Description
This time I'm sharing my personal indicator it does calculates weighted standard deviation per side to get the upper and lower volatility the advantage of differentiating the volatilities is that allows the Cbots and the Trader to identify which side is the market getting increasing volatility, is also useful to set SL & TP protections based on the indicator. I recommend a timeframe = 30M Periods=14.
Example of how to invocate the measurements:
// OnStart() wssd = Indicators.GetIndicator(MarketSeries.Close, 14); // OnTick() double upperVol=wssd.SDUp.LastValue; double lowerVol=wssd.SDDown.LastValue;
Yellow Line represents upper volatility and blue represents lower volatility.
/*+-----------------------------------------------------------------+
| Doux WeightedSideStandardDeviation |
| Copyright 2015 © All Rights Reserved |
+-----------------------------------------------------------------+*/
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
using System.Collections.Generic;
using System.Collections;
namespace cAlgo.Indicators
{
[Indicator(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class WeightedSideStandardDeviation : Indicator
{
[Parameter(DefaultValue = 14, MinValue = 2)]
public int Periods { get; set; }
[Parameter("Source")]
public DataSeries Source { get; set; }
[Output("SDUp", Color = Colors.Orange)]
public IndicatorDataSeries Result { get; set; }
[Output("SDDown", Color = Colors.Aquamarine)]
public IndicatorDataSeries Result1 { get; set; }
protected override void Initialize()
{
summ(Periods);
}
private int summ(int period)
{
int result = 0;
for (int i = 1; i <= period; i++)
result += i;
return result;
}
public override void Calculate(int index)
{
double sumsma = 0.0;
double sumsma2 = 0.0;
int weight = 0;
int weight1 = 0;
for (int i = index - Periods + 1; i <= index; i++)
{
if (Source[i] - Source[i - 1] > 0)
{
weight++;
sumsma += Math.Abs(Source[i] - Source[i - 1]) * weight;
}
else if (Source[i] - Source[i - 1] < 0)
{
weight1++;
sumsma2 += Math.Abs(Source[i] - Source[i - 1]) * weight1;
}
}
int su = summ(weight);
int su2 = summ(weight1);
double average = sumsma / su;
double average1 = sumsma2 / su2;
double sum = 0;
double sum1 = 0;
for (var period = index - Periods + 1; period <= index; period++)
{
if (Source[period] - Source[period - 1] > 0)
sum += Math.Pow(Math.Abs(Source[period] - Source[period - 1]) - average, 2.0);
if (Source[period] - Source[period - 1] < 0)
sum1 += Math.Pow(Math.Abs(Source[period] - Source[period - 1]) - average1, 2.0);
}
Result[index] = Math.Sqrt(sum / su);
Result1[index] = Math.Sqrt(sum1 / su2);
}
}
}
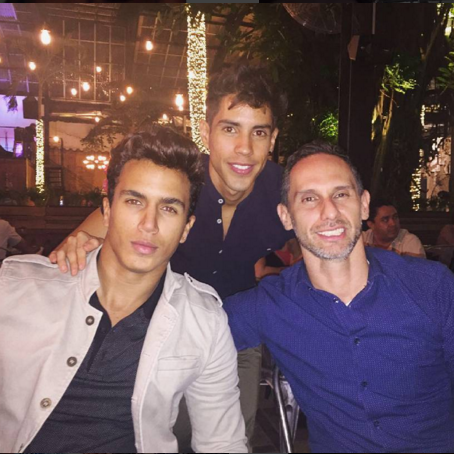
aimerdoux
Joined on 07.06.2015
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: WSSD.algo
- Rating: 0
- Installs: 2674
- Modified: 13/10/2021 09:55
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
Hello and much thanks for sharing your special indicator. I'm testing it to see if it can be useful in somewhere, and noticed there were some mistakes in your: "Example of how to invocate the measurements:"
// OnStart()
wssd = Indicators.GetIndicator(MarketSeries.Close, 14);
// OnTick()
double upperVol=wssd.SDUp.LastValue;
double lowerVol=wssd.SDDown.LastValue;
Corrected lines:
// OnStart()
wssd = Indicators.GetIndicator(14, MarketSeries.Close);
// OnTick()
double upperVol=wssd.Result.LastValue;
double lowerVol=wssd.Result1.LastValue;