Description
Based on the strategy of The difference between 2 RSIs(5,14)
When the Fast RSI Crosses the Slow RSI , you may scalp in that direction.
The ADX component of DMS is added with adjustable threshold (usually 40 for Stocks , Forex 20 or something)
Use at your own Risk
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Indicator("DoubleRSI", ScalePrecision = 0, IsOverlay = false, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class DoubleRSI : Indicator
{
private RelativeStrengthIndex _rsi;
private RelativeStrengthIndex _rsi2;
private DirectionalMovementSystem _dms;
// ADX Histogram
[Parameter(DefaultValue = 14)]
public int ADX_Periods { get; set; }
[Parameter(DefaultValue = 20)]
public int ADX_Threshold { get; set; }
[Parameter()]
public DataSeries Source { get; set; }
[Parameter(DefaultValue = 5)]
public int FastRSI { get; set; }
[Parameter(DefaultValue = 10)]
public int SlowRSI { get; set; }
//Colors
[Output("ADX", Color = Colors.Black, PlotType = PlotType.Histogram, Thickness = 3)]
public IndicatorDataSeries ADX { get; set; }
[Output("Thrust", Color = Colors.DimGray, PlotType = PlotType.Histogram, Thickness = 3)]
public IndicatorDataSeries ADX_Thrust { get; set; }
[Output("FastRSI", Color = Colors.LightGreen)]
public IndicatorDataSeries Result { get; set; }
[Output("SlowRSI", Color = Colors.Blue)]
public IndicatorDataSeries Result2 { get; set; }
protected override void Initialize()
{
_dms = Indicators.DirectionalMovementSystem(ADX_Periods);
_rsi = Indicators.RelativeStrengthIndex(Source, FastRSI);
_rsi2 = Indicators.RelativeStrengthIndex(Source, SlowRSI);
}
public override void Calculate(int index)
{
if ((int)_dms.ADX.LastValue >= 0 && ((int)_dms.ADX.LastValue < ADX_Threshold))
{
ADX[index] = (int)_dms.ADX.LastValue;
}
else if ((int)_dms.ADX.LastValue >= ADX_Threshold)
{
ADX_Thrust[index] = (int)_dms.ADX.LastValue;
}
Result[index] = (int)_rsi.Result[index];
Result2[index] = (int)_rsi2.Result[index];
}
}
}
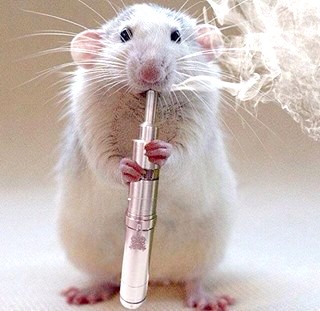
cyfer
Joined on 27.09.2015
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Cyf_Double RSI.algo
- Rating: 0
- Installs: 3937
- Modified: 13/10/2021 09:54
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.