Description
using System; using System.Linq; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; namespace cAlgo { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class CCIReversal : Robot { [Parameter(DefaultValue = 26)] public int cciPeriod { get; set; } [Parameter(DefaultValue = 9)] public int maPeriod { get; set; } [Parameter("Quantity (Lots)", DefaultValue = 1, MinValue = 0.01, Step = 0.01)] public double Quantity { get; set; } private CommodityChannelIndex cci; //private Position _position; private const string robotname = "CCIReversal"; protected override void OnStart() { cci = Indicators.CommodityChannelIndex(cciPeriod); } protected override void OnBar() { var longPos = Positions.Find(robotname, Symbol, TradeType.Buy); var shortPos = Positions.Find(robotname, Symbol, TradeType.Sell); var pre_cci = cci.Result.Last(2); var cur_cci = cci.Result.Last(0); bool LongPosition = _position != null && _position.TradeType == TradeType.Buy; bool ShortPosition = _position != null && _position.TradeType == TradeType.Sell; Print("CCI = " + cur_cci + " Pre CCI" + pre_cci); if ((cci.Result.HasCrossedAbove(-100.0, 1) && cur_cci > pre_cci) || (cci.Result.HasCrossedAbove(100.0, 1) && cur_cci > pre_cci)) { if (shortPos != null) { ClosePosition(shortPos); ExecuteMarketOrder(TradeType.Buy, Symbol, VolumeInUnits, robotname); } else if (longPos == null) { ExecuteMarketOrder(TradeType.Buy, Symbol, VolumeInUnits, robotname); } else { ExecuteMarketOrder(TradeType.Buy, Symbol, VolumeInUnits, robotname); } Print("con1"); } else if ((cci.Result.HasCrossedBelow(-100.0, 1) && cur_cci < pre_cci) || (cci.Result.HasCrossedBelow(100, 1) && cur_cci < pre_cci)) { if (longPos != null) { ClosePosition(longPos); ExecuteMarketOrder(TradeType.Sell, Symbol, VolumeInUnits, robotname); } else if (shortPos == null) { ExecuteMarketOrder(TradeType.Sell, Symbol, VolumeInUnits, robotname); } else { ExecuteMarketOrder(TradeType.Sell, Symbol, VolumeInUnits, robotname); } Print("con2"); } Print("Long" + (longPos != null)); Print("Short" + (shortPos != null)); } private long VolumeInUnits { get { return Symbol.QuantityToVolume(Quantity); } } } /* private void Close(TradeType tradeType) { foreach (var position in Positions.FindAll(robotname, Symbol, tradeType)) ClosePosition(_position); } private void Open(TradeType tradeType) { _position = Positions.Find(robotname, Symbol, tradeType); var volumeInUnits = Symbol.QuantityToVolume(Quantity); if (_position == null) ExecuteMarketOrder(tradeType, Symbol, volumeInUnits, robotname); }*/ }
Backtest on Silver
The CCI Reversal Buy when CCI(26) Cross above -100 or 100(when no buy position open), in the case of crossing above -100 the cbot will be selling when CCI(26) cross below 0, but if the CCI(26) reach over 100 it will be selling when CCI(26) cross below 100 .
In case of short position, it's the reversal of buy position.
There is parameter name 'MA' but notthing importance for now, I added it for the next version.
and the parameter Trading Size, value 2 mean trade both side long and short, 0 = trade on short side only, 1 = trade on long side only.
If you find any mistake of this algorithm please let me know.
using System;
using System.Linq;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
namespace cAlgo
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class CCIReversal : Robot
{
[Parameter(DefaultValue = 26)]
public int cciPeriod { get; set; }
[Parameter(DefaultValue = 9)]
public int maPeriod { get; set; }
[Parameter("Quantity (Lots)", DefaultValue = 1, MinValue = 0.01, Step = 0.01)]
public double Quantity { get; set; }
[Parameter("Trading Side", DefaultValue = 2, MinValue = 0, MaxValue = 2, Step = 1)]
public int tradeSize { get; set; }
private CommodityChannelIndex cci;
//private Position _position;
private const string robotname = "CCIReversal";
private int side;
//private int magic;
protected override void OnStart()
{
cci = Indicators.CommodityChannelIndex(cciPeriod);
side = tradeSize;
}
protected override void OnBar()
{
var longPos = Positions.Find(robotname, Symbol, TradeType.Buy);
var shortPos = Positions.Find(robotname, Symbol, TradeType.Sell);
var pre_cci = cci.Result.Last(2);
var cur_cci = cci.Result.Last(0);
Print("CCI = " + cur_cci + " Pre CCI" + pre_cci);
if ((cci.Result.HasCrossedAbove(-100.0, 1) && cur_cci > pre_cci) || (cci.Result.HasCrossedAbove(100.0, 1) && cur_cci > pre_cci))
{
if (shortPos != null)
{
ClosePosition(shortPos);
if (side == 1 || side == 2)
{
ExecuteMarketOrder(TradeType.Buy, Symbol, VolumeInUnits, robotname);
}
}
else if (longPos == null)
{
if (side == 1 || side == 2)
{
ExecuteMarketOrder(TradeType.Buy, Symbol, VolumeInUnits, robotname);
}
}
else if (longPos != null)
{
/*if (side == 1 || side == 2)
{
ExecuteMarketOrder(TradeType.Buy, Symbol, VolumeInUnits, robotname);
}*/
}
Print("con1");
}
else if ((cci.Result.HasCrossedBelow(-100.0, 1) && cur_cci < pre_cci) || (cci.Result.HasCrossedBelow(100, 1) && cur_cci < pre_cci))
{
if (longPos != null)
{
ClosePosition(longPos);
if (side == 0 || side == 2)
{
ExecuteMarketOrder(TradeType.Sell, Symbol, VolumeInUnits, robotname);
}
}
else if (shortPos == null)
{
if (side == 0 || side == 2)
{
ExecuteMarketOrder(TradeType.Sell, Symbol, VolumeInUnits, robotname);
}
}
else if (shortPos != null)
{
/*if (side == 0 || side == 2)
{
ExecuteMarketOrder(TradeType.Sell, Symbol, VolumeInUnits, robotname);
}*/
}
Print("con2");
}
Print("Long" + (longPos != null));
Print("Short" + (shortPos != null));
}
private long VolumeInUnits
{
get { return Symbol.QuantityToVolume(Quantity); }
}
private void cci_reversal()
{
}
}
}
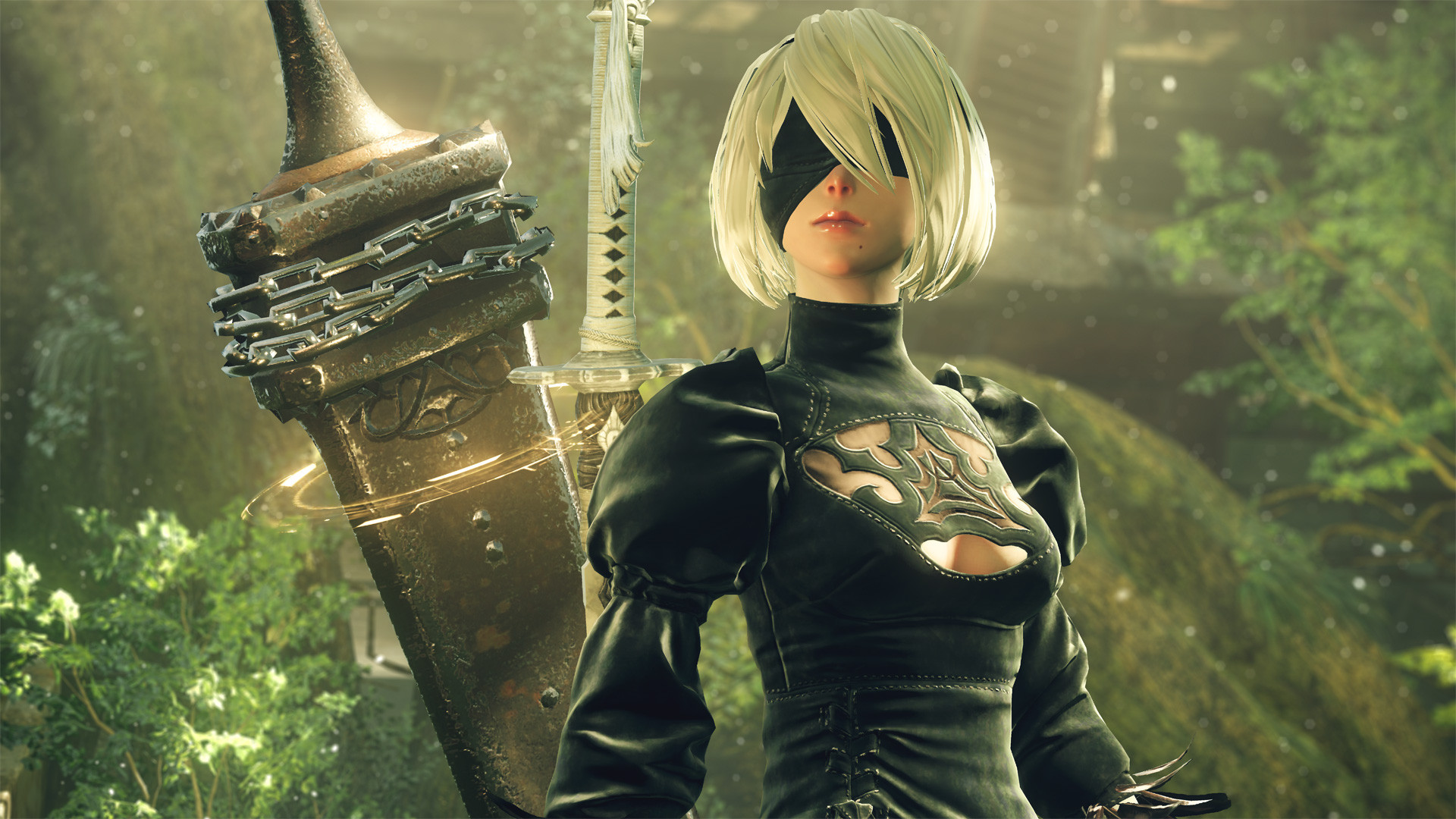
jamikorn.k.1990
Joined on 10.11.2016
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: CCI Reversal.algo
- Rating: 0
- Installs: 3422
Comments
I tried to add stop loss and take profit but I failed. Anyone can help what to add and where?
Where do you guys subscribe to sports news information?
You can see all the major sites and various useful articles.
We recommend checking it out now.
메이저사이트