Description
Simple example that shows how to send a message to a Telegram channel. Requires a Telegram API Key and a ChannelId.
To get a API key, follow these directions.
https://core.telegram.org/bots#botfather
The code in OnStart tries to find a ChannelId based on recent interactions with the robot. Once you know a particular ChannelId, set it as a parameter. It i's a little strange, but I could not figure out any other way to get a channelid.
using System;
using System.Linq;
using System.Net;
using System.Text;
using System.IO;
using System.Text.RegularExpressions;
using System.Collections.Generic;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
namespace cAlgo
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.FullAccess)]
public class TelegramBotExample : Robot
{
[Parameter("Telegram Bot Key", DefaultValue = "__bot_key__")]
public string BOT_API_KEY { get; set; }
// if you know which channel you want to broadcast to, add it here
// otherwise the bot looks at the people and channels its' interacted with and
// sends messages to everyone
[Parameter("ChannelId", DefaultValue = "")]
public string ChannelId { get; set; }
List<string> _telegramChannels = new List<string>();
protected override void OnStart()
{
// If you didn't set a channel, then find channels by looking at the updates
if (string.IsNullOrEmpty(ChannelId))
{
_telegramChannels = ParseChannels(GetBotUpdates());
}
else
{
_telegramChannels.Add(ChannelId);
}
SendMessageToAllChannels("Hellow world.. ");
}
protected override void OnTick()
{
// Put your core logic here
}
protected override void OnBar()
{
// Put your core logic here
int idx = this.MarketSeries.Close.Count - 2;
if (this.MarketSeries.Close[idx] > this.MarketSeries.Open[idx])
{
SendMessageToAllChannels("Up Candle " + this.MarketSeries.Close[idx] + " " + this.MarketSeries.Open[idx]);
}
else
{
SendMessageToAllChannels("Down Candle " + this.MarketSeries.Close[idx] + " " + this.MarketSeries.Open[idx]);
}
}
protected override void OnStop()
{
// Put your deinitialization logic here
}
// Parses messages for bot and determines which channesl are listening
private List<string> ParseChannels(string jsonData)
{
var matches = new Regex("\"id\"\\:(\\d+)").Matches(jsonData);
List<string> channels = new List<string>();
if (matches.Count > 0)
{
foreach (Match m in matches)
{
if (!channels.Contains(m.Groups[1].Value))
{
channels.Add(m.Groups[1].Value);
}
}
}
foreach (var v in channels)
{
Print("DEBUG: Found Channel {0} ", v);
}
return channels;
}
protected int updateOffset = -1;
private string GetBotUpdates()
{
Dictionary<string, string> values = new Dictionary<string, string>();
if (updateOffset > -1)
{
values.Add("offset", (updateOffset++).ToString());
}
var jsonData = MakeTelegramRequest(BOT_API_KEY, "getUpdates", values);
var matches = new Regex("\"message_id\"\\:(\\d+)").Matches(jsonData);
if (matches.Count > 0)
{
foreach (Match m in matches)
{
int msg_id = -1;
int.TryParse(m.Groups[1].Value, out msg_id);
if (msg_id > updateOffset)
{
updateOffset = msg_id;
}
}
}
return jsonData;
}
private void SendMessageToAllChannels(string message)
{
foreach (var c in _telegramChannels)
{
SendMessageToChannel(c, message);
}
}
private string SendMessageToChannel(string chat_id, string message)
{
var values = new Dictionary<string, string>
{
{
"chat_id",
chat_id
},
{
"text",
message
}
};
return MakeTelegramRequest(BOT_API_KEY, "sendMessage", values);
}
private string MakeTelegramRequest(string api_key, string method, Dictionary<string, string> values)
{
string TELEGRAM_CALL_URI = string.Format("https://api.telegram.org/bot{0}/{1}", api_key, method);
var request = WebRequest.Create(TELEGRAM_CALL_URI);
request.ContentType = "application/x-www-form-urlencoded";
request.Method = "POST";
StringBuilder data = new StringBuilder();
foreach (var d in values)
{
data.Append(string.Format("{0}={1}&", d.Key, d.Value));
}
byte[] byteArray = Encoding.UTF8.GetBytes(data.ToString());
request.ContentLength = byteArray.Length;
Stream dataStream = request.GetRequestStream();
dataStream.Write(byteArray, 0, byteArray.Length);
dataStream.Close();
WebResponse response = request.GetResponse();
Print("DEBUG {0}", ((HttpWebResponse)response).StatusDescription);
dataStream = response.GetResponseStream();
StreamReader reader = new StreamReader(dataStream);
string outStr = reader.ReadToEnd();
Print("DEBUG {0}", outStr);
reader.Close();
return outStr;
}
}
}
pennyfx
Joined on 27.09.2012
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: New cBot.algo
- Rating: 0
- Installs: 2744
Comments
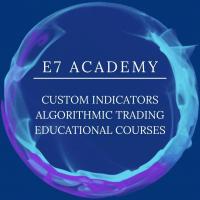
Hi guys, how did you solve the bot instantly stopping, after starting it? Any help will be much appreciated. Thanks.
Thanks a lot
Fix detail here
https://ctrader.com/forum/third-party-products/22853
My OnStart() now as follows
protected override void OnStart()
{
ServicePointManager.Expect100Continue = true;
ServicePointManager.SecurityProtocol = SecurityProtocolType.Tls12;
// If you didn't set a channel, then find channels by looking at the updates
if (string.IsNullOrEmpty(ChannelId))
{
_telegramChannels = ParseChannels(GetBotUpdates());
}
else
{
_telegramChannels.Add(ChannelId);
}
SendMessageToAllChannels("Hellow world.. ");
}
I have having the same issue.
Crashed in OnStart with WebException: The request was aborted: Could not create SSL/TLS secure channel.
When I run this is Visual studio with dubug it does not trap any error
Hi. Hope you can help out with the issue since the latest update this Feb-2020. The code is having an error "The request was aborted: Could not create SSL/TLS secure channel." I have been using this code since last year for sending alerts to my telegram bot, but now the ctrader bot is crashing. Do you have a workaround? Appreciate your help. Cheers!
This work not any more with cTrader 3.5 (for me). Do you have another code for telegram in cBot to use?
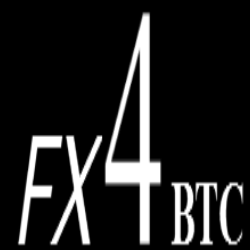
maybe this will help you out. Best luck - http://fx4btc.com/2016/05/telegram-bot-tradingview-charts/
got it to work, thanks :)
I figured out the issue with the robot instantly stopping. I am now having an issue with receiving messages. Its not getting sent to my channel.
I entered my api key and channel ID and started the robot but it instantly stopped after. Any idea why?
Thanks
@Gwave
Relplace OnStart Function with this one below
protected override void OnStart()
{
ServicePointManager.Expect100Continue = true;
ServicePointManager.SecurityProtocol = SecurityProtocolType.Tls12;
// If you didn't set a channel, then find channels by looking at the updates
if (string.IsNullOrEmpty(ChannelId))
{
_telegramChannels = ParseChannels(GetBotUpdates());
}
else
{
_telegramChannels.Add(ChannelId);
}
SendMessageToAllChannels("Hellow world.. ");
}
if u r using visual studio , you get error line under Tls12, just ignore it as it works when u build in CTrader.
It will fix the code for you