Description
custom MovingAverageV02
Moving Average with shift for different price types;
(0) = Typical Price
(1) = Weighted Close Price
(2) = Median Price
Typical Price = (O+H+L+C) /4
Weighted Close Price = (O+H+L+C+C) /5
Median Price = (H+L) /2
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
using System;
namespace cAlgo.Indicators
{
[Indicator(IsOverlay = true, AccessRights = AccessRights.None)]
public class customMovingAverageV02 : Indicator
{
[Parameter("Price Type: (0)=Typical (OHLC) , (1)=WClose (OHLCC) , (2)=Median (HL)", DefaultValue = 0, MinValue = 0, MaxValue = 2)]
public int PriceType { get; set; }
[Parameter("MA Type:", DefaultValue = MovingAverageType.Simple)]
public MovingAverageType MAType { get; set; }
[Parameter("Period:", DefaultValue = 8)]
public int Period { get; set; }
[Parameter("Shift:", DefaultValue = 0, MinValue = -100, MaxValue = 500)]
public int Shift { get; set; }
[Output("Main", Color = Colors.Blue)]
public IndicatorDataSeries MAResult { get; set; }
private MovingAverage movingAverage;
private IndicatorDataSeries dataSeries;
protected override void Initialize()
{
dataSeries = CreateDataSeries();
movingAverage = Indicators.MovingAverage(dataSeries, Period, MAType);
}
public override void Calculate(int index)
{
if (Shift < 0 && index < Math.Abs(Shift))
return;
if (PriceType == 0)
{
dataSeries[index] = (MarketSeries.Open[index] + MarketSeries.High[index] + MarketSeries.Low[index] + MarketSeries.Close[index]) / 4;
}
else if (PriceType == 1)
{
dataSeries[index] = (MarketSeries.Open[index] + MarketSeries.High[index] + MarketSeries.Low[index] + MarketSeries.Close[index] + MarketSeries.Close[index]) / 5;
}
else if (PriceType == 2)
{
dataSeries[index] = (MarketSeries.High[index] + MarketSeries.Low[index]) / 2;
}
MAResult[index + Shift] = movingAverage.Result[index];
}
}
}
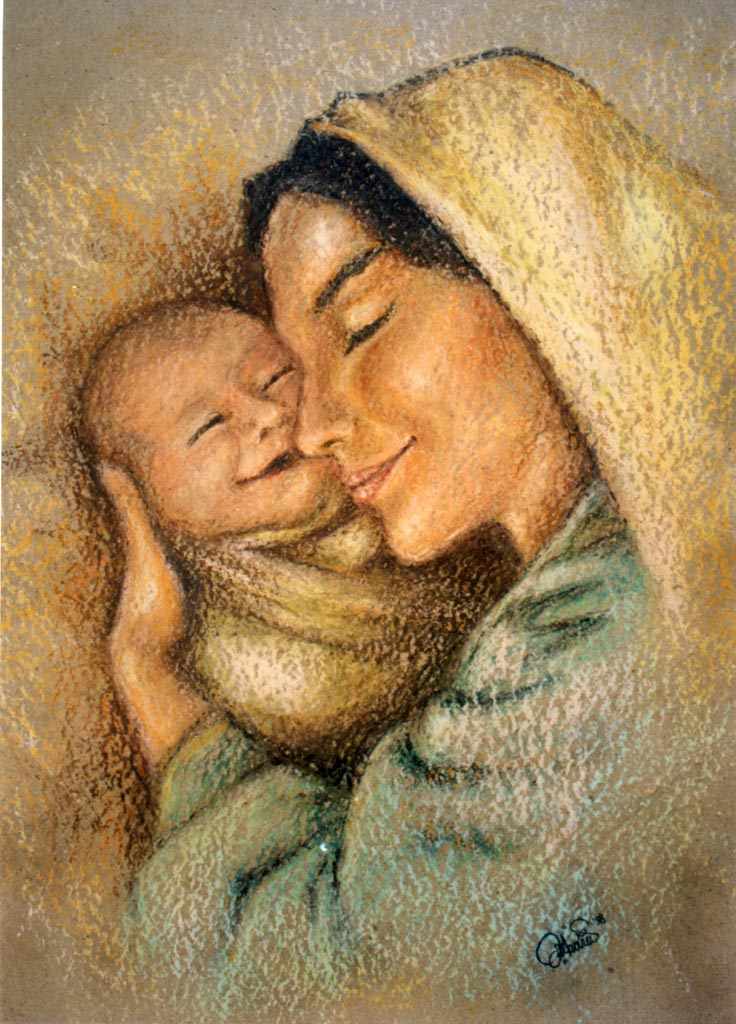
ozan
Joined on 07.11.2015
- Distribution: Free
- Language: C#
- Trading platform: cTrader Automate
- File name: customMovingAverage.algo
- Rating: 5
- Installs: 5453
- Modified: 13/10/2021 09:54
Note that publishing copyrighted material is strictly prohibited. If you believe there is copyrighted material in this section, please use the Copyright Infringement Notification form to submit a claim.
Comments
Log in to add a comment.
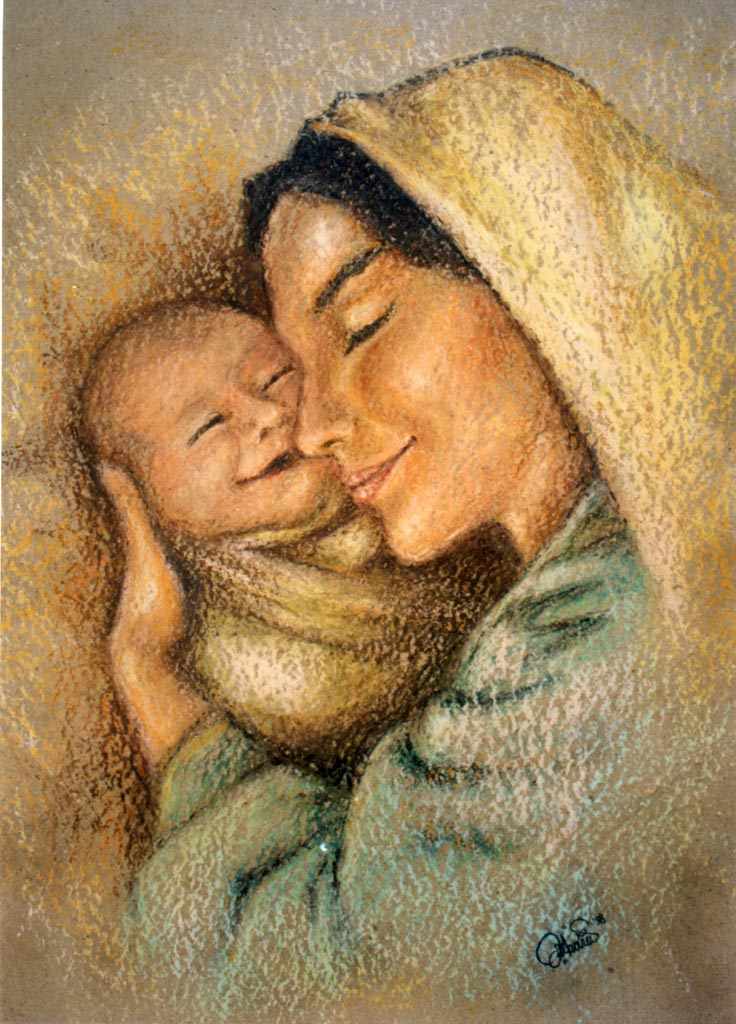
For a better Source Price Type Selection with dropdown list , please vote for enum parameters support in cAlgo.
Hi Ozan,
Regarding your TradeManagerV03 ( /algos/cbots/show/1080 ) :
Is it possible to use that cBot and set the 'Maximum DrawDown %' parameter – so that my open trades get closed, once I reach a specific Equity profit target ?
For example: When I make 8% in equity growth, then my open trades have their Stop Losses moved up close to lock in the profit.
Thanks.