Description
Hi,
This Indicator basically displays :
1. Displays the PIPS (hourly, daily, weekly, monthly) and
2. Draw Lines through +/- ATR values on the CHART.
Have divided the ATR Value by x (x=1,2,3,4). And converted it to Pips. Added these Pips value to "OPEN PRICES" and drawn the lines on Chart.
How to USE IT :
1. Good for Trade : If the Price has crossed +D-1/2 ATR or -D-1/2 ATR, then it means that this PAIR is good for trading (either Buy or Sell. depending on your other triggers).
2. Trigger : After it has crossed Daily ATR lines, then wait for the prices to cross the "Hourly +ve or -ve" ATR line to start trading. (Direction based on other trigger indicators).
3. Trending : If Both Weekly ATR lines (+ve or -ve) are above Both the Monthly ATR Lines or vice versa. The Pair is trending.
4. Ranging : if Daily lines are within Weekly ATR Lines, and Weekly ATR Line are between the Monthly ATR lines. The Pair is ranging.
5. Short-Term Reversal : if Price have broken all +ve (-ve) Daily, Weekly, Monthly ATR lines in single session. *if it was early in ranging setup.
6. OPEN PRICES : if Daily Open Price is above Weekly Open Price, and Weekly Open Price is above Monthly Open Price or vice versa ), then usually this Pair is also in trending mode but ranging setup (#4) will confirm or reject it.
Disclaimer :
This is not a Holy grail. Please use this for short-term inter-day trading. And keep Take Profit as per 15-min ATR value in Pips. And keep Stop Loss outside 1-Hr ATR Line.
Leave your comments and feedback, so a cBOT can also be developed.
Hope it helps other too who want to know how many Pips a Pair has moved from its opening price.
Please rate it and leave a comment.
Thank you.
///S.Khan
(*Still Learning to TRADE )
////////////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////////////
/// ///
/// REV. DATE : TUE-08-MAR-16 ///
/// MADE BY : ///S.KHAN ///
/// CONTACT ON : skhan.projects@gmail.com ///
/// DESCRIPTION : ///
/// (*THIS INDICATOR WILL DISPLAY THE DAILY, ///
/// WEEKLY, MONTHLY PIPS AND 1/3RD ATR ///
/// VALUES ON THE CHART FOR EASY REFERENCE.*) ///
////////////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////////////
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
using System.Text;
namespace cAlgo
{
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class DisplayDWMPipsonCharts : Indicator
{
////////////////////////////////////////////////////////////////////////////////
/// USER INPUT ///
////////////////////////////////////////////////////////////////////////////////
[Parameter("1-HOUR ATR, Period ", DefaultValue = 6, MinValue = 1, MaxValue = 500)]
public int p_Period_0 { get; set; }
[Parameter("Daily ATR, Period ", DefaultValue = 5, MinValue = 1, MaxValue = 60)]
public int p_Period_1 { get; set; }
[Parameter("Weekly ATR, Period ", DefaultValue = 4, MinValue = 1, MaxValue = 30)]
public int p_Period_2 { get; set; }
[Parameter("Monthly ATR, Period ", DefaultValue = 3, MinValue = 1, MaxValue = 20)]
public int p_Period_3 { get; set; }
[Parameter("Display Position, 1-8", DefaultValue = 8, MinValue = 1, MaxValue = 8)]
public int p_Display_Text { get; set; }
[Parameter("Display Color", DefaultValue = "White")]
public string p_Display_Color { get; set; }
////////////////////////////////////////////////////////////////////////////////
/// GLOBAL VARIABLES ///
////////////////////////////////////////////////////////////////////////////////
private MarketSeries my_Series_Hourly;
private MarketSeries my_Series_Daily;
private MarketSeries my_Series_Weekly;
private MarketSeries my_Series_Monthly;
private AverageTrueRange ATR_Hourly;
private AverageTrueRange ATR_Daily;
private AverageTrueRange ATR_Weekly;
private AverageTrueRange ATR_Monthly;
private StaticPosition my_Position;
double H1_Open, H1_Close, H1_Pips, D_Open, D_Close, Daily_Pips, W_Open, Weekly_Pips, M_Open, Monthly_Pips;
double Hourly_ATR, Daily_ATR, Weekly_ATR, Monthly_ATR;
double H1_Diff_1, H1_Diff_2, D_Diff_1, D_Diff_2, W_Diff_1, W_Diff_2, M_Diff_1, M_Diff_2;
StringBuilder Display_TEXT;
////////////////////////////////////////////////////////////////////////////////
/// INITIALIZE ///
////////////////////////////////////////////////////////////////////////////////
protected override void Initialize()
{
try
{
my_Series_Hourly = MarketData.GetSeries(Symbol, TimeFrame.Hour);
my_Series_Daily = MarketData.GetSeries(Symbol, TimeFrame.Daily);
my_Series_Weekly = MarketData.GetSeries(Symbol, TimeFrame.Weekly);
my_Series_Monthly = MarketData.GetSeries(Symbol, TimeFrame.Monthly);
ATR_Hourly = Indicators.AverageTrueRange(MarketData.GetSeries(TimeFrame.Hour), p_Period_0, MovingAverageType.Simple);
ATR_Daily = Indicators.AverageTrueRange(MarketData.GetSeries(TimeFrame.Daily), p_Period_1, MovingAverageType.Simple);
ATR_Weekly = Indicators.AverageTrueRange(MarketData.GetSeries(TimeFrame.Weekly), p_Period_2, MovingAverageType.Simple);
ATR_Monthly = Indicators.AverageTrueRange(MarketData.GetSeries(TimeFrame.Monthly), p_Period_3, MovingAverageType.Simple);
} catch
{
}
//FOR DISPLAY POSITION OF THE DISPLAY TEXT
switch (p_Display_Text)
{
case 1:
my_Position = StaticPosition.TopLeft;
break;
case 2:
my_Position = StaticPosition.TopCenter;
break;
case 3:
my_Position = StaticPosition.TopRight;
break;
case 4:
my_Position = StaticPosition.Left;
break;
case 5:
my_Position = StaticPosition.Center;
break;
case 6:
my_Position = StaticPosition.Right;
break;
case 7:
my_Position = StaticPosition.BottomLeft;
break;
case 8:
my_Position = StaticPosition.BottomCenter;
break;
default:
my_Position = StaticPosition.BottomRight;
break;
}
}
//END METHOD INITIALIZE
////////////////////////////////////////////////////////////////////////////////
/// CALCULATE ///
////////////////////////////////////////////////////////////////////////////////
public override void Calculate(int index)
{
Display_TEXT = new StringBuilder();
// During high volatility display warning to user
Colors my_Color = (Colors)Enum.Parse(typeof(Colors), p_Display_Color, true);
// 1-HOUR CLOSE VALUE
H1_Close = my_Series_Hourly.Close.LastValue;
// DAILY PIPS
H1_Open = my_Series_Hourly.Open.LastValue;
H1_Pips = (H1_Close - H1_Open) / Symbol.PipSize;
// DAILY CLOSE VALUE
D_Close = my_Series_Daily.Close.LastValue;
// DAILY PIPS
D_Open = my_Series_Daily.Open.LastValue;
Daily_Pips = (D_Close - D_Open) / Symbol.PipSize;
// PREVIOUS WEEK AND MONTH
// WEEKLY PIPS
W_Open = my_Series_Weekly.Open.LastValue;
//W_Open = my_Series_Weekly.Open.Last(index - 1);
Weekly_Pips = (D_Close - W_Open) / Symbol.PipSize;
// MONTHLY PIPS
M_Open = my_Series_Monthly.Open.LastValue;
//M_Open = my_Series_Monthly.Open.Last(index - 1);
Monthly_Pips = (D_Close - M_Open) / Symbol.PipSize;
// 1/3rd ATR VALUE : HOURLY, DAILY, WEEKLY, MONTHLY
Hourly_ATR = (ATR_Hourly.Result.LastValue / Symbol.PipSize) / 1;
Daily_ATR = (ATR_Daily.Result.LastValue / Symbol.PipSize) / 2;
Weekly_ATR = (ATR_Weekly.Result.LastValue / Symbol.PipSize) / 3;
Monthly_ATR = (ATR_Monthly.Result.LastValue / Symbol.PipSize) / 4;
// ---------------------------------------------------------------------------------------------------------
// DRAW LINES THROUGH : HOURLY, DAILY, WEEKLY, MONTHLY : ATR VALUES
// ---------------------------------------------------------------------------------------------------------
// GET PRICE : HOW FAR IS ATR LINE FROM HOURLY OPEN PRICE
H1_Diff_1 = H1_Open + (Hourly_ATR * Symbol.PipSize);
H1_Diff_2 = H1_Open - (Hourly_ATR * Symbol.PipSize);
// DRAW THE (1/1)ATR LINES, FAR FROM HOURLY OPEN PRICE
ChartObjects.DrawHorizontalLine("HUpperLine01", (H1_Diff_1), Colors.Snow, 1, LineStyle.Lines);
ChartObjects.DrawHorizontalLine("HUpperLine02", (H1_Diff_2), Colors.Snow, 1, LineStyle.Lines);
// DRAW TEXT ON THE ABOVE LINE
ChartObjects.DrawText("ATR0H1", "+H1 ATR", (index + 10), H1_Diff_1, VerticalAlignment.Top, HorizontalAlignment.Left, Colors.Snow);
ChartObjects.DrawText("ATR0H2", "-H1 ATR", (index + 10), H1_Diff_2, VerticalAlignment.Top, HorizontalAlignment.Left, Colors.Snow);
// ---------------------------------------------------------------------------------------------------------
// GET PRICE : HOW FAR IS ATR LINE FROM DAILY OPEN PRICE
D_Diff_1 = D_Open + (Daily_ATR * Symbol.PipSize);
D_Diff_2 = D_Open - (Daily_ATR * Symbol.PipSize);
// DRAW THE (1/2)ATR LINES, FAR FROM DAILY OPEN PRICE
ChartObjects.DrawHorizontalLine("DUpperLine01", (D_Diff_1), Colors.LimeGreen, 1, LineStyle.Lines);
ChartObjects.DrawHorizontalLine("DUpperLine02", (D_Diff_2), Colors.LimeGreen, 1, LineStyle.Lines);
// DRAW TEXT ON THE ABOVE LINE
ChartObjects.DrawText("ATR001", "+D-1/2 ATR", (index + 10), D_Diff_1, VerticalAlignment.Top, HorizontalAlignment.Left, Colors.LimeGreen);
ChartObjects.DrawText("ATR002", "-D-1/2 ATR", (index + 10), D_Diff_2, VerticalAlignment.Top, HorizontalAlignment.Left, Colors.LimeGreen);
// ---------------------------------------------------------------------------------------------------------
// GET PRICE : HOW FAR IS ATR LINE FROM WEEKLY OPEN PRICE
W_Diff_1 = W_Open + (Weekly_ATR * Symbol.PipSize);
W_Diff_2 = W_Open - (Weekly_ATR * Symbol.PipSize);
// DRAW THE (1/3)ATR LINES, FAR FROM WEEKLY OPEN PRICE
ChartObjects.DrawHorizontalLine("WUpperLine01", (W_Diff_1), Colors.DarkOrange, 1, LineStyle.Lines);
ChartObjects.DrawHorizontalLine("WUpperLine02", (W_Diff_2), Colors.DarkOrange, 1, LineStyle.Lines);
// DRAW TEXT ON THE ABOVE LINE
ChartObjects.DrawText("ATR003", "+W-1/3 ATR", (index + 10), W_Diff_1, VerticalAlignment.Top, HorizontalAlignment.Left, Colors.DarkOrange);
ChartObjects.DrawText("ATR004", "-W-1/3 ATR", (index + 10), W_Diff_2, VerticalAlignment.Top, HorizontalAlignment.Left, Colors.DarkOrange);
// ---------------------------------------------------------------------------------------------------------
// GET PRICE : HOW FAR IS ATR LINE FROM MONTHLY OPEN PRICE
M_Diff_1 = M_Open + (Monthly_ATR * Symbol.PipSize);
M_Diff_2 = M_Open - (Monthly_ATR * Symbol.PipSize);
// DRAW THE (1/4)ATR LINES, FAR FROM WEEKLY OPEN PRICE
ChartObjects.DrawHorizontalLine("MUpperLine01", (M_Diff_1), Colors.Yellow, 1, LineStyle.Lines);
ChartObjects.DrawHorizontalLine("MUpperLine02", (M_Diff_2), Colors.Yellow, 1, LineStyle.Lines);
// DRAW TEXT ON THE ABOVE LINE
ChartObjects.DrawText("ATR005", "+M-1/4 ATR", (index + 10), M_Diff_1, VerticalAlignment.Top, HorizontalAlignment.Left, Colors.Yellow);
ChartObjects.DrawText("ATR006", "-M-1/4 ATR", (index + 10), M_Diff_2, VerticalAlignment.Top, HorizontalAlignment.Left, Colors.Yellow);
// ---------------------------------------------------------------------------------------------------------
// ----------------------------------------------------------------------------------------------------------
// DRAW : DAILY, WEEKLY, MONTHLY LINES THROUGH OPEN PRICES
// ----------------------------------------------------------------------------------------------------------
// DRAW LINE ON OPEN PRICES : DAILY, WEEKLY, MONTHLY
//ChartObjects.DrawHorizontalLine("OpenLine00", (H1_Open), Colors.Snow, 2, LineStyle.Solid);
//ChartObjects.DrawHorizontalLine("OpenLine01", (D_Open), Colors.LimeGreen, 2, LineStyle.Solid);
//ChartObjects.DrawHorizontalLine("OpenLine02", (W_Open), Colors.DarkOrange, 2, LineStyle.Solid);
//ChartObjects.DrawHorizontalLine("OpenLine03", (M_Open), Colors.Yellow, 2, LineStyle.Solid);
// DRAW TEXT ON THE ABOVE LINE
ChartObjects.DrawText("dr000", "Hourly Open", (index + 50), H1_Open, VerticalAlignment.Top, HorizontalAlignment.Left, Colors.Snow);
ChartObjects.DrawText("dr001", "Daily Open", (index + 50), D_Open, VerticalAlignment.Top, HorizontalAlignment.Left, Colors.LimeGreen);
ChartObjects.DrawText("dr002", "Weekly Open", (index + 50), W_Open, VerticalAlignment.Top, HorizontalAlignment.Left, Colors.DarkOrange);
ChartObjects.DrawText("dr003", "Monthly Open", (index + 50), M_Open, VerticalAlignment.Top, HorizontalAlignment.Left, Colors.Yellow);
// ----------------------------------------------------------------------------------------------------------
// ----------------------------------------------------------------------------------------------------------
// DISPLAY : PIPS AND ATR VALUES ON THE CHART
// ----------------------------------------------------------------------------------------------------------
// CONVERT TO STRING
Display_TEXT.Append(" TIME FRAME : Pips, (1/X) ATR");
Display_TEXT.AppendLine();
// HOURLY
Display_TEXT.Append("H, (1/1) : ");
Display_TEXT.AppendFormat("{0:N0}", H1_Pips);
Display_TEXT.Append(", ");
Display_TEXT.AppendFormat("{0:N0}", Hourly_ATR);
Display_TEXT.AppendLine();
// DAILY
Display_TEXT.Append("D, (1/2) : ");
Display_TEXT.AppendFormat("{0:N0}", Daily_Pips);
Display_TEXT.Append(", ");
Display_TEXT.AppendFormat("{0:N0}", Daily_ATR);
Display_TEXT.AppendLine();
// WEEKLY
Display_TEXT.Append("W, (1/3) : ");
Display_TEXT.AppendFormat("{0:N0}", Weekly_Pips);
Display_TEXT.Append(", ");
Display_TEXT.AppendFormat("{0:N0}", Weekly_ATR);
Display_TEXT.AppendLine();
// MONTHLY
Display_TEXT.Append("M, (1/4) : ");
Display_TEXT.AppendFormat("{0:N0}", Monthly_Pips);
Display_TEXT.Append(", ");
Display_TEXT.AppendFormat("{0:N0}", Monthly_ATR);
Display_TEXT.AppendLine();
//DISPLAY ON CHART
ChartObjects.DrawText("my001", Display_TEXT.ToString(), my_Position, my_Color);
// ----------------------------------------------------------------------------------------------------------
}
//END METHOD CALCULATE
}
//END class DisplayDWMPipsonCharts
}
//END namespace cAlgo
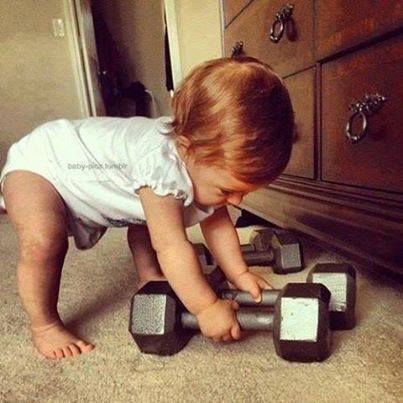
GoldnOil750
Joined on 07.04.2015
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: 1SK- Display DWM Pips on Charts.algo
- Rating: 5
- Installs: 5347
thank you