Description
- Plots custom period separator.
Updates:
- 21/06/2016 - Version 1.0
- Released.
Screenshot:
Notes:
- Works on tick charts as well.
Make a Donation:
- If you like my work and effort then please consider to make a kind donation thru PayPal or any Credit Card via this link.
using System;
using cAlgo.API;
namespace cAlgo
{
[Indicator(IsOverlay = true, AutoRescale = false, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class CustomPeriodSeparator : Indicator
{
[Parameter(DefaultValue = 60)]
public int Minutes { get; set; }
[Output("Separator", PlotType = PlotType.Histogram)]
public IndicatorDataSeries Separator { get; set; }
private IndicatorDataSeries totalMinutes;
private double lastSeparatorMinutes;
protected override void Initialize()
{
totalMinutes = CreateDataSeries();
lastSeparatorMinutes = TotalMinutes(MarketSeries.OpenTime[0]);
lastSeparatorMinutes -= lastSeparatorMinutes % Minutes;
}
public override void Calculate(int index)
{
totalMinutes[index] = TotalMinutes(MarketSeries.OpenTime[index]);
if (totalMinutes[index] >= lastSeparatorMinutes + Minutes)
{
lastSeparatorMinutes = totalMinutes[index];
lastSeparatorMinutes -= lastSeparatorMinutes % Minutes;
Separator[index] = MarketSeries.High.Maximum(MarketSeries.Open.Count) * 1.2;
}
if (MarketSeries.OpenTime[index].Day != MarketSeries.OpenTime[index - 1].Day)
{
lastSeparatorMinutes = 0;
Separator[index] = MarketSeries.High.Maximum(MarketSeries.Open.Count) * 1.2;
}
}
private double TotalMinutes(DateTime dt)
{
var delta = dt.Ticks % TimeSpan.FromMinutes(1).Ticks;
var dateTime = new DateTime(dt.Ticks - delta, dt.Kind);
return dateTime.Subtract(dateTime.Date).TotalMinutes;
}
}
}
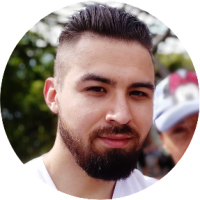
Jiri
Joined on 31.08.2015
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: CustomPeriodSeparator.algo
- Rating: 3.33
- Installs: 3795
- Modified: 13/10/2021 09:55
Comments
I'm very hesitant about downloading and installing any indicator that requires unrestricted access rights. Can you please explain to me why this indicator requires these rights whilst so many others don't? Thank you.
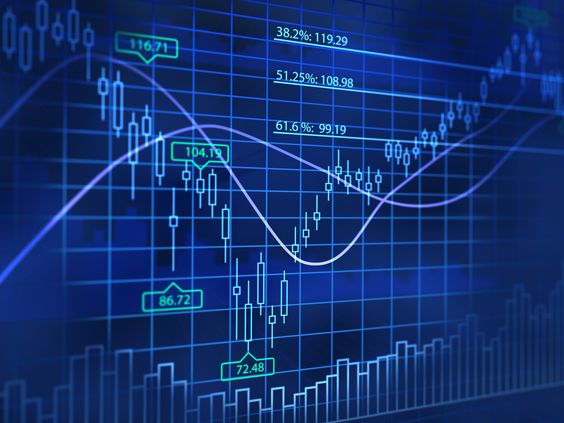
Hi Jiri, this separator plots the start of the day at 3am because my local server is UTC+3 ...
i've tried to change the time zone in the script to UTC+3 to plot the lines on 00:00 but it didn't work..
please tell me how i can do this ?
thank you so much
How come it cannot be downloaded (the Download button is orange and non-operative)?
Hello, this is the indicator I was looking for, but I would like you to also draw the lines of 05:00 future, not just the past ones, is it possible to improve this indicator?
http://pastebin.com/embed_iframe/EAk442yZ
Thank you very much
Best regards
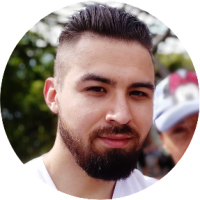
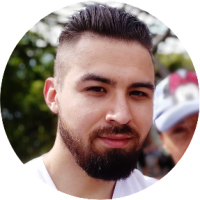
Hi, here you go. http://pastebin.com/embed_iframe/EAk442yZ
Great Stuff TMC!.
I was wondering if its possible to have a period seperator set to any time on my charts, but more specifically for mine id like a period separator on 05:00 UTC+0 of each day?
Any help is much appreciated thankyou!
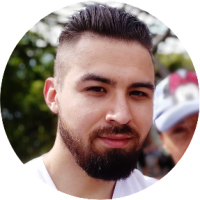
This indicator is meant to be used for intraday trading and it's limited to 1440 minutes (1 day) because it resets on each new day. How would you want to seperate 100 days? Where should it start counting days? On first bar on the chart or first day of the year?
I am trying to searate days but the algo only allows up to 60minutes.. is there a way I can add days? i.e 100 day seperator?
i'm trying to use this indicator on renko charts as default period seperation but by 1440 min it have a offset equal to interval between my time zone and server time zone.
how can i correct that?