Description
TradeManagerV03:
Control your maximum drawdown, change TP and/or SL of your all open positions at once.
Maximum DrawDown % = (Default Value) 5
If your drawdown exceeds % "x" of your balance, all open positions will be closed.
Modify Take Profit of Your Long Orders:
Enter your TP price for your Long Orders @ Long Orders TP parameter (0 removes TP)
select "yes" @ Modify Long Orders TP parameter
select "no" to stop modifying TP
Modify Stop Loss of Your Long Orders:
Enter your SL price for your Long Orders @ Long Orders SL parameter (0 removes SL)
select "yes" @ Modify Long Orders SL parameter
select "no" to stop modifying SL
Modify Take Profit of Your Short Orders:
Enter your TP price for your Short Orders @ Short Orders TP parameter (0 removes TP)
select "yes" @ Modify Short Orders TP parameter
select "no" to stop modifying TP
Modify Stop Loss of Your Short Orders:
Enter your SL price for your Short Orders @ Short Orders SL parameter (0 removes SL)
select "yes" @ Modify Short Orders SL parameter
select "no" to stop modifying SL
using System;
using System.Linq;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
namespace cAlgo
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class TradeManagerV03 : Robot
{
[Parameter("Maximum DrawDown % :", DefaultValue = 5.0, MinValue = 1.0, MaxValue = 50.0)]
public double maxDrawDown { get; set; }
[Parameter("Modify Long Orders TP :", DefaultValue = false)]
public bool modifyLongTakeProfit { get; set; }
[Parameter("Modify Long Orders SL :", DefaultValue = false)]
public bool modifyLongStopLoss { get; set; }
[Parameter("Long Orders TP :", DefaultValue = 0.0, MinValue = 0.0)]
public double longTakeProfit { get; set; }
[Parameter("Long Orders SL :", DefaultValue = 0.0, MinValue = 0.0)]
public double longStopLoss { get; set; }
[Parameter("Modify Short Orders TP :", DefaultValue = false)]
public bool modifyShortTakeProfit { get; set; }
[Parameter("Modify Short Orders SL :", DefaultValue = false)]
public bool modifyShortStopLoss { get; set; }
[Parameter("Short Orders TP :", DefaultValue = 0.0, MinValue = 0.0)]
public double shortTakeProfit { get; set; }
[Parameter("Short Orders SL :", DefaultValue = 0.0, MinValue = 0.0)]
public double shortStopLoss { get; set; }
protected override void OnStart()
{
// Put your initialization logic here
longTakeProfit = Math.Round(longTakeProfit, Symbol.Digits);
shortTakeProfit = Math.Round(shortTakeProfit, Symbol.Digits);
longStopLoss = Math.Round(longStopLoss, Symbol.Digits);
shortStopLoss = Math.Round(shortStopLoss, Symbol.Digits);
}
protected override void OnTick()
{
// Put your core logic here
if (Account.Equity < Account.Balance * ((100 - maxDrawDown) / 100))
{
foreach (var position in Positions)
ClosePositionAsync(position);
}
if (modifyLongTakeProfit == true || modifyLongStopLoss == true || modifyShortTakeProfit == true || modifyShortStopLoss == true)
{
foreach (var position in Positions)
{
double? stopLoss = position.StopLoss;
double? takeProfit = position.TakeProfit;
RefreshData();
if (position.SymbolCode != Symbol.Code)
continue;
else if (position.TradeType == TradeType.Buy)
{
if (modifyLongTakeProfit == true && longTakeProfit == 0)
takeProfit = null;
else if (modifyLongTakeProfit == true && longTakeProfit > 0 && longTakeProfit > Symbol.Bid)
takeProfit = longTakeProfit;
if (modifyLongStopLoss == true && longStopLoss == 0)
stopLoss = null;
else if (modifyLongStopLoss == true && longStopLoss > 0 && longStopLoss < Symbol.Bid)
stopLoss = longStopLoss;
}
else if (position.TradeType == TradeType.Sell)
{
if (modifyShortTakeProfit == true && shortTakeProfit == 0)
takeProfit = null;
else if (modifyShortTakeProfit == true && shortTakeProfit > 0 && shortTakeProfit < Symbol.Ask)
takeProfit = shortTakeProfit;
if (modifyShortStopLoss == true && shortStopLoss == 0)
stopLoss = null;
else if (modifyShortStopLoss == true && shortStopLoss > 0 && shortStopLoss > Symbol.Ask)
stopLoss = shortStopLoss;
}
if (stopLoss != position.StopLoss || takeProfit != position.TakeProfit)
ModifyPositionAsync(position, stopLoss, takeProfit);
}
}
}
protected override void OnStop()
{
// Put your deinitialization logic here
}
}
}
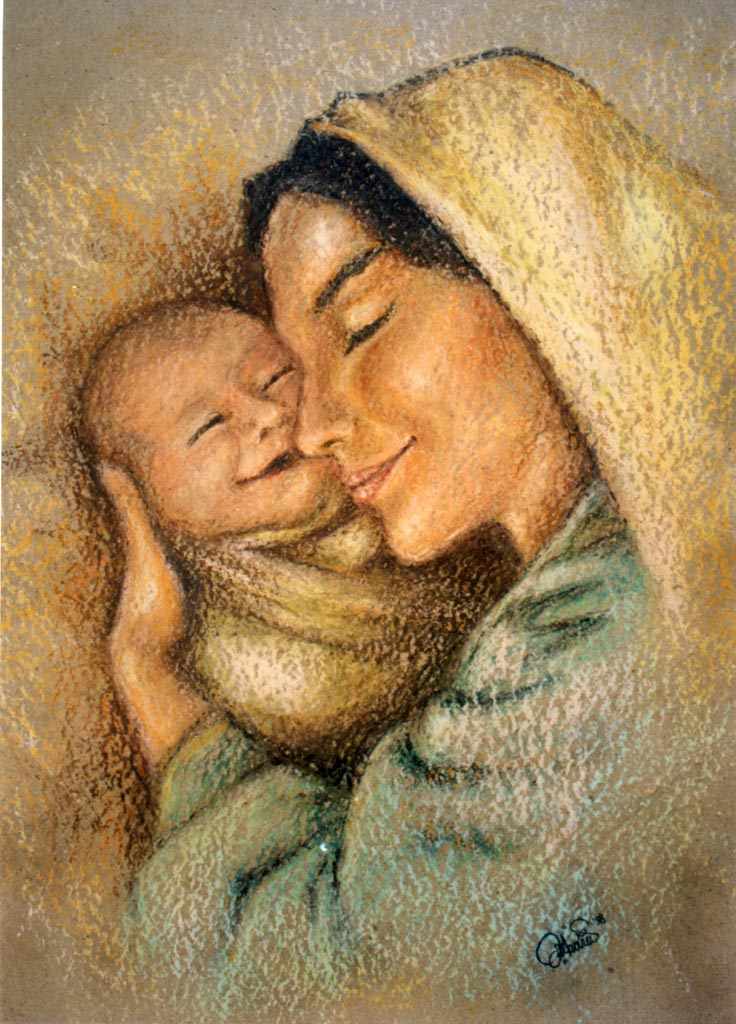
ozan
Joined on 07.11.2015
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: TradeManagerV03.algo
- Rating: 5
- Installs: 3346
- Modified: 13/10/2021 09:54
Comments
It's on top of that a superb upload that him and i definitely treasured analyzing. It's actually not each day we have opportunity to know a specific thing. term loans
That is furthermore a great submit that people genuinely cherished examining. It isn't every day that we hold the probability to find out one thing.Buy cocaine in Hamilton
My partner and i favour your overall write-up. It could be great to look at any person describe inside terms from the aerobic in addition to lucidity because of this essential problem could be swiftly noticed.movierulz com movies download app
Are you looking to sell merchandise? Overstock Goods Outlet is the perfect place to do it. We are always looking for new merchandise to purchase and sell to our customers. If you have overstock, shelf pulls, surplus, or returns from major retailers or catalog companies, we would love to hear from you. Contact us today to learn more about how you can sell your merchandise to us.
Vielen dank für den Artikel. daraus kann man sich einen guten Eindruck verschaffen Grüsse Meike Snorkeling adventures in the Caribbean Sea
That's the reason it is best you must corresponding investigation in advance of designing. You're able to present better send in using this method.roller shades
Thank you, I have recently been looking for information approximately this topic for a long time and yours is the greatest I’ve found out till now. However, what about the conclusion? Are you positive about the source?Visit Here
Wanderlust Waves Travel is a comprehensive travel website that offers everything you need to plan your next vacation, tour, Trip, or holiday. Whether you're a solo traveler, a family, or a group of friends, this website provides a wide range of options to help you find the perfect travel plans that suit your budget and preferences. With an easy-to-use interface, users can easily search for destinations, book flights, accommodations, and travel packages. You can also book local experiences and get expert advice from the travel team. The website offers 24/7 customer support, ensuring that you have peace of mind when booking your next travel adventure. Book your next trip with Wanderlust Waves Travel for a hassle-free and memorable travel experience.
Explore more about Tops We offer a curated collection of clothing inspired by a range of alternative styles.
Hi, i believe that i saw you visited my web site thus i got here to “go back the want”.I’m trying to in finding things to enhance my web site!I guess its good enough to use some of your ideas!!Tour Sigulda
Usually there are some dissertation sites making use of the website whenever you develop into in plain english reported in the site.物理課程
Not long ago, We didn’t provide a bunch of shown to allowing reactions on website page content pieces and still have placed suggestions also a lesser amount of. Looking at by your pleasurable posting, helps me personally to take action often.egr delete lbz