Description
This is William Blau Tick Volume Indicator written for cTrader
Combine this with your method of trading at your own risk .
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = false, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class Cyf_TVI : Indicator
{
private IndicatorDataSeries UpTick;
private IndicatorDataSeries DnTick;
private IndicatorDataSeries TVI_Calculate;
private ExponentialMovingAverage EMA_UpTick;
private ExponentialMovingAverage EMA_DnTick;
private ExponentialMovingAverage DEMA_UpTick;
private ExponentialMovingAverage DEMA_DnTick;
private ExponentialMovingAverage TVI;
[Parameter("r", DefaultValue = 12)]
public int EMA { get; set; }
[Parameter("s", DefaultValue = 12)]
public int DEMA { get; set; }
[Parameter("u", DefaultValue = 5)]
public int TEMA { get; set; }
[Output("TVI_Up", PlotType = PlotType.Histogram, Color = Colors.AliceBlue, Thickness = 3)]
public IndicatorDataSeries TVI_Draw_Up { get; set; }
[Output("TVI_Dn", PlotType = PlotType.Histogram, Color = Colors.Red, Thickness = 3)]
public IndicatorDataSeries TVI_Draw_Dn { get; set; }
protected override void Initialize()
{
UpTick = CreateDataSeries();
DnTick = CreateDataSeries();
TVI_Calculate = CreateDataSeries();
EMA_UpTick = Indicators.ExponentialMovingAverage(UpTick, EMA);
EMA_DnTick = Indicators.ExponentialMovingAverage(DnTick, EMA);
DEMA_UpTick = Indicators.ExponentialMovingAverage(EMA_UpTick.Result, DEMA);
DEMA_DnTick = Indicators.ExponentialMovingAverage(EMA_DnTick.Result, DEMA);
TVI = Indicators.ExponentialMovingAverage(TVI_Calculate, TEMA);
}
public override void Calculate(int index)
{
UpTick[index] = (MarketSeries.TickVolume[index] + (MarketSeries.Close[index] - MarketSeries.Open[index]) / Symbol.TickSize) / 2;
DnTick[index] = MarketSeries.TickVolume[index] - UpTick[index];
TVI_Calculate[index] = 100 * ((DEMA_UpTick.Result[index] - DEMA_DnTick.Result[index]) / (DEMA_UpTick.Result[index] + DEMA_DnTick.Result[index]));
if (TVI.Result[index] > TVI.Result[index - 1])
{
TVI_Draw_Up[index] = TVI.Result[index];
}
else
{
TVI_Draw_Dn[index] = TVI.Result[index];
}
}
}
}
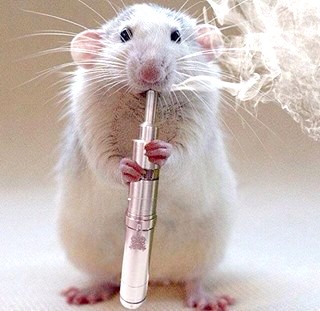
cyfer
Joined on 27.09.2015
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Cyf_TVI.algo
- Rating: 5
- Installs: 4640
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
MA
Hi Cyfer, Thanks for uploading. I am actually also really interested in that matrix indicator you posted; already used it in Metatrader. Is it possible to upload that one as well?
Thanks in advance!
Hello Cyfer,
Thank for sharing. This is a really clever way to look at tick volume!