Description
I decided to make a simple indicator to see how wicks behave, some cool patterns seem to emerge.
You can see for example Upwicks tend to be bigger in downtrends and viceversa.
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = false, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class AverageWick : Indicator
{
[Parameter("Period", DefaultValue = 10)]
public int _Period { get; set; }
[Output("UpWick", Color = Colors.Blue)]
public IndicatorDataSeries _UpWick { get; set; }
[Output("DownWick", Color = Colors.Red)]
public IndicatorDataSeries _DownWick { get; set; }
double _upresult, _downresult;
protected override void Initialize()
{
}
public override void Calculate(int index)
{
if (MarketSeries.Open.LastValue < MarketSeries.Close.LastValue)
{
for (int i = _Period; i >= 1; i--)
{
_upresult += MarketSeries.High.Last(i) - MarketSeries.Close.Last(i);
}
_UpWick[index] = (_upresult / _Period) / Symbol.PipSize;
_upresult = 0;
}
else if (MarketSeries.Open.LastValue > MarketSeries.Close.LastValue)
{
for (int i = _Period; i >= 1; i--)
{
_downresult += MarketSeries.Close.Last(i) - MarketSeries.Low.Last(i);
}
_DownWick[index] = (_downresult / _Period) / Symbol.PipSize;
_downresult = 0;
}
}
}
}
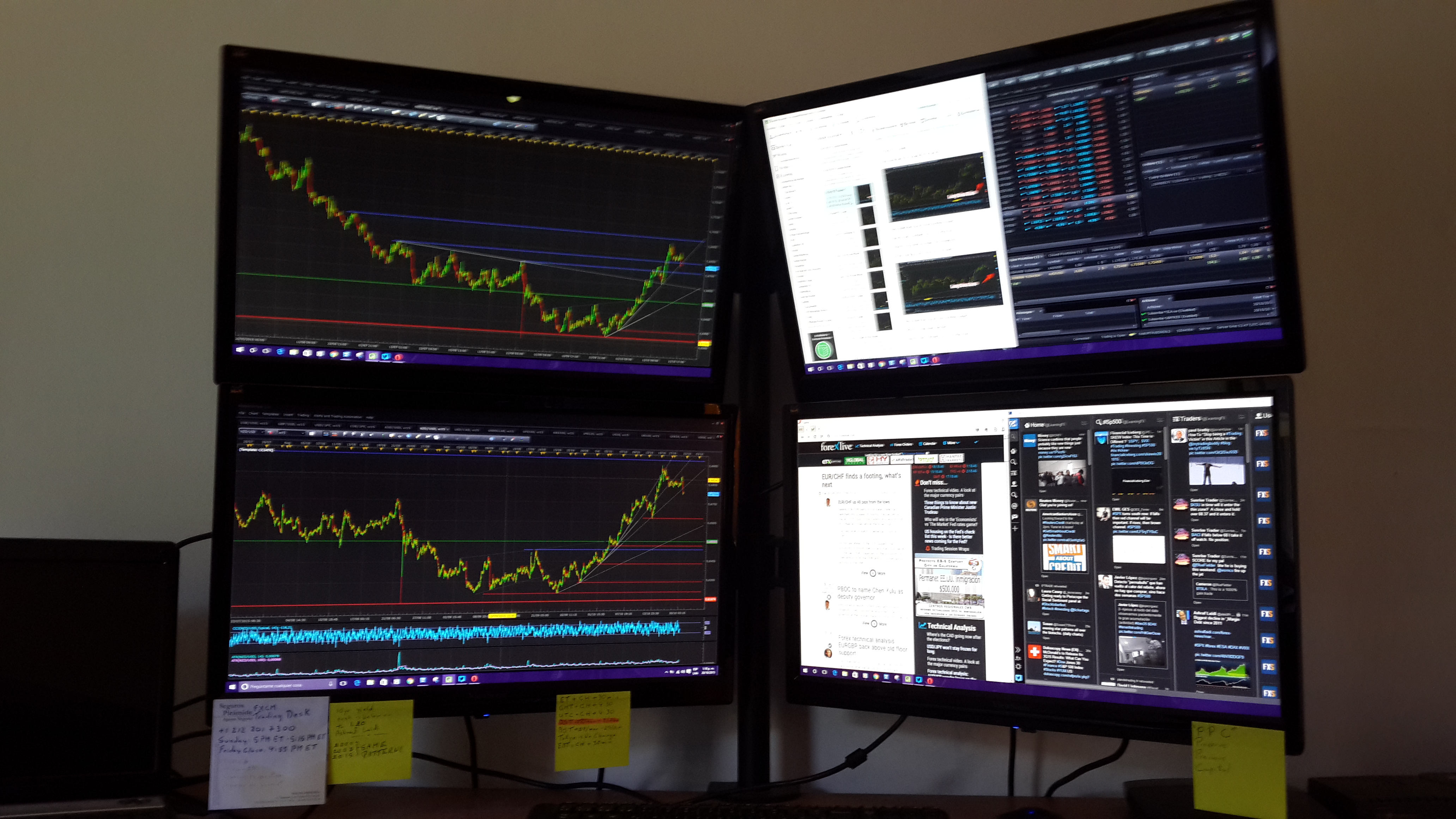
Waxy
Joined on 12.05.2015
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Average Wick.algo
- Rating: 5
- Installs: 2936
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
So what's the concept of how to use it?