Description
DSS Bressert - Double Smoothed Stochastic Indicator by Walter Bressert
Its calculation algorithm is very similar to that of stochastic indicator. The value above 80 signifies the overbuying, the value below 20 signifies the overselling.
Description:
One after the other, William Blau and Walter Bressert each presented a version of the Double Smoothed Stochastics. Two exponentially smoothed MAs are used to even out the input values (H, L and C), in a similar way to the well-known stochastic formula.
Calculation:
Calculation of the DSS indicator according to Bressert is similar to stochastics.
1.) The numerator: first the difference between the current close and the period low is formed. The denominator: here the difference between the period high minus the period low is calculated. Now the quotient of numerator and denominator is calculated, exponentially smoothed and then multiplied by 100.
2.) The method is analogous to 1.) with the distinction that now the prices of the newly calculated price series of 1.) is used.
Parameters:
The adjustable period length can be chosen from 2 to 500. The most common settings will have a period length ranging from 5 to 30. In addition, the indicator can be smoothed in the interval from 1 to 50. Meaningful smoothing values lie in the short-term range.
Interpretation:
The application of the DSS is comparable with that of the stochastic method. Accordingly, values above 70 or 80 must be regarded as overbought and values below 20 or 30 as oversold. A rise of the DSS above its center line should be viewed as bullish, and a fall of the DSS below its center line as bearish.
Github: GitHub - Doustzadeh/cTrader-Indicator
using cAlgo.API;
using cAlgo.API.Indicators;
namespace cAlgo
{
[Levels(0, 20, 80, 100)]
[Indicator(IsOverlay = false, ScalePrecision = 2, AccessRights = AccessRights.None)]
public class DSSBressert : Indicator
{
[Parameter("Stochastic Periods", DefaultValue = 13)]
public int StochasticPeriods { get; set; }
[Parameter("EMA Periods", DefaultValue = 8)]
public int EmaPeriods { get; set; }
[Parameter("WMA Periods", DefaultValue = 5)]
public int WmaPeriods { get; set; }
[Output("DSS", LineColor = "White", Thickness = 1)]
public IndicatorDataSeries DSS { get; set; }
[Output("DSS Up", LineColor = "DodgerBlue", PlotType = PlotType.Points, Thickness = 5)]
public IndicatorDataSeries DssUp { get; set; }
[Output("DSS Down", LineColor = "Red", PlotType = PlotType.Points, Thickness = 5)]
public IndicatorDataSeries DssDown { get; set; }
[Output("WMA", LineColor = "Gold", Thickness = 1)]
public IndicatorDataSeries WmaResult { get; set; }
private double Ln = 0;
private double Hn = 0;
private double LXn = 0;
private double HXn = 0;
private double alpha = 0;
private IndicatorDataSeries mit;
private WeightedMovingAverage WMA;
protected override void Initialize()
{
mit = CreateDataSeries();
alpha = 2.0 / (1.0 + EmaPeriods);
WMA = Indicators.WeightedMovingAverage(DSS, WmaPeriods);
}
public override void Calculate(int index)
{
if (double.IsNaN(mit[index - 1]))
{
mit[index - 1] = 0;
}
if (double.IsNaN(DSS[index - 1]))
{
DSS[index - 1] = 0;
}
Ln = Bars.LowPrices.Minimum(StochasticPeriods);
Hn = Bars.HighPrices.Maximum(StochasticPeriods);
mit[index] = mit[index - 1] + alpha * ((((Bars.ClosePrices[index] - Ln) / (Hn - Ln)) * 100) - mit[index - 1]);
LXn = mit.Minimum(StochasticPeriods);
HXn = mit.Maximum(StochasticPeriods);
DSS[index] = DSS[index - 1] + alpha * ((((mit[index] - LXn) / (HXn - LXn)) * 100) - DSS[index - 1]);
if (DSS[index] > DSS[index - 1])
{
DssUp[index] = DSS[index];
DssDown[index] = double.NaN;
}
if (DSS[index] < DSS[index - 1])
{
DssDown[index] = DSS[index];
DssUp[index] = double.NaN;
}
WmaResult[index] = WMA.Result[index];
}
}
}
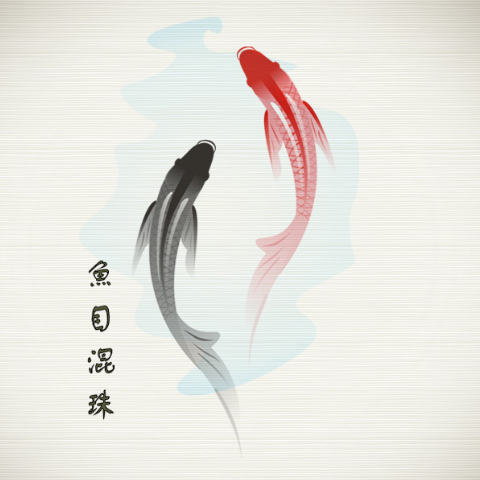
Doustzadeh
Joined on 20.03.2016
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: DSS Bressert.algo
- Rating: 0
- Installs: 4043
Comments
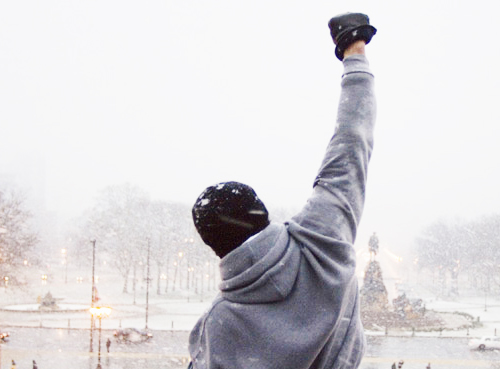
Hi,
Just want to say thank you for coding the DSS Bressert indicator, i'm using it a lot on Metatrader 4.
Now it is available on CTrader which is amazing http://fxpro.ctrader.com/images/screens/vrMTn.png
Regards,
matfx
Many thanks!