Description
Fractals TF allows you to see higher TimeFrame fractals on low TimeFrame charts. For example, I like to use M5 fractals on M1 charts.
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class FractalsTF : Indicator
{
[Parameter()]
public TimeFrame FractalTimeFrame { get; set; }
[Output("Top Fractal", Color = Colors.Blue, PlotType = PlotType.Points, Thickness = 5)]
public IndicatorDataSeries TopFractal { get; set; }
[Output("Bottom Fractal", Color = Colors.Blue, PlotType = PlotType.Points, Thickness = 5)]
public IndicatorDataSeries BottomFractal { get; set; }
protected MarketSeries _source;
private int _lookback = 3;
protected override void Initialize()
{
// Initialize and create nested indicators
_source = this.MarketData.GetSeries(FractalTimeFrame);
}
public override void Calculate(int index)
{
DrawUpFractal(index, _lookback, TopFractal);
DrawDownFractal(index, _lookback, BottomFractal);
}
protected void DrawUpFractal(int index, int period, IndicatorDataSeries series)
{
var sourceIdx = _source.OpenTime.GetIndexByTime(this.MarketSeries.OpenTime[index]);
int highIdx = -1;
double highPoint = double.MinValue;
int cnt = 0;
while (cnt < period)
{
int idx = sourceIdx - cnt;
if (_source.High[idx] > highPoint)
{
highPoint = _source.High[idx];
highIdx = idx;
}
cnt++;
}
if (_source.High[highIdx - 1] < highPoint && _source.High[highIdx + 1] < highPoint)
{
// We're scaling between timeframes, so we have to find the exact index
// of the price on the chart timeframe.
int outPutIdx = this.MarketSeries.OpenTime.GetIndexByTime(_source.OpenTime[highIdx]);
double currerntHigh = this.MarketSeries.High[outPutIdx];
while (currerntHigh != highPoint && outPutIdx < this.MarketSeries.High.Count)
{
currerntHigh = this.MarketSeries.High[++outPutIdx];
series[outPutIdx] = double.NaN;
}
series[outPutIdx] = highPoint;
}
}
protected void DrawDownFractal(int index, int period, IndicatorDataSeries series)
{
var sourceIdx = _source.OpenTime.GetIndexByTime(this.MarketSeries.OpenTime[index]);
int lowIdx = -1;
double lowPoint = double.MaxValue;
int cnt = 0;
while (cnt < period)
{
int idx = sourceIdx - cnt;
series[idx] = double.NaN;
if (_source.Low[idx] < lowPoint)
{
lowPoint = _source.Low[idx];
lowIdx = idx;
}
cnt++;
}
if (_source.Low[lowIdx - 1] > lowPoint && _source.Low[lowIdx + 1] > lowPoint)
{
// We're scaling between timeframes, so we have to find the exact index
// of the price on the chart timeframe.
int outPutIdx = this.MarketSeries.OpenTime.GetIndexByTime(_source.OpenTime[lowIdx]);
double currerntLow = this.MarketSeries.Low[outPutIdx];
while (currerntLow != lowPoint && outPutIdx < this.MarketSeries.Low.Count)
{
currerntLow = this.MarketSeries.Low[++outPutIdx];
series[outPutIdx] = double.NaN;
}
series[outPutIdx] = lowPoint;
}
}
}
}
PE
pennyfx
Joined on 27.09.2012
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: Fractals TF.algo
- Rating: 5
- Installs: 5536
- Modified: 13/10/2021 09:54
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
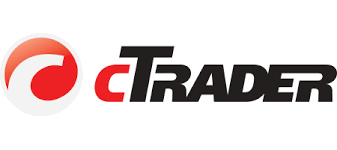
This doesnt work right fyi. It shows me a fractal exists when it doesnt.
on 15min using 1hr fractals it shows a fractal. switch to 1hr, no fractal.
DA
It is a very good and usefull indicator.
can you an alarm option for telegram and email add.
This will be really usefull.
Thanks.
Very good indicator, do you think it is possible to insert some lines that can show fractals from the past nowadays? and automatically as they are hit they update