Warning! This section will be deprecated on February 1st 2025. Please move all your Indicators to the cTrader Store catalogue.
Description
This indicator is for 5min or 15min chart and it contains:
- 25 Color EMA
- 70 Color EMA
- Position Size Calculator Acourding to your risk percentage
- Shows you the level of SL and TP with Risk Reward Ratio of 1:2 accourding to your SL
I personally use for my 5min chart combined with Asia_Range Indicator.
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo.Indicators
{
[Indicator(IsOverlay = true, TimeZone = TimeZones.EAfricaStandardTime, AccessRights = AccessRights.None)]
public class MyIndicator : Indicator
{
//-----------PARAMETER---------------------------
[Parameter("Info", DefaultValue = 1)]
public bool info { get; set; }
[Parameter("Stop Loss", DefaultValue = 6)]
public int StopLoss { get; set; }
[Parameter("Stop Loss Risk %", DefaultValue = 1)]
public double stopLossRiskPercent { get; set; }
public int TakeProfit;
[Parameter(DefaultValue = 0)]
public double SydneyOpen { get; set; }
public ExponentialMovingAverage ema;
[Parameter("Period", DefaultValue = 25)]
public int Period { get; set; }
[Parameter("Source")]
public DataSeries Source { get; set; }
public ExponentialMovingAverage sema;
[Parameter("Slow Period", DefaultValue = 70)]
public int sPeriod { get; set; }
[Parameter("Slow Source")]
public DataSeries sSource { get; set; }
//------------------OUTPUT----------------------------------------------------------
[Output("Fast Main", Color = Colors.Black)]
public IndicatorDataSeries Result { get; set; }
[Output("Fast UP", Color = Colors.Green, PlotType = PlotType.DiscontinuousLine)]
public IndicatorDataSeries Up { get; set; }
[Output("Fast Down", Color = Colors.OrangeRed, PlotType = PlotType.DiscontinuousLine)]
public IndicatorDataSeries Down { get; set; }
[Output("Slow Main", Color = Colors.Black)]
public IndicatorDataSeries sResult { get; set; }
[Output("Slow UP", Color = Colors.Green, PlotType = PlotType.DiscontinuousLine)]
public IndicatorDataSeries sUp { get; set; }
[Output("Slow Down", Color = Colors.OrangeRed, PlotType = PlotType.DiscontinuousLine)]
public IndicatorDataSeries sDown { get; set; }
[Output("Bull Take Profit", Color = Colors.Green, PlotType = PlotType.Points, Thickness = 1)]
public IndicatorDataSeries BullTP { get; set; }
[Output("Bull Stop Loss", Color = Colors.Green, PlotType = PlotType.Points, Thickness = 1)]
public IndicatorDataSeries BullSL { get; set; }
[Output("Bear Take Profit", Color = Colors.OrangeRed, PlotType = PlotType.Points, Thickness = 1)]
public IndicatorDataSeries BearTP { get; set; }
[Output("Bear Stop Loss", Color = Colors.OrangeRed, PlotType = PlotType.Points, Thickness = 1)]
public IndicatorDataSeries BearSL { get; set; }
protected override void Initialize()
{
ema = Indicators.ExponentialMovingAverage(Source, Period);
sema = Indicators.ExponentialMovingAverage(sSource, sPeriod);
}
public override void Calculate(int index)
{
Result[index] = ema.Result[index];
if (Result[index - 1] < Result[index])
{
Up[index] = Result[index];
Down[index] = double.NaN;
}
else
{
Up[index] = double.NaN;
Down[index] = Result[index];
}
sResult[index] = sema.Result[index];
if (sResult[index - 1] < sResult[index])
{
sUp[index] = sResult[index];
sDown[index] = double.NaN;
}
else
{
sUp[index] = double.NaN;
sDown[index] = sResult[index];
}
TakeProfit = StopLoss * 2;
if (info == true)
{
DisplayPositionSizeRiskOnChart();
}
if (TimeFrame < TimeFrame.Minute10)
{
VerticalLineSydney(index);
//-----------TAKE PROFIT AND STOP LOSS INDICATOR---------------------------
BullTP[index] = double.NaN;
BullSL[index] = double.NaN;
BearTP[index] = double.NaN;
BearSL[index] = double.NaN;
BullTP[index + 1] = MarketSeries.Close[index] + TakeProfit * Symbol.PipSize;
BullSL[index + 1] = MarketSeries.Close[index] - StopLoss * Symbol.PipSize;
BearTP[index + 1] = MarketSeries.Close[index] - TakeProfit * Symbol.PipSize;
BearSL[index + 1] = MarketSeries.Close[index] + StopLoss * Symbol.PipSize;
}
}
//---------VERTICAL LINE----------------------------------------------------------
// Sydney
protected void VerticalLineSydney(int index)
{
if (MarketSeries.OpenTime[index].Hour == SydneyOpen & MarketSeries.OpenTime[index].Minute == 5)
{
ChartObjects.DrawVerticalLine("SydneyLine" + index, index, Colors.Green, 1, LineStyle.LinesDots);
}
}
// Position Size Calculator
private void DisplayPositionSizeRiskOnChart()
{
double costPerPip = (double)((int)(Symbol.PipValue * 10000000)) / 100;
double positionSizeForRisk = (Account.Balance * stopLossRiskPercent / 100) / (StopLoss * costPerPip);
string text = "SL is " + StopLoss + " pips = " + Math.Round(positionSizeForRisk, 2) + " Lot";
ChartObjects.DrawText("positionRisk", text, StaticPosition.TopLeft, Colors.Gray);
if (TimeFrame < TimeFrame.Minute30)
{
string size = "\n" + "06 pips = " + Math.Round((Account.Balance * stopLossRiskPercent / 100) / (6 * costPerPip), 2) + " Lot";
size = size + "\n" + "08 pips = " + Math.Round((Account.Balance * stopLossRiskPercent / 100) / (8 * costPerPip), 2) + " Lot";
size = size + "\n" + "10 pips = " + Math.Round((Account.Balance * stopLossRiskPercent / 100) / (10 * costPerPip), 2) + " Lot";
size = size + "\n" + "12 pips = " + Math.Round((Account.Balance * stopLossRiskPercent / 100) / (12 * costPerPip), 2) + " Lot";
size = size + "\n" + "15 pips = " + Math.Round((Account.Balance * stopLossRiskPercent / 100) / (15 * costPerPip), 2) + " Lot";
ChartObjects.DrawText("calculator", size, StaticPosition.TopLeft, Colors.Silver);
}
}
}
}
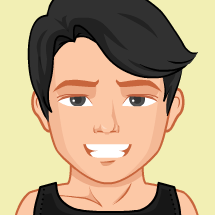
BigDeal
Joined on 03.12.2015
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: My Indicator.algo
- Rating: 0
- Installs: 3854
- Modified: 13/10/2021 09:54
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
Any chance you can make just the position size calculator but with units instead of lots? Also would like to be able to put it on the right top corner, instead of left top corner? :) Thanks