Description
- The Fibonacci retracement is the potential retracement of a financial asset's original move in price. Fibonacci retracements use horizontal lines to indicate areas of support or resistance at the key Fibonacci levels before it continues in the original direction.
Updates
- 29/01/2016
- Released.
Inputs
- Days To Show - Defines how many days will be plotted.
- Shift (Hours) - Shifts levels by hours.
Screenshot
Make a Donation
- If you like my work and effort then please consider to make a kind donation thru PayPal or any Credit Card at the top right corner.
using System;
using cAlgo.API;
using cAlgo.API.Internals;
namespace cAlgo.Indicators
{
[Indicator(IsOverlay = true, AutoRescale = false, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class DailyFiboRetracement : Indicator
{
[Parameter("Days To Show", DefaultValue = 5)]
public int DaysToShow { get; set; }
[Parameter("Shift (Hours)", DefaultValue = 0)]
public int ShiftInHours { get; set; }
private MarketSeries daily;
protected override void Initialize()
{
daily = MarketData.GetSeries(TimeFrame.Daily);
}
public override void Calculate(int index)
{
for (int i = 0; i < DaysToShow; i++)
{
DateTime startOfDay = daily.OpenTime.Last(i).Date.AddDays(1).AddHours(ShiftInHours);
DateTime endOfDay = startOfDay.AddDays(1);
var dailyHigh = daily.High.Last(i);
var dailyLow = daily.Low.Last(i);
var fib618n = dailyLow - (dailyHigh - dailyLow) * 0.618;
var fib382 = dailyLow + (dailyHigh - dailyLow) * 0.382;
var fib500 = dailyLow + (dailyHigh - dailyLow) * 0.5;
var fib618 = dailyLow + (dailyHigh - dailyLow) * 0.618;
var fib1618 = dailyLow + (dailyHigh - dailyLow) * 0.618;
ChartObjects.DrawLine("-61.8%" + i, startOfDay, fib618n, endOfDay, fib618n, Colors.Gray, 1, LineStyle.LinesDots);
ChartObjects.DrawLine("0.0%" + i, startOfDay, dailyHigh, endOfDay, dailyHigh, Colors.Gray, 1);
ChartObjects.DrawLine("38.2%" + i, startOfDay, fib382, endOfDay, fib382, Colors.Gray, 1, LineStyle.LinesDots);
ChartObjects.DrawLine("50.0%" + i, startOfDay, fib500, endOfDay, fib500, Colors.Gray, 1, LineStyle.Solid);
ChartObjects.DrawLine("61.8%" + i, startOfDay, fib618, endOfDay, fib618, Colors.Gray, 1, LineStyle.LinesDots);
ChartObjects.DrawLine("100.0%" + i, startOfDay, dailyLow, endOfDay, dailyLow, Colors.Gray, 1);
ChartObjects.DrawLine("161.8%" + i, startOfDay, fib1618, endOfDay, fib1618, Colors.Gray, 1, LineStyle.LinesDots);
}
}
}
}
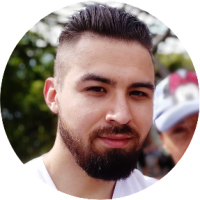
Jiri
Joined on 31.08.2015
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: DailyFiboRetracement.algo
- Rating: 4.17
- Installs: 7301
- Modified: 13/10/2021 09:55
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
No comments found.