Description
Trend Indicator showing an Up Trend when the current close price is higher than the main trend line in uptrend and a Down Trend when the current close price is lower than main trend line in downtrend. The main trend line in uptrend equals to the median price plus a multiple of the average true range and the main line in downtrend equals the median price minus the multiple of the average true range.
using System;
using cAlgo.API;
using cAlgo.API.Indicators;
namespace cAlgo.Indicators
{
[Indicator(IsOverlay = true, AccessRights = AccessRights.None)]
public class Supertrend : Indicator
{
[Parameter(DefaultValue = 10)]
public int Period { get; set; }
[Parameter(DefaultValue = 3.0)]
public double Multiplier { get; set; }
[Output("UpTrend", Color = Colors.Green, PlotType = PlotType.Points, Thickness = 3)]
public IndicatorDataSeries UpTrend { get; set; }
[Output("DownTrend", Color = Colors.Red, PlotType = PlotType.Points, Thickness = 3)]
public IndicatorDataSeries DownTrend { get; set; }
private IndicatorDataSeries _upBuffer;
private IndicatorDataSeries _downBuffer;
private AverageTrueRange _averageTrueRange;
private int[] _trend;
private bool _changeofTrend;
protected override void Initialize()
{
_trend = new int[1];
_upBuffer = CreateDataSeries();
_downBuffer = CreateDataSeries();
_averageTrueRange = Indicators.AverageTrueRange(Period, MovingAverageType.WilderSmoothing);
}
public override void Calculate(int index)
{
// Init
UpTrend[index] = double.NaN;
DownTrend[index] = double.NaN;
double median = (MarketSeries.High[index] + MarketSeries.Low[index]) / 2;
double atr = _averageTrueRange.Result[index];
_upBuffer[index] = median + Multiplier * atr;
_downBuffer[index] = median - Multiplier * atr;
if (index < 1)
{
_trend[index] = 1;
return;
}
Array.Resize(ref _trend, _trend.Length + 1);
// Main Logic
if (MarketSeries.Close[index] > _upBuffer[index - 1])
{
_trend[index] = 1;
if (_trend[index - 1] == -1)
_changeofTrend = true;
}
else if (MarketSeries.Close[index] < _downBuffer[index - 1])
{
_trend[index] = -1;
if (_trend[index - 1] == -1)
_changeofTrend = true;
}
else if (_trend[index - 1] == 1)
{
_trend[index] = 1;
_changeofTrend = false;
}
else if (_trend[index - 1] == -1)
{
_trend[index] = -1;
_changeofTrend = false;
}
if (_trend[index] < 0 && _trend[index - 1] > 0)
_upBuffer[index] = median + (Multiplier * atr);
else if (_trend[index] < 0 && _upBuffer[index] > _upBuffer[index - 1])
_upBuffer[index] = _upBuffer[index - 1];
if (_trend[index] > 0 && _trend[index - 1] < 0)
_downBuffer[index] = median - (Multiplier * atr);
else if (_trend[index] > 0 && _downBuffer[index] < _downBuffer[index - 1])
_downBuffer[index] = _downBuffer[index - 1];
// Draw Indicator
if (_trend[index] == 1)
{
UpTrend[index] = _downBuffer[index];
if (_changeofTrend)
{
UpTrend[index - 1] = DownTrend[index - 1];
_changeofTrend = false;
}
}
else if (_trend[index] == -1)
{
DownTrend[index] = _upBuffer[index];
if (_changeofTrend)
{
DownTrend[index - 1] = UpTrend[index - 1];
_changeofTrend = false;
}
}
}
}
}
spka111
Joined on 16.08.2012
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Supertrend.algo
- Rating: 3.75
- Installs: 18226
- Modified: 13/10/2021 09:55
Comments
For Lines only change line 16,19 into PlotType.DiscontinuousLine
ale someone ablle to add an alert sound by changing the direction of super trend ?
there is stop loss and take profit (at 5 and 20) for example). what is this number mean is it 5 bars or 5 minuetes or 5 Pips or what exactly
I also prefer it to lines rather than dots. Please help in this regard
HI at all, i'm new here and i need help..
Some one can tell me in wich way i can change Supertrend indicator from Dots ti Lines?
Thanks
Hi spka111,
I modified a little of your Indicator and referenced to you. If you do not want it referenced or displayed in the indicator section please say so and I will remove it.
https://ctrader.com/algos/indicators/show/1775
Kind regards,
Vince
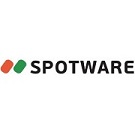
Is not there a mistake at line 67?