Description
An advanced version of the Hedging and Scalping cBot. It implements an automatic position scaling out logic and has good returns on many timeframes and time periods. See below backtesting results on minute timeframe over a two years period.
using System;
using System.Linq;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
namespace cAlgo
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class AdvancedHedgingandScalpingcBot : Robot
{
[Parameter("Volume", DefaultValue = 1000, MinValue = 1, Step = 1)]
public int Volume { get; set; }
private double _equity;
private int noOfPositions = 4;
private int pips = 2;
protected override void OnStart()
{
_equity = Account.Balance;
ExecuteMarketOrder(TradeType.Buy, Symbol, Volume, "BUY");
ExecuteMarketOrder(TradeType.Sell, Symbol, Volume, "SELL");
}
protected override void OnBar()
{
foreach (var position in Positions)
{
if (position.Pips > pips)
{
ClosePosition(position);
}
}
if (Positions.Count < noOfPositions)
{
ExecuteMarketOrder(TradeType.Buy, Symbol, Volume, "BUY");
ExecuteMarketOrder(TradeType.Sell, Symbol, Volume, "SELL");
}
int buyCount = 0;
int sellCount = 0;
foreach (var position in Positions)
{
if (position.TradeType == TradeType.Buy)
buyCount++;
if (position.TradeType == TradeType.Sell)
sellCount++;
}
if (buyCount == 0 || sellCount == 0)
{
Volume += 1000;
noOfPositions += 2;
pips++;
ExecuteMarketOrder(TradeType.Buy, Symbol, Volume, "BUY");
ExecuteMarketOrder(TradeType.Sell, Symbol, Volume, "SELL");
}
if (Account.Equity > _equity + Account.Equity / 100)
{
foreach (var position in Positions)
{
ClosePosition(position);
}
_equity = Account.Equity;
Volume = 1000;
noOfPositions = 4;
pips = 2;
ExecuteMarketOrder(TradeType.Buy, Symbol, Volume, "BUY");
ExecuteMarketOrder(TradeType.Sell, Symbol, Volume, "SELL");
}
}
}
}
skoutz.rothchild
Joined on 13.03.2017
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Advanced Hedging and Scalping cBot.algo
- Rating: 0
- Installs: 6840
- Modified: 13/10/2021 09:54
Comments
hello how can i add a stoploss in this bot. i like the bot but i miss a stoploss to avoid blowing my account
How do I stop the robot from opening more trades once the ones that are open get closed?
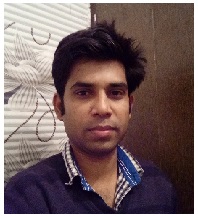
Good to Play With, not for real trade...
Greeting to you skoutz.rothchild! I appreciate the work you sharing with others.
Can you please describe the exact logic of this code and how differently it performs compared to your first H&S cBot?
Thank you
Thanks a lot, im doing backtesting and sometimes really good, but i have time which my Account gettin zero. Can you please add Stopp Loss? What Pait do you use with timeframe? Thanks Ian
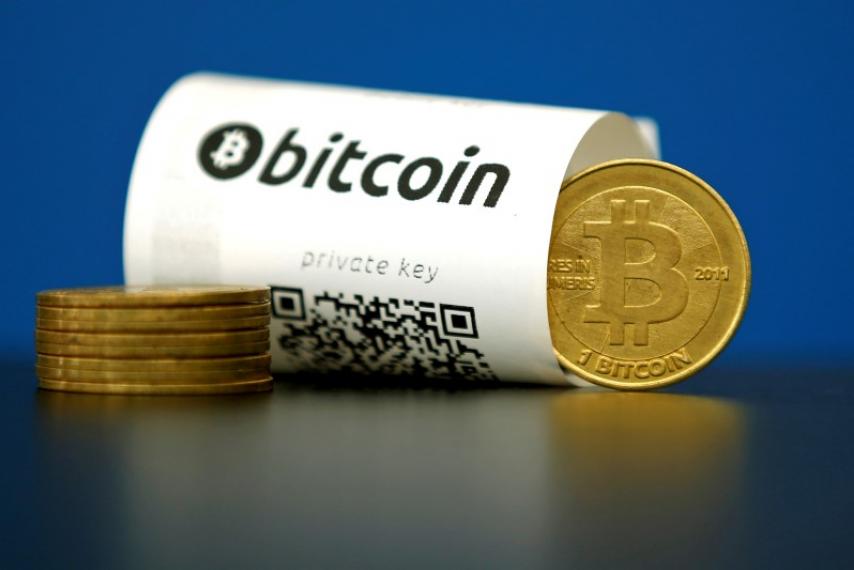
Power Full Backtest
Thank you
can you write a code like this one?
can you make just a hedging bot like ea recovery zone
when i use this bot it just opens buys and sells at the same time