Description
- Linear regression channel based.
- Overlaying channels indicate respected channel.
- Additional description will be added in the future.
Notes
- It might be useful for those who like to trade channels but are lazy to draw them manually - just like me. :D
- Any feedback or ideas appreciated.
Screenshot
Make a Donation
- If you like my work and effort then please consider to make a kind donation thru PayPal or any Credit Card at the top right corner.
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
namespace cAlgo.Indicators
{
[Indicator(IsOverlay = true, AutoRescale = false, AccessRights = AccessRights.None)]
public class ChannelSketcher : Indicator
{
[Parameter()]
public DataSeries Source { get; set; }
[Parameter(DefaultValue = 20, MinValue = 1)]
public int Period { get; set; }
[Parameter(DefaultValue = 0, MinValue = 0)]
public int Extend { get; set; }
[Parameter(DefaultValue = 0.1, MinValue = 0.01, Step = 0.01)]
public double Thickness { get; set; }
[Output("Diff", PlotType = PlotType.Points)]
public IndicatorDataSeries Diff { get; set; }
protected override void Initialize()
{
}
public override void Calculate(int index)
{
// Starting and ending bars
int x1 = Source.Count - Period;
int x2 = Source.Count - 1;
// Linear regression parameters
double sumX = 0;
double sumX2 = 0;
double sumY = 0;
double sumXY = 0;
for (int count = x1; count <= x2; count++)
{
sumX += count;
sumX2 += count * count;
sumY += Source[count];
sumXY += Source[count] * count;
}
double divisor = (Period * sumX2 - sumX * sumX);
double slope = (Period * sumXY - sumX * sumY) / divisor;
double intercept = (sumY - slope * sumX) / Period;
// Distance to the regression line
double deviation = 0;
for (int count = x1; count <= x2; count++)
{
double regression = slope * count + intercept;
deviation = Math.Max(Math.Abs(Source[count] - regression), deviation);
}
// Linear regression channel
x2 += Extend;
double y1 = slope * x1 + intercept;
double y2 = slope * x2 + intercept;
var color = y1 < y2 ? Colors.LimeGreen : Colors.Red;
ChartObjects.DrawLine("Upper" + index, x1, y1 + deviation, x2, y2 + deviation, color, Thickness, LineStyle.Solid);
ChartObjects.DrawLine("Lower" + index, x1, y1 - deviation, x2, y2 - deviation, color, Thickness, LineStyle.Solid);
Diff[index] = y2 - y1;
}
}
}
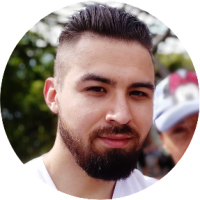
Jiri
Joined on 31.08.2015
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: ChannelSketcher.algo
- Rating: 4.17
- Installs: 3286
Comments
Download is broken. Can someone please upload again or check whats wrong?
Kind Regards
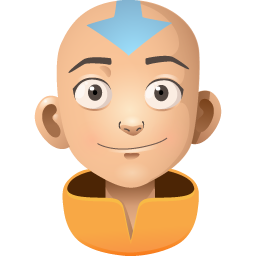
nevermind all good.
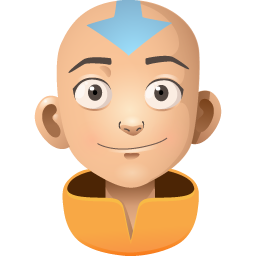
Smart approach. Thank you.
I replaced the Black color with White but it's not showing on the chart. Any suggistion?
can you add slope value for the channel and alert that can be selecteld on slope value input
great work! seems very useful. thank you for sharing
Very nice idea. Thank you for sharing.
Absolutely love this indicator.
I have an idea and am wondering what you think...as the angle of the channels change and become flatter, you could change the channel colour to amber or orange, or cycle through a range of colours that would indicate when a trend is weakening?
Is that something that CTrader is capable of? Forgive me, I am no programmer.
Kudos to you for this, it is helping me in my trades...thank you.
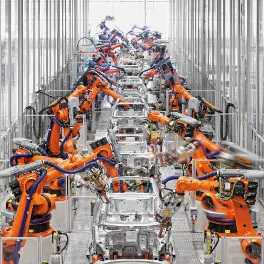
Very creative! You could print the chart on a canvas, and make an exhibition... seriously.
Got it manually to work. But There is no more 0.1 thickness option. Or ist there? I can only choose 1, and thats no like in the picture above. With thickness 1 it's terrible, can someone help?