProfit);
double up = Account.UnrealizedNetProfit;
double ae = Account.Equity / 1000;
string ps = "" + up.ToString("0") + " (" + ae.ToString("0.0") + "k )";
string vi = ps + "\n" + tv / 1000 + " / " + maxv / 1000 + " (" + (int)ds / 1000 + ")";
if (pg < 0)
tc = Colors.DarkRed;
else
tc = Colors.DarkSeaGreen;
if (wg - Account.UnrealizedNetProfit > 0)
wc = Colors.DarkRed;
else
wc = Colors.DarkSeaGreen;
if (pg - Account.UnrealizedNetProfit > 0)
rc = Colors.DarkRed;
else
rc = Colors.DarkSeaGreen;
if ((Account.UnrealizedNetProfit / pg) > (tv / (ds * 5)))
{
ChartObjects.DrawText("gtc", "Good to Close: " + Account.UnrealizedNetProfit.ToString("0"), StaticPosition.BottomLeft, Colors.LightYellow);
ChartObjects.RemoveObject("vi");
}
else
{
ChartObjects.DrawText("vi", vi, StaticPosition.BottomLeft, Colors.LightYellow);
ChartObjects.RemoveObject("gtc");
}
ChartObjects.DrawText("p", rp, StaticPosition.TopRight, rc);
ChartObjects.DrawText("wpi", wp, StaticPosition.BottomRight, wc);
ChartObjects.DrawLine("agg", index - 5, p, index + 10, p, Colors.Blue);
ChartObjects.DrawText("aggn", be, index + 3, p, VerticalAlignment.Top, HorizontalAlignment.Right, Colors.NavajoWhite);
if (PendingOrders.Count > 0)
{
ChartObjects.DrawLine("agg o", index - 5, op, index + 10, op, Colors.MediumSeaGreen);
ChartObjects.DrawLine("agg ou", index - 1, op + og, index + 10, op + og, Colors.MediumSeaGreen);
ChartObjects.DrawText("aggn o", bo, index + 3, op, VerticalAlignment.Top, HorizontalAlignment.Right, Colors.MediumSeaGreen);
ChartObjects.DrawLine("ogg d", index - 5, op - od, index + 10, op - od, Colors.MediumSeaGreen);
ChartObjects.DrawLine("ot d", index - 5, op - od, index + 10, op - od, Colors.SeaGreen);
ChartObjects.DrawLine("ot25 d", index - 5, op - od * 2, index + 10, op - od * 2, Colors.SeaGreen, 1, LineStyle.DotsVeryRare);
ChartObjects.DrawLine("ot30 d", index - 5, op - od * 3, index + 10, op - od * 3, Colors.SeaGreen, 1, LineStyle.DotsVeryRare);
ChartObjects.DrawLine("ot50 d", index - 5, op - od * 4, index + 10, op - od * 4, Colors.SeaGreen, 1, LineStyle.DotsRare);
ChartObjects.DrawText("ottd5", tl5, index + 6, op - od * 4, VerticalAlignment.Top, HorizontalAlignment.Right, Colors.DarkRed);
ChartObjects.DrawLine("ox d", index - 5, op - ox, index + 10, op - ox, Colors.IndianRed);
if (ov > 0)
{
ChartObjects.DrawLine("omn", index - 90, opmx - range, index - 60, opmx - range, Colors.ForestGreen, 1, LineStyle.DotsRare);
for (i = 1; (ov + ds * i) <= maxv; i++)
{
string ntl = "OPos" + i;
string ntl2 = ntl + "2";
ChartObjects.DrawLine(ntl, index - 90, opmn - pips * i, index - 60, opmn - pips * i, Colors.ForestGreen, 1, LineStyle.DotsVeryRare);
ChartObjects.DrawLine(ntl2, index - 70, opmn - pips * i + pips / 2, index - 60, opmn - pips * i + pips / 2, Colors.ForestGreen, 1, LineStyle.DotsVeryRare);
}
}
if (ov < 0)
{
ChartObjects.DrawLine("omx", index - 90, opmn + range, index - 60, opmn + range, Colors.ForestGreen, 1, LineStyle.DotsRare);
for (i = 1; (ov - ds * i) >= maxv * -1; i++)
{
string ntl = "OPos" + i;
string ntl2 = ntl + "2";
ChartObjects.DrawLine(ntl, index - 90, opmx + pips * i, index - 60, opmx + pips * i, Colors.ForestGreen, 1, LineStyle.DotsVeryRare);
ChartObjects.DrawLine(ntl2, index - 70, opmx + pips * i - pips / 2, index - 60, opmx + pips * i - pips / 2, Colors.ForestGreen, 1, LineStyle.DotsVeryRare);
}
}
for (k = i; k < 6; k++)
{
string ntl = "OPos" + k;
string ntl2 = ntl + "2";
ChartObjects.RemoveObject(ntl);
ChartObjects.RemoveObject(ntl2);
}
}
else
{
ChartObjects.RemoveObject("agg o");
ChartObjects.RemoveObject("agg ou");
ChartObjects.RemoveObject("aggn o");
ChartObjects.RemoveObject("ogg d");
ChartObjects.RemoveObject("ot d");
ChartObjects.RemoveObject("ot25 d");
ChartObjects.RemoveObject("ot30 d");
ChartObjects.RemoveObject("ot50 d");
ChartObjects.RemoveObject("ox d");
ChartObjects.RemoveObject("omx");
ChartObjects.RemoveObject("omn");
for (k = 1; k < 6; k++)
{
string ntl = "OPos" + k;
string ntl2 = ntl + "2";
ChartObjects.RemoveObject(ntl);
ChartObjects.RemoveObject(ntl2);
}
}
if (Positions.Count == 0)
{
ChartObjects.RemoveObject("agg dd");
ChartObjects.RemoveObject("agg um");
ChartObjects.RemoveObject("gpu");
ChartObjects.RemoveObject("agg cp");
ChartObjects.RemoveObject("agg d");
ChartObjects.RemoveObject("dpd");
ChartObjects.RemoveObject("t u");
ChartObjects.RemoveObject("t25 u");
ChartObjects.RemoveObject("t30 u");
ChartObjects.RemoveObject("t50 u");
ChartObjects.RemoveObject("ttd3");
ChartObjects.RemoveObject("ttd4");
ChartObjects.RemoveObject("ttd5");
ChartObjects.RemoveObject("pmx");
ChartObjects.RemoveObject("pmn");
for (k = 1; k < 6; k++)
{
string ntl = "Pos" + k;
string ntl2 = ntl + "2";
ChartObjects.RemoveObject(ntl);
ChartObjects.RemoveObject(ntl2);
}
if (pg > 0)
{
ChartObjects.DrawText("aggt", bg, index + 5, p - g, VerticalAlignment.Top, HorizontalAlignment.Right, Colors.Bisque);
ChartObjects.DrawText("aggtu", bg, index + 5, p + g, VerticalAlignment.Top, HorizontalAlignment.Right, Colors.Bisque);
ChartObjects.DrawLine("agg tu", index - 1, p - g, index + 10, p - g, Colors.Bisque);
ChartObjects.DrawLine("agg td", index - 1, p + g, index + 10, p + g, Colors.Bisque);
}
}
else
{
ChartObjects.RemoveObject("aggt");
ChartObjects.RemoveObject("aggtu");
ChartObjects.RemoveObject("agg tu");
ChartObjects.RemoveObject("agg td");
ChartObjects.DrawLine("agg dd", index - 5, Symbol.Bid - x, index + 10, Symbol.Bid - x, Colors.OrangeRed);
ChartObjects.DrawLine("agg um", index - 1, p + g, index + 10, p + g, pColor);
ChartObjects.DrawText("gpu", dp, index + 6, p + g, VerticalAlignment.Top, HorizontalAlignment.Right, tc);
if (pg > 0)
ChartObjects.DrawLine("agg cp", index - 1, p + cp, index + 10, p + cp, pColor, 1, LineStyle.Dots);
ChartObjects.DrawLine("agg d", index - 5, p - d, index + 10, p - d, pColor);
ChartObjects.DrawText("dpd", de, index + 6, p - d, VerticalAlignment.Top, HorizontalAlignment.Right, Colors.DarkRed);
ChartObjects.DrawLine("t u", index - 5, p - d, index + 10, p - d, Colors.AliceBlue);
ChartObjects.DrawLine("t25 u", index - 5, p - d * 2, index + 10, p - d * 2, Colors.AliceBlue, 1, LineStyle.DotsVeryRare);
ChartObjects.DrawLine("t30 u", index - 5, p - d * 3, index + 10, p - d * 3, Colors.AliceBlue, 1, LineStyle.DotsVeryRare);
ChartObjects.DrawLine("t50 u", index - 5, p - d * 4, index + 10, p - d * 4, Colors.AliceBlue, 1, LineStyle.DotsRare);
ChartObjects.DrawText("ttd3", tl3, index + 6, p - d * 2, VerticalAlignment.Top, HorizontalAlignment.Right, Colors.DimGray);
ChartObjects.DrawText("ttd4", tl4, index + 6, p - d * 3, VerticalAlignment.Top, HorizontalAlignment.Right, Colors.DimGray);
ChartObjects.DrawText("ttd5", tl5, index + 6, p - d * 4, VerticalAlignment.Top, HorizontalAlignment.Right, Colors.DimGray);
if (v > 0)
{
ChartObjects.DrawLine("pmn", index - 90, epmx - range, index - 60, epmx - range, Colors.DarkCyan, 1, LineStyle.DotsRare);
for (i = 1; (v + ds * i) <= maxv; i++)
{
string ntl = "Pos" + i;
string ntl2 = ntl + "2";
ChartObjects.DrawLine(ntl, index - 90, epmn - pips * i, index - 60, epmn - pips * i, Colors.DarkCyan, 1, LineStyle.DotsVeryRare);
ChartObjects.DrawLine(ntl2, index - 70, epmn - pips * i + pips / 2, index - 60, epmn - pips * i + pips / 2, Colors.DarkCyan, 1, LineStyle.DotsVeryRare);
}
}
else
{
ChartObjects.DrawLine("pmx", index - 90, epmn + range, index - 60, epmn + range, Colors.DarkCyan, 1, LineStyle.DotsRare);
for (i = 1; (v - ds * i) >= maxv * -1; i++)
{
string ntl = "Pos" + i;
string ntl2 = ntl + "2";
ChartObjects.DrawLine(ntl, index - 90, epmx + pips * i, index - 60, epmx + pips * i, Colors.DarkCyan, 1, LineStyle.DotsVeryRare);
ChartObjects.DrawLine(ntl2, index - 70, epmx + pips * i - pips / 2, index - 60, epmx + pips * i - pips / 2, Colors.DarkCyan, 1, LineStyle.DotsVeryRare);
}
}
for (k = i; k < 6; k++)
{
string ntl = "Pos" + k;
string ntl2 = ntl + "2";
ChartObjects.RemoveObject(ntl);
ChartObjects.RemoveObject(ntl2);
}
}
}
private void localtrend(int li)
{
int count = MarketSeries.Close.Count;
int maxIndex1 = FindNextLocalExtremum(MarketSeries.High, count - 1, true);
int maxIndex2 = FindNextLocalExtremum(MarketSeries.High, maxIndex1 - trendperiod, true);
int minIndex1 = FindNextLocalExtremum(MarketSeries.Low, count - 1, false);
int minIndex2 = FindNextLocalExtremum(MarketSeries.Low, minIndex1 - trendperiod, false);
int startIndex = Math.Min(maxIndex2, minIndex2) - 100;
int endIndex = count + 100;
double mx = MarketSeries.High[maxIndex1];
double mn = MarketSeries.Low[minIndex1];
double diff = mx - mn;
Colors bull = Colors.DarkGreen;
Colors strongbull = Colors.Green;
Colors bear = Colors.DarkRed;
Colors strongbear = Colors.Red;
string marketstatus = "EMA Trend: ";
if (ema50.Result.LastValue > ema200.Result.LastValue)
{
marketstatus = marketstatus + "Up\n";
mainup = true;
}
else
{
marketstatus = marketstatus + "Down\n";
mainup = false;
}
if (maxIndex1 < minIndex1)
{
// bullish retracement
rp = (int)((Symbol.Bid - min) / diff * 100);
marketstatus = marketstatus + "Retracing: Up (" + rp + "%)\n";
bearres = false;
bullres = false;
brokeres = false;
ChartObjects.DrawLine("236", li - 45, mx - diff * 0.764, li - 1, mx - diff * 0.764, bear);
ChartObjects.DrawLine("382", li - 45, mx - diff * 0.382, li - 1, mx - diff * 0.382, strongbear, 1);
ChartObjects.DrawLine("500", li - 45, mx - diff * 0.5, li - 1, mx - diff * 0.5, bear, 1, LineStyle.Dots);
ChartObjects.DrawLine("618", li - 45, mx - diff * 0.618, li - 1, mx - diff * 0.618, strongbear, 1);
if ((mx - diff * 0.764) - Symbol.Bid < 5 * Symbol.PipSize && Symbol.Bid < (mx - diff * 0.764))
marketstatus = marketstatus + " (approaching resistance)\n";
if (Symbol.Bid > (mx - diff * 0.764) && Symbol.Bid < (mx - diff * 0.5))
{
marketstatus = marketstatus + " (within bearish resistance)\n";
bearres = true;
}
if (Symbol.Bid > (mx - diff * 0.5) && Symbol.Bid < (mx - diff * 0.382))
{
marketstatus = marketstatus + " (within bullish resistance)\n";
bullres = true;
}
if (Symbol.Bid > (mx - diff * 0.382 + range / 10 / Symbol.PipSize))
{
marketstatus = marketstatus + " (broke resistance)\n";
brokeres = true;
}
else if (Symbol.Bid > (mx - diff * 0.382))
marketstatus = marketstatus + " (breaking resistance)\n";
localup = true;
}
else
{
// bearish retracement
rp = (int)((mx - Symbol.Bid) / diff * 100);
marketstatus = marketstatus + "Retracing: Down (" + rp + "%)\n";
bullsup = false;
bearsup = false;
brokesup = false;
ChartObjects.DrawLine("236", li - 45, mn + diff * 0.764, li - 1, mn + diff * 0.764, bull);
ChartObjects.DrawLine("382", li - 45, mn + diff * 0.382, li - 1, mn + diff * 0.382, strongbull, 1);
ChartObjects.DrawLine("500", li - 45, mn + diff * 0.5, li - 1, mn + diff * 0.5, bull, 1, LineStyle.Dots);
ChartObjects.DrawLine("618", li - 45, mn + diff * 0.618, li - 1, mn + diff * 0.618, strongbull, 1);
if (Symbol.Bid - (mn + diff * 0.764) < 5 * Symbol.PipSize && Symbol.Bid > (mn + diff * 0.764))
marketstatus = marketstatus + " (approaching support)\n";
if (Symbol.Bid < (mn + diff * 0.764) && Symbol.Bid > (mn + diff * 0.5))
{
marketstatus = marketstatus + " (within bullish support)\n";
bullsup = true;
}
if (Symbol.Bid < (mn + diff * 0.5) && Symbol.Bid > (mn + diff * 0.382))
{
marketstatus = marketstatus + " (within bearish support)\n";
bearsup = true;
}
if (Symbol.Bid < (mn + diff * 0.382 - range / 10 / Symbol.PipSize))
{
marketstatus = marketstatus + " (broke support)\n";
brokesup = true;
}
else if (Symbol.Bid < (mn + diff * 0.382))
marketstatus = marketstatus + " (breaking support)\n";
localup = false;
}
if (Symbol.Bid < min + f38)
{
marketstatus = marketstatus + "Bearish";
immdup = false;
}
if (Symbol.Bid > min + f61)
{
marketstatus = marketstatus + "Bullish";
immdup = true;
}
bullrange = false;
bearrange = false;
if (Symbol.Bid < min + f50 && Symbol.Bid > min + f38)
{
marketstatus = marketstatus + "Bearish range";
bearrange = true;
}
if (Symbol.Bid > min + f50 && Symbol.Bid < min + f61)
{
marketstatus = marketstatus + "Bullish range";
bullrange = true;
}
ChartObjects.DrawText("marketstatus", marketstatus, StaticPosition.TopCenter);
ChartObjects.RemoveObject("suggestion");
ChartObjects.RemoveObject("bulr");
ChartObjects.RemoveObject("bearr");
if ((mainup && !localup && bullsup && bullrange) ^ (!mainup && immdup && brokeres))
{
ChartObjects.DrawText("suggestion", "!! BUY !!\n", StaticPosition.BottomCenter, Colors.Green);
// \n" + " mainup:" + mainup + " localup:" + localup + " immdup:" + immdup + " bearsup:" + bearsup + " bullres:" + bullres + " bearres:" + bearres + " brokesup:" + brokesup + " brokeres:" + brokeres, StaticPosition.BottomCenter, Colors.LimeGreen);
}
if ((!mainup && localup && bearres && bearrange) ^ (mainup && !immdup && brokesup))
{
ChartObjects.DrawText("suggestion", "!! SELL !!\n", StaticPosition.BottomCenter, Colors.Red);
}
if (bullres)
ChartObjects.DrawText("bulr", "(caution - potential bullish reversal)", StaticPosition.BottomCenter, Colors.Green);
if (bearsup)
ChartObjects.DrawText("bearr", "(caution - potential bearish reversal)", StaticPosition.BottomCenter, Colors.Red);
}
private int FindNextLocalExtremum(DataSeries series, int maxIndex, bool findMax)
{
for (int index = maxIndex; index >= 0; index--)
{
if (IsLocalExtremum(series, index, findMax))
{
return index;
}
}
return 0;
}
private bool IsLocalExtremum(DataSeries series, int index, bool findMax)
{
int end = Math.Min(index + trendperiod, series.Count - 1);
int start = Math.Max(index - trendperiod, 0);
double value = series[index];
for (int i = start; i < end; i++)
{
if (findMax && value < series[i])
return false;
if (!findMax && value > series[i])
return false;
}
return true;
}
}
}
solid
Joined on 08.02.2018
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Solid Horizon.algo
- Rating: 5
- Installs: 2940
- Modified: 13/10/2021 09:54
Comments
Good day to you and thank you for your kind response
Again I would say yes it is a great tool you have built here
But I will keep pushing on you to have a multi time frame info on your great indicator :)
I totally agree with you on risk control/management and I believe part of it is also to take decision to not take a trade and not only how much we stand to loss :) and on other hand why not use the indicator to make money :$
Back to multi time info : ) take example of GBPJPY on H4 Trend UP and on M30 Trend Down here some traders might decide to wait so to save time no need to switch between time frames to find about trend status it will be great to have it on same time frame you looking at
I have another Idea to add it to have this great indicator more powerful and more risk control useful tool : )
Yay!
Thank you for the kind words; indeed, the idea was to make it useful. As, the idea developed, it became more about a risk control. So, not about how much you could make, but about how much you would stand to lose.
In that regard, I need to add to my answer to the other question; as now, I've realized which dotted lines were in question. Thoses dotted lines are the ones that show you, where to place your bets when market moves against you. And the longer one, is where you should probably give up on the idea all together.
To answer the question about different time-frames, in fact the indicator already does calculate that, so the top - middle - 3 lines are actually showing local, and "global" trend of an instrument. (I think they should anyway, as lots of beers were involved, as can probably be seen by some of the variables names). But yep, there you will see local trend vs global.
Regarding, MT4 - nope, have stopped using the platform a while back, while it's a great platform, just didn't feel it was as easy to cope with as ct.
Hi,
Thanks for the great Indicator
Very useful, if I may add suggestion to improve the usefulness of the indicator for the users, is if possible for you to add option to show the trend status on two more time frames in addition to current
So for example current time frame is H1 and say to show in addition to it the trend for H4 and daily trend details
And one more question do you have the same for MT4 :)
Yo!
thanks for the feedback; glad a couple of guys found it useful :)
To answer your question: the dotted lines are FIB retracement levels of the larger trend; so if you zoom out, you will see yellow lines showing the "local" price action max/min levels, so the dotted lines are basically 50%, the red one, and 23.6 the orange ones; quite common you will see market bounce somewhere around the orange lines - not sure while people believe in Fib so much, but hey, if it works - it works. Play around on a few different instruments on daily charts, and you will see this to hold true.
Regarding your 2nd question about numbers in the corners; well the indicators thinks it's smarter than you, so it tries to give you some idea about how much you should be making. Top right number is the daily, bottom right is the weekly, and low left is your positions, with recommended position size - this will change to "good to close", when indicator believes it's time to book profits.
o/
Hi, Thanks for this fantastic indicator. Could you tell me briefly what the dotted lines mean? (and the numbers in the corners, of course). Thank you
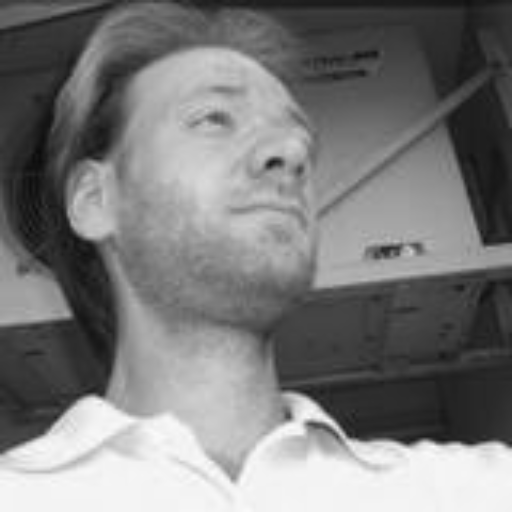
there is some extremely interesting stuff in your code .. which provides a ton of good examples.
DAIZEL TRANSLATOR
visit : penerjemah tersumpah