Description
- Current open markets, updated in real time.
- Time bar progresses from left to right.
- Hours are optimized to local time.
Updates:
- 19/03/2016 - Released.
- 26/03/2016 - Updated strings.
- 30/03/2016 - Fixed offset issue.
- 01/04/2016 - Fixed opening times.
Screenshot:
Make a Donation
- If you like my work and effort then please consider to make a kind donation thru PayPal or any Credit Card at the top right corner.
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class MarketHours : Indicator
{
private string[] hours = new string[24];
private TimeSpan offset = DateTime.Now.Subtract(DateTime.UtcNow);
private int sync = -1;
private Colors color;
protected override void Initialize()
{
SetHours();
Timer.Start(TimeSpan.FromMilliseconds(1));
}
protected override void OnTimer()
{
DateTime utc = DateTime.UtcNow;
int vLine = 240 - (utc.AddHours(3).Hour * 10 + utc.Minute / 6);
for (int i = 0; i <= 4; i++)
{
int shift = 0;
for (int h = 1; h <= 24; h++)
{
if (i == 0)
{
color = Colors.DimGray;
if (shift + 10 >= vLine && vLine > shift)
color = Colors.LimeGreen;
}
else
{
color = Colors.Gainsboro;
if (i == 1)
if (240 > shift && shift >= 150)
if (240 >= vLine && vLine > 150)
color = Colors.Orange;
else
color = Colors.BurlyWood;
if (i == 2)
if (220 > shift && shift >= 130)
if (220 >= vLine && vLine > 130)
color = Colors.YellowGreen;
else
color = Colors.LightGreen;
if (i == 3)
if (140 > shift && shift >= 50)
if (140 >= vLine && vLine > 50)
color = Colors.DodgerBlue;
else
color = Colors.LightBlue;
if (i == 4)
if (90 > shift && shift >= 0)
if (90 >= vLine && vLine > 0)
color = Colors.DeepPink;
else
color = Colors.LightPink;
}
ChartObjects.DrawText("background" + h + i, y(i) + (char)9609 + x(shift + 5), StaticPosition.TopRight, color);
int hour = (21 - h + offset.Hours);
hour = hour >= 24 ? hour - 24 : hour < 0 ? hour + 24 : hour;
color = Colors.White;
ChartObjects.DrawText("hour" + h, hours[hour] + x(shift + 6), StaticPosition.TopRight, color);
shift += 10;
}
}
string sydney = "" + (char)42801 + (char)655 + (char)7429 + (char)628 + (char)7431 + (char)655;
string tokyo = "" + (char)7451 + (char)7439 + (char)7435 + (char)655 + (char)7439;
string london = "" + (char)671 + (char)7439 + (char)628 + (char)7429 + (char)7439 + (char)628;
string newyork = "" + (char)628 + (char)7431 + (char)7457 + " " + (char)655 + (char)7439 + (char)640 + (char)7435;
ChartObjects.DrawText("Sydney", y(1) + sydney + x(182), StaticPosition.TopRight, color);
ChartObjects.DrawText("Tokyo", y(2) + tokyo + x(165), StaticPosition.TopRight, color);
ChartObjects.DrawText("London", y(3) + london + x(80), StaticPosition.TopRight, color);
ChartObjects.DrawText("New York", y(4) + newyork + x(26), StaticPosition.TopRight, color);
color = Colors.LimeGreen;
for (int i = 1; i <= 4; i++)
{
ChartObjects.DrawText("vLine" + i, y(i) + (char)9474 + x(vLine), StaticPosition.TopRight, color);
}
if (sync < 1)
{
Timer.Stop();
TimeSpan interval;
if (sync < 0)
{
var nextUpdate = new DateTime(utc.Year, utc.Month, utc.Day, utc.Hour, (utc.Minute / 6 + 1) * 6, 2);
var difference = nextUpdate.Subtract(utc);
interval = difference;
Timer.Start(interval);
sync = 0;
}
else
{
interval = TimeSpan.FromMinutes(6);
Timer.Start(interval);
sync = 1;
}
}
}
public override void Calculate(int index)
{
// Do nothing.
}
private string x(int count)
{
return new string((char)8202, count);
}
private string y(int count)
{
return new string('\v', count);
}
private void SetHours()
{
for (int i = 0; i <= 23; i++)
{
if (i <= 9)
{
hours[i] = "" + (char)(8320 + i) + x(2);
}
else if (i <= 19)
{
hours[i] = "" + (char)8321 + (char)(8310 + i);
}
else
{
hours[i] = "" + (char)8322 + (char)(8300 + i);
}
}
}
}
}
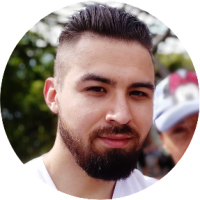
Jiri
Joined on 31.08.2015
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: MarketHours.algo
- Rating: 4.17
- Installs: 4259
- Modified: 13/10/2021 09:55
Comments
hi
how can i shift the timezone half an hour forward ?
by the way please manipulate the code that refresh itself every second. i do it manually that it show me the exact time or session. if it possible
thanks.
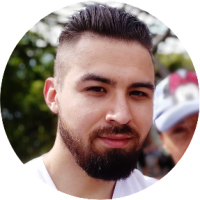
Does Sydney open at 9pm utc? If so, I had it wrong in the code. Fixed version uploaded.
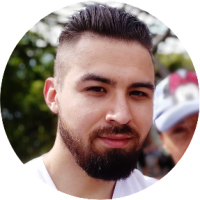
Does the vertical line showing your local time correctly?
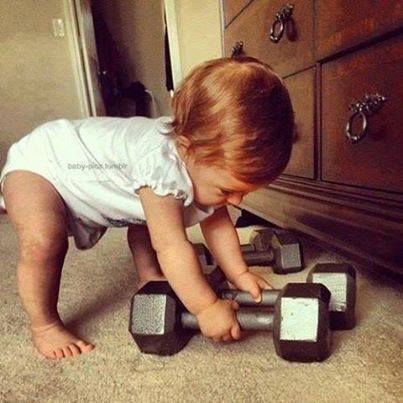
PERFECT !!! working just fine. haven't checked its accuracy...... (*just noticed that NEW YORK will open at 18:00 hours as per my local time, but actually NEW YORK will open around 17:00 hrs. Can you check that or any user-input where a user can add +1 or -1 to get the correct time, if there is error.
thank you !
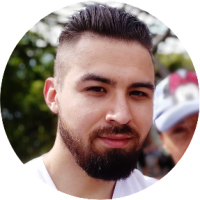
There was a bug in offset, which might caused the issue. Please, try it now and let me know if it works.
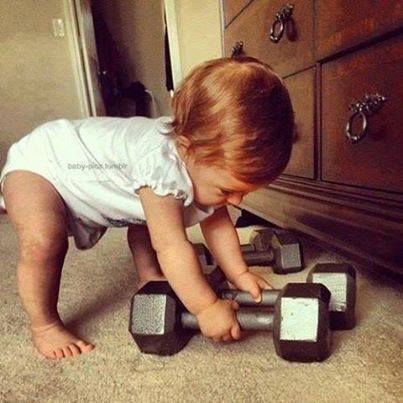
Hi,
it compiled but it is not showing the table as shown in your chart screen shot. It only display 3 very small grey color boxex, with blank, 1, 2 written on it, on the top right corner of the Chart. (*tried with RoboForex and FxPro ctrader platform. same result.
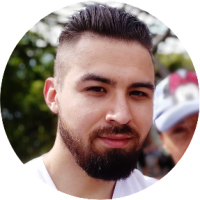
I cleaned up the code. Try it now.
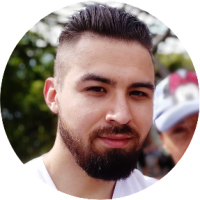
Did you download the algo or copied the source code above? The code above is full of messed up characters.
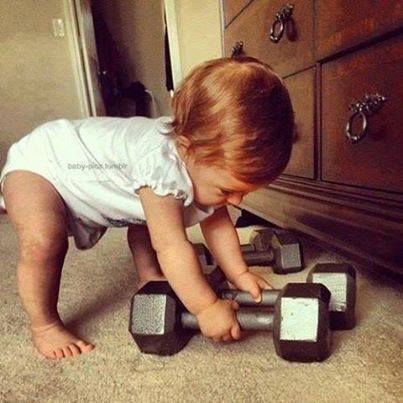
It is not compiling. Giving Error on 84 onwards.
how to change the font size of the session hours ?